Initial release
Fork of nrf51-sdk by
Embed:
(wiki syntax)
Show/hide line numbers
nrf_mbr.h
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 /** 00033 @defgroup nrf_mbr_api Master Boot Record API 00034 @{ 00035 00036 @brief APIs for updating SoftDevice and BootLoader 00037 00038 */ 00039 00040 /* Header guard */ 00041 #ifndef NRF_MBR_H__ 00042 #define NRF_MBR_H__ 00043 00044 #include "nrf_svc.h" 00045 #include <stdint.h> 00046 00047 00048 /** @addtogroup NRF_MBR_DEFINES Defines 00049 * @{ */ 00050 00051 /**@brief MBR SVC Base number. */ 00052 #define MBR_SVC_BASE (0x18) 00053 00054 /**@brief Page size in words. */ 00055 #define PAGE_SIZE_IN_WORDS 256 00056 /** @} */ 00057 00058 /** @addtogroup NRF_MBR_ENUMS Enumerations 00059 * @{ */ 00060 00061 /**@brief nRF Master Boot Record API SVC numbers. */ 00062 enum NRF_MBR_SVCS 00063 { 00064 SD_MBR_COMMAND = MBR_SVC_BASE, /**< ::sd_mbr_command */ 00065 }; 00066 00067 /**@brief Possible values for ::sd_mbr_command_t.command */ 00068 enum NRF_MBR_COMMANDS 00069 { 00070 SD_MBR_COMMAND_COPY_BL, /**< Copy a new BootLoader. @see sd_mbr_command_copy_bl_t */ 00071 SD_MBR_COMMAND_COPY_SD, /**< Copy a new SoftDevice. @see ::sd_mbr_command_copy_sd_t*/ 00072 SD_MBR_COMMAND_INIT_SD, /**< Init forwarding interrupts to SD, and run reset function in SD*/ 00073 SD_MBR_COMMAND_COMPARE, /**< This command works like memcmp. @see ::sd_mbr_command_compare_t*/ 00074 SD_MBR_COMMAND_VECTOR_TABLE_BASE_SET, /**< Start forwarding all exception to this address @see ::sd_mbr_command_vector_table_base_set_t*/ 00075 }; 00076 00077 /** @} */ 00078 00079 /** @addtogroup NRF_MBR_TYPES Types 00080 * @{ */ 00081 00082 /**@brief This command copies part of a new SoftDevice 00083 * The destination area is erased before copying. 00084 * If dst is in the middle of a flash page, that whole flash page will be erased. 00085 * If (dst+len) is in the middle of a flash page, that whole flash page will be erased. 00086 * 00087 * The user of this function is responsible for setting the PROTENSET registers. 00088 * 00089 * @retval ::NRF_SUCCESS indicates that the contents of the memory blocks where copied correctly. 00090 * @retval ::NRF_ERROR_INTERNAL indicates that the contents of the memory blocks where not verified correctly after copying. 00091 */ 00092 typedef struct 00093 { 00094 uint32_t *src; /**< Pointer to the source of data to be copied.*/ 00095 uint32_t *dst; /**< Pointer to the destination where the content is to be copied.*/ 00096 uint32_t len; /**< Number of 32 bit words to copy. Must be a multiple of @ref PAGE_SIZE_IN_WORDS words.*/ 00097 } sd_mbr_command_copy_sd_t; 00098 00099 00100 /**@brief This command works like memcmp, but takes the length in words. 00101 * 00102 * @retval ::NRF_SUCCESS indicates that the contents of both memory blocks are equal. 00103 * @retval ::NRF_ERROR_NULL indicates that the contents of the memory blocks are not equal. 00104 */ 00105 typedef struct 00106 { 00107 uint32_t *ptr1; /**< Pointer to block of memory. */ 00108 uint32_t *ptr2; /**< Pointer to block of memory. */ 00109 uint32_t len; /**< Number of 32 bit words to compare.*/ 00110 } sd_mbr_command_compare_t; 00111 00112 00113 /**@brief This command copies a new BootLoader. 00114 * With this command, destination of BootLoader is always the address written in NRF_UICR->BOOTADDR. 00115 * 00116 * Destination is erased by this function. 00117 * If (destination+bl_len) is in the middle of a flash page, that whole flash page will be erased. 00118 * 00119 * This function will use PROTENSET to protect the flash that is not intended to be written. 00120 * 00121 * On Success, this function will not return. It will start the new BootLoader from reset-vector as normal. 00122 * 00123 * @retval ::NRF_ERROR_INTERNAL indicates an internal error that should not happen. 00124 * @retval ::NRF_ERROR_FORBIDDEN if NRF_UICR->BOOTADDR is not set. 00125 * @retval ::NRF_ERROR_INVALID_LENGTH if parameters attempts to read or write outside flash area. 00126 */ 00127 typedef struct 00128 { 00129 uint32_t *bl_src; /**< Pointer to the source of the Bootloader to be be copied.*/ 00130 uint32_t bl_len; /**< Number of 32 bit words to copy for BootLoader. */ 00131 } sd_mbr_command_copy_bl_t; 00132 00133 /**@brief Sets the base address of the interrupt vector table for interrupts forwarded from the MBR 00134 * 00135 * Once this function has been called, this address is where the MBR will start to forward interrupts to after a reset. 00136 * 00137 * To restore default forwarding this function should be called with @param address set to 0. 00138 * The MBR will then start forwarding to interrupts to the address in NFR_UICR->BOOTADDR or to the SoftDevice if the BOOTADDR is not set. 00139 * 00140 * @retval ::NRF_ERROR_INTERNAL indicates an internal error that should not happen. 00141 * @retval ::NRF_ERROR_INVALID_ADDR if parameter address is outside of the flash size. 00142 */ 00143 typedef struct 00144 { 00145 uint32_t address; /**< The base address of the interrupt vector table for forwarded interrupts.*/ 00146 } sd_mbr_command_vector_table_base_set_t; 00147 00148 typedef struct 00149 { 00150 uint32_t command; /**< type of command to be issued see @ref NRF_MBR_COMMANDS. */ 00151 union 00152 { 00153 sd_mbr_command_copy_sd_t copy_sd; /**< Parameters for copy SoftDevice.*/ 00154 sd_mbr_command_copy_bl_t copy_bl; /**< Parameters for copy BootLoader.*/ 00155 sd_mbr_command_compare_t compare; /**< Parameters for verify.*/ 00156 sd_mbr_command_vector_table_base_set_t base_set; /**< Parameters for vector table base set.*/ 00157 } params; 00158 } sd_mbr_command_t; 00159 00160 /** @} */ 00161 00162 /** @addtogroup NRF_MBR_FUNCTIONS Functions 00163 * @{ */ 00164 00165 /**@brief Issue Master Boot Record commands 00166 * 00167 * Commands used when updating a SoftDevice and bootloader. 00168 * 00169 * @param[in] param Pointer to a struct describing the command. 00170 * 00171 *@note for retvals see ::sd_mbr_command_copy_sd_t ::sd_mbr_command_copy_bl_t ::sd_mbr_command_compare_t 00172 00173 */ 00174 SVCALL(SD_MBR_COMMAND, uint32_t, sd_mbr_command(sd_mbr_command_t* param)); 00175 00176 /** @} */ 00177 #endif // NRF_MBR_H__ 00178 00179 /** 00180 @} 00181 */
Generated on Tue Jul 12 2022 11:17:18 by
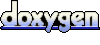