Build Realtime Apps With The Real-Time Network - the mbed PubNub API+SDK
Embed:
(wiki syntax)
Show/hide line numbers
PubNub.h
Go to the documentation of this file.
00001 /* PubNub.h */ 00002 /* Copyright (c) 2013 PubNub Inc. 00003 * http://www.pubnub.com/ 00004 * http://www.pubnub.com/terms 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 /** \file 00026 PubNub Client header file 00027 */ 00028 00029 #ifndef PubNub_h 00030 #define PubNub_h 00031 00032 #include "TCPSocketConnection.h" 00033 00034 00035 /* TODO: UUID */ 00036 /* TODO: secret_key */ 00037 /* TODO: cipher_key */ 00038 /* TODO: timeout support */ 00039 00040 /* Maximum length of the HTTP reply. This buffer is dynamically allocated 00041 * only when loading the reply from the network. */ 00042 /* XXX: Replies of API calls longer than this will be discarded and instead, 00043 * PNR_FORMAT_ERROR will be reported. Specifically, this may cause lost 00044 * messages returned by subscribe if too many too large messages got queued. */ 00045 #ifndef PUBNUB_REPLY_MAXLEN 00046 #define PUBNUB_REPLY_MAXLEN (2048-8-1) 00047 #endif 00048 00049 00050 /* Result codes for PubNub methods. */ 00051 enum PubNubRes { 00052 /* Success. */ 00053 PNR_OK, 00054 /* Time out before the request has completed. */ 00055 PNR_TIMEOUT, 00056 /* Communication error (network or HTTP response format). */ 00057 PNR_IO_ERROR, 00058 /* HTTP error. */ 00059 PNR_HTTP_ERROR, 00060 /* Unexpected input in received JSON. */ 00061 PNR_FORMAT_ERROR, 00062 /* PubNub JSON reply indicates failure. */ 00063 PNR_PUBNUB_ERROR, 00064 }; 00065 00066 00067 /** A PubNub Client context. Declare it as a local or global variable, 00068 * then you may start calling the API immediately. 00069 * 00070 * All methods are blocking; if you need to communicate with 00071 * PubNub asynchronously, run PubNub in a dedicated thread. 00072 * Always use the PubNub context only in a single thread at once. 00073 * 00074 * Message are passed as strings instead of JSON structures due 00075 * to much higher RAM efficiency. Often, ad hoc composing and 00076 * parsing JSON messages will work fine in practice. Otherwise, 00077 * take a look at e.g. the picojson library. */ 00078 class PubNub { 00079 public: 00080 /** Init a Pubnub Client context 00081 * 00082 * Note that the string parameters are not copied; do not 00083 * overwrite or free the memory where you stored the keys! 00084 * (If you are passing string literals, don't worry about it.) 00085 * 00086 * @param string publish_key required key to send messages. 00087 * @param string subscribe_key required key to receive messages. 00088 * @param string origin optional setting for cloud origin. */ 00089 PubNub(const char *publish_key, const char *subscribe_key, const char *origin = "http://pubsub.pubnub.com"); 00090 00091 ~PubNub(); 00092 00093 /** Publish API call 00094 * 00095 * Send a message (assumed to be well-formed JSON) to a given channel. 00096 * 00097 * Examples: 00098 * @code 00099 p.publish("demo", "\"lala\""); 00100 * @endcode 00101 * or 00102 * @code 00103 if (p.publish("demo", "{\"lala\":1}") != PNR_OK) { 00104 blink_error(); 00105 } 00106 * @endcode 00107 * 00108 * @param channel required channel name. 00109 * @param message required JSON message object. 00110 * @param reply optional pointer for passing the returned reply (free() after use). 00111 * @return PNR_OK on success. */ 00112 PubNubRes publish(const char *channel, const char *message, char **reply = NULL); 00113 00114 /** Subscribe API call 00115 * 00116 * Listen for a message on a given channel. The function will block 00117 * and return when a message arrives. Typically, you will run this 00118 * function in a loop to keep listening for messages indefinitely. 00119 * 00120 * As a reply, you will get a JSON message. If you are used to 00121 * PubNub API in other environments, you would expect an array 00122 * of messages; here, even if the device received multiple messages 00123 * batched, they are spread over subsequent PubNub.subscribe() calls 00124 * and each returns only a single message. However, *reply can be 00125 * set NULL - in that case, simply retry the call, this indicates 00126 * an empty reply from the server (what happens e.g. after waiting 00127 * for certain interval, or immediately after subscribing). 00128 * 00129 * Contrary to publish(), you should NOT free() the reply yourself! 00130 * Instead, you are expected to call another subscribe() just after 00131 * processing the reply; subscribe() will release any memory it can 00132 * before waiting for new data from the server. 00133 * 00134 * Examples: 00135 * @code 00136 while (1) { 00137 char *reply; 00138 if (p.subscribe("demo", &reply) != PNR_OK) continue; 00139 if (!reply) continue; 00140 int code = -1; 00141 if (sscanf(reply, "{\"code\":%d}", &code) == 1) 00142 printf("received JSON msg with code %d\n", code); 00143 } 00144 * @endcode 00145 * 00146 * @param channel required channel name. 00147 * @param reply required pointer for passing the returned reply (do not free()). 00148 * @return PNR_OK on success. */ 00149 PubNubRes subscribe(const char *channel, char **reply); 00150 00151 /* TODO: subscribe_multi */ 00152 00153 /** History API call 00154 * 00155 * Receive list of the last N messages on the given channel. 00156 * 00157 * The messages are returned in the reply buffer, with each message 00158 * terminated by a NUL byte, i.e. being a standalone C string; to 00159 * iterate over all the messages, you can use the replysize. reply 00160 * can be NULL if there is no history for this channel. 00161 * 00162 * Example: 00163 * @code 00164 char *reply; 00165 int replysize; 00166 if (p.history("demo", &reply, &replysize) != PNR_OK) return; 00167 if (!reply) return; 00168 for (char *msg = reply; msg < reply + replysize; msg += strlen(msg)+1) 00169 printf("historic message: %s\n", msg); 00170 free(reply); 00171 * @endcode 00172 * 00173 * @param channel required channel name. 00174 * @param reply required pointer for passing the returned reply (free() after use). 00175 * @param replysize required pointer for returning the total reply size. 00176 * @param int limit optional number of messages to retrieve. 00177 * @return PNR_OK on success. */ 00178 PubNubRes history(const char *channel, char **reply, int *replysize, int limit = 10); 00179 00180 /** Time API call 00181 * 00182 * Receive the current server timestamp and store it in the 00183 * provided string buffer (a char[32] or larger). 00184 * 00185 * @param ts required pointer to the string buffer. 00186 * @return PNR_OK on success. */ 00187 PubNubRes time(char *ts); 00188 00189 /* TODO: here_now */ 00190 00191 protected: 00192 const char *m_publish_key, *m_subscribe_key; 00193 00194 const char *m_origin; 00195 const char *origin_hostname(); 00196 00197 char m_timetoken[32]; 00198 char *m_replybuf; 00199 int m_replylen; 00200 00201 /* Back-end post-processing of subscribe(). free()s *reply 00202 * in the process. */ 00203 PubNubRes subscribe_processjson(char *reply, int replylen); 00204 }; 00205 00206 #endif
Generated on Tue Jul 12 2022 13:56:24 by
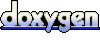