
Test program for PwmSound class. Provides tests (demos) for the various sound generating functions in the class. Includes several MML melodies and music clips to test the play() function. Tested on an mbed LPC1768.
main.cpp
00001 /****************************************************************************** 00002 * File: main.cpp 00003 * Author: Paul Griffith 00004 * Created: 25 Mar 2014 00005 * Last Edit: see below 00006 * Version: see below 00007 * 00008 * Description: 00009 * Test program for PwmSound class. 00010 * 00011 * Copyright (c) 2014 Paul Griffith, MIT License 00012 * 00013 * Permission is hereby granted, free of charge, to any person obtaining a copy 00014 * of this software and associated documentation files (the "Software"), to 00015 * deal in the Software without restriction, including without limitation the 00016 * rights to use, copy, modify, merge, publish, distribute, sublicense, and/or 00017 * sell copies of the Software, and to permit persons to whom the Software is 00018 * furnished to do so, subject to the following conditions: 00019 * 00020 * The above copyright notice and this permission notice shall be included in 00021 * all copies or substantial portions of the Software. 00022 * 00023 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00024 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00025 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00026 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00027 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00028 * FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS 00029 * IN THE SOFTWARE. 00030 * 00031 * Modifications: 00032 * Ver Date By Details 00033 * 0.00 25Mar14 PG File created. 00034 * 1.00 30Mar14 PG Initial release. 00035 * 2.00 06May14 PG Many changes and additions to test V2.00 library. 00036 * 00037 ******************************************************************************/ 00038 00039 #include "mbed.h" 00040 #include "PwmSound.h" 00041 00042 PwmSound mySpeaker(p24); 00043 Serial pc(USBTX, USBRX); 00044 00045 void printHelps(void); 00046 void badArgs(void); 00047 void nyi(void); 00048 void pause(void); 00049 00050 // Tone fragments 00051 00052 #define DOODLY "O3>CG8G8AGPB>C" 00053 #define YAY "O4L32MLCDEFG" //success 00054 #define WAH "T80O2L32GFEDC" //failure 00055 00056 // Preset melodies in MML format 00057 // Melodies 0-3 are taken from Michael J. Mefford's MS-DOS PLAY utility which 00058 // was published as part of a PC Magazine cover disk in 1992. 00059 00060 // Super Mario Brothers theme (11 notes) 00061 00062 char* smb = { 00063 "T180 O3 E8 E8 P8 E8 P8 C8 D#4 G4 P4 <G4 P4" 00064 }; 00065 00066 char* preset0 = { //short Dragnet theme 00067 "T90 O2 L8E. L16F# L8G E P2 L8E. L16F# L8G E L3 B-" 00068 }; 00069 00070 char* preset1 = { //long Dragnet theme 00071 "T190O2L4EL8EL4MLG.MNL4G.EL8GL4AL8AL4MLG.MNL2G.L4EL8EL4MLG.MNL4G.EL8GL4AL8AL4MLG." 00072 "MNL2G.T90L8E.L16F#L8GEP2L8E.L16F#L8GEL3B-" 00073 }; 00074 00075 char* preset2 = { //Greensleeves 00076 "O3A O4L2CL4D E.L8FL4E L2DL4O3B G.L8AL4B O4L2CO3L4A A.L8G#L4A L2BL4G# O3L2EL4A" 00077 "O4L2CL4D E.L8FL4E L2DL4O3B G.L8AL4B O4L4C.O3L8BL4A L4G#.L8F#L4G# L2A. L2A." 00078 "O4L2G. L4G.L8F#L4E L2DO3L4B G.L8AL4B L2O4CO3L4A A.L8G#L4A L2BL4G# L2E." 00079 "O4L2G. L4G.L8F#L4E L2DO3L4B G.L8AL4B O4L4C.O3L8BL4A G#.L8F#L4G# L2A. A." 00080 }; 00081 00082 char* preset3 = { //The Entertainer by Scott Joplin 00083 ":The Entertainer by Scott Joplin\n" //comment lines need a \n 00084 "" 00085 "T80 :Prelude\n" 00086 "" 00087 "O4MLL64C#MNL16DECO3L8AL16BMSL8G" 00088 "MLL64C#MNL16DECO2L8AL16BL8MSGMN" 00089 "O2MLL64C#MNL16DECO1L8AL16BAA-" 00090 "MSL8GMNP4O3L8GO2L16MLDMND#" 00091 "" 00092 "MLO2L16EMSL8O3CMLO2L16EO3MSL8C" 00093 "MNO2L16EO3L4C.L16O4CDD#" 00094 "ECDL8EL16O3BO4MSL8D" 00095 "MNCL16O3MLEGO4MSL8CMLO2L16DMND#" 00096 "MLO2L16EMSL8O3CMLO2L16EO3MSL8C" 00097 "MLO2L16EO3L4C.L16MLAMNG" 00098 "F#AO4CL8EL16DCO3A" 00099 "O4L4D.O1L16MLDMND#" 00100 "MLO2L16EMSL8O3CMLO2L16EO3MSL8C" 00101 "MNO2L16EO3L4C.L16O4CDD#" 00102 "ECDL8EL16O3BO4MSL8D" 00103 "MNCL16O3MLEGO4MSL8CMNL16CD" 00104 "ECDL8EL16CDC" 00105 "ECDL8EL16CDC" 00106 "ECDL8EL16O3BO4L8MSDMN" 00107 "L5MLC.MNL16O3EFF#" 00108 "" 00109 "O3L8MSGMNL16AL8GL16EFF#" 00110 "MSL8GMNL16AL8GL16MSECO2G" 00111 "MLL16ABO3CDEDCD" 00112 "MNO2GMLO3EFGAGEMNF" 00113 "L8MSGMNL16AL8GL16EFF#" 00114 "L8MSGMNL16AL8GL16GAA#" 00115 "BL8BL16L8BL16AF#D" 00116 "L5MLG.MNL16EFF#" 00117 "MSL8GMNL16AL8GL16EFF#" 00118 "L8MSGMNL16AL8GL16MLECO3MNG" 00119 "MLABO4CDEDCD" 00120 "MSL8MLCL16EGMSO5CO4MNGF#G" 00121 "MSO5L8CO4MNL16AO5L8CL16O4AO5CO4A" 00122 "GO5CEL8GL16ECO4G" 00123 "MSL8AO5CMNL16EL8D" 00124 "L4C..L16O4EFF#" 00125 "MSL8GMNL16AL8GL16EFF#" 00126 "L8GL16AL8GL16MSECO3G" 00127 "MNABO4CDEDCD" 00128 "O3GO4EFGAGEF" 00129 "MSL8GMNL16AL8GL16EFF#" 00130 "L8GL16AL8GL16GAA#" 00131 "" 00132 "BL8BBL16AF#D" 00133 "MLL64GAGAGAGAGAGAGAGAGL16MNEFF#" 00134 "L8MSGL16AL8GL16EFF#" 00135 "L8GL16AL8GL16MSECO3G" 00136 "MNABO4CDEDCD" 00137 "MSL8CL16MLEGO5MNCO4GF#G" 00138 "O5MSL8CO4MNL16AMLO5L8CL16O4AO5" 00139 "CO4AGO5CEL8GL16ECO4G" 00140 "L8MSAO5CMNL16EL8DL16MLC" 00141 "L4CL8C" 00142 }; 00143 00144 // The M.GAKKOU KOUKA 00145 // copyright "Music Composed by Kenkichi Motoi 2009 Wikimedia version 2012" 00146 00147 char* preset4 = { //play with sticky shift? 00148 "T160 O3L4" 00149 "ED8CE8 GG8ER8 AA8>C<A8 G2R" 00150 "AA8GA8 >CC8D<R8 EE8DE8 C2R" 00151 "DD8DD8 DD8DR8 ED8EF8 G2R" 00152 "AA8GA8 >CC8<AR8 >DC8DE8 D2<R" 00153 ">EE8DC8< AB8>CC8< GG8EA8 G2R" 00154 ">CC8<GE8 CD8EA8 GG8DE8 C2R" 00155 }; 00156 00157 int main() 00158 { 00159 char buf[40]; 00160 int i, n; 00161 Timer t; 00162 00163 pc.printf("\nPWM Sound Demo 8, %s %s\n", __DATE__, __TIME__); 00164 mySpeaker.play(DOODLY); 00165 00166 while(1) { 00167 pc.printf("Enter command (? for help): "); 00168 n = pc.getc(); 00169 pc.printf("%c", n); 00170 if (n == '?') { 00171 pc.printf("\n"); 00172 printHelps(); 00173 } else if (n =='0') { 00174 mySpeaker.siren(0); 00175 } else if (n == '1') { 00176 i = pc.getc() - '0'; 00177 pc.printf("%d", i); 00178 mySpeaker.play(YAY); 00179 } else if (n == '2') { 00180 i = pc.getc() - '0'; 00181 pc.printf("%d", i); 00182 mySpeaker.play(WAH); 00183 } else if (n == '3') { 00184 i = pc.getc() - '0'; 00185 pc.printf("%d", i); 00186 mySpeaker.trill(i); 00187 } else if (n == '4') { 00188 i = pc.getc() - '0'; 00189 pc.printf("%d", i); 00190 mySpeaker.phone(i); 00191 } else if (n == '5') { 00192 i = pc.getc() - '0'; 00193 pc.printf("%d", i); 00194 mySpeaker.bip(i); 00195 wait(1); 00196 mySpeaker.bop(i); 00197 } else if (n == '6') { 00198 i = pc.getc() - '0'; 00199 pc.printf("%d", i); 00200 mySpeaker.bop(i); 00201 wait(1); 00202 mySpeaker.bop(i); 00203 } else if (n == '7') { 00204 i = pc.getc() - '0'; 00205 pc.printf("%d", i); 00206 mySpeaker.beep(i); 00207 } else if (n == '8') { 00208 i = pc.getc() - '0'; 00209 pc.printf("%d", i); 00210 mySpeaker.bleep(i); 00211 } else if (n =='9') { 00212 i = pc.getc() - '0'; 00213 pc.printf("%d", i); 00214 mySpeaker.buzz(i); 00215 } else if (n == '=') { //press = to stop a continuous sound 00216 mySpeaker.stop(); 00217 } else if (n == 'm') { //press m for SMB theme 00218 mySpeaker.play(smb); 00219 } else if (n == 'p') { //press pn to play a tune in MML format 00220 i = pc.getc() - '0'; 00221 pc.printf("%d", i); 00222 if (i == 0) { 00223 n = mySpeaker.play(preset0); 00224 } else if (i == 1) { 00225 n = mySpeaker.play(preset1); 00226 } else if (i == 2) { 00227 n = mySpeaker.play(preset2); 00228 } else if (i == 3) { 00229 n = mySpeaker.play(preset3); 00230 } else if (i == 4) { 00231 n = mySpeaker.play(preset4, 0); 00232 } else if (i == 5) { 00233 n = mySpeaker.play(preset4, 1); 00234 } else if (i == 6) { 00235 n = mySpeaker.play(preset4, 2); 00236 } else if (i == 7) { 00237 n = mySpeaker.play(preset4, 3); 00238 } 00239 if (n > 0) { 00240 pc.printf(" ??input error at char %d", n); 00241 } 00242 } else if (n == 'q') { //play quick tune 00243 scanf("%39s", buf); 00244 pc.printf("%s", buf); 00245 n = mySpeaker.play(buf); 00246 if (n > 0) { 00247 pc.printf(" ??input error at char %d", n); 00248 } 00249 } else if (n == 't') { //press tn to set timbre 00250 i = pc.getc() - '0'; 00251 pc.printf("%d", i); 00252 mySpeaker.timbre(i); 00253 } else if (n == 'z') { //press z to time tone() call 00254 t.reset(); 00255 t.start(); 00256 for (int i = 0; i < 1000000; i++) { 00257 mySpeaker.tone(440.0, 0.0); 00258 } 00259 t.stop(); 00260 pc.printf("<1 millon calls took %f seconds>", t.read()); 00261 } else { 00262 badArgs(); 00263 } 00264 pc.printf("\n"); 00265 } //end of while 00266 } 00267 00268 void printHelps(void) { 00269 pc.printf("0 Two-tone (police siren) in background (press = to stop)\n"); 00270 pc.printf("1x Yay (success) x times\n"); 00271 pc.printf("2x Wah (failure) x times\n"); 00272 pc.printf("3x Trill x times (0 = continuous in background)\n"); 00273 pc.printf("4x Phone x times\n"); 00274 pc.printf("5 Bip x times (0 = continuous in background)\n"); 00275 pc.printf("6x Bop x times (0 = continuous in background)\n"); 00276 pc.printf("7x Beep x times (0 = continuous in background)\n"); 00277 pc.printf("8x Bleep x times (0 = continuous in background)\n"); 00278 pc.printf("9x Buzz x times (0 = continuous in background)\n"); 00279 pc.printf("= Stop background sound or music\n"); 00280 pc.printf("m Super Mario Brothers theme\n"); 00281 pc.printf("px Play preset tune x in MML format (x = 0-7)\n"); 00282 pc.printf("q abc Play quick tune as entered on the command line\n"); 00283 pc.printf("tx Set timbre (x = 1-4)\n"); 00284 } 00285 00286 //************************ 00287 // Miscellaneous functions 00288 //************************ 00289 00290 void badArgs(void) { 00291 pc.printf("?? Bad arguments\n"); 00292 } 00293 00294 void nyi(void) { 00295 pc.printf("!! Not yet implemented\n"); 00296 } 00297 00298 void pause(void) 00299 { 00300 pc.printf("Press any key to continue . . .\n"); 00301 pc.getc(); 00302 } 00303 00304 //END of main.cpp
Generated on Thu Jul 14 2022 20:19:19 by
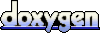