GPS for mbed
Dependents: ecu_reader gsm_SET
GPS.h
00001 /* GPS class for mbed Microcontroller 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "mbed.h" 00006 00007 #ifndef GPS_H 00008 #define GPS_H 00009 00010 /* Class: GPS 00011 * A GPS interface for reading from a Globalsat EM-406 GPS Module 00012 */ 00013 class GPS { 00014 00015 public: 00016 00017 /* Constructor: GPS 00018 * Create the GPS, connected to the specified serial port 00019 */ 00020 GPS(PinName tx, PinName rx); 00021 00022 /* Function: sample 00023 * Sample the incoming GPS data, returning whether there is a lock 00024 * 00025 * Variables: 00026 * returns - 1 if there was a lock when the sample was taken (and therefore .longitude and .latitude are valid), else 0 00027 */ 00028 int sample(); 00029 00030 /* Variable: longitude 00031 * The longitude (call sample() to set) 00032 */ 00033 float longitude; 00034 00035 /* Variable: latitude 00036 * The latitude (call sample() to set) 00037 */ 00038 float latitude; 00039 00040 private: 00041 00042 float trunc(float v); 00043 void getline(); 00044 00045 Serial _gps; 00046 char msg[256]; 00047 00048 }; 00049 00050 #endif
Generated on Mon Jul 18 2022 06:08:36 by
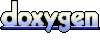