GPS for mbed
Dependents: ecu_reader gsm_SET
GPS.cpp
00001 /* GPS class for mbed Microcontroller 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "GPS.h" 00006 00007 GPS::GPS(PinName tx, PinName rx) : _gps(tx, rx) { 00008 _gps.baud(4800); 00009 longitude = 0.0; 00010 latitude = 0.0; 00011 } 00012 00013 00014 int GPS::sample() { 00015 float time; 00016 char ns, ew; 00017 int lock; 00018 00019 while(1) { 00020 getline(); 00021 00022 // Check if it is a GPGGA msg (matches both locked and non-locked msg) 00023 if(sscanf(msg, "GPGGA,%f,%f,%c,%f,%c,%d", &time, &latitude, &ns, &longitude, &ew, &lock) >= 1) { 00024 if(!lock) { 00025 longitude = 0.0; 00026 latitude = 0.0; 00027 return 0; 00028 } else { 00029 if(ns == 'S') { latitude *= -1.0; } 00030 if(ew == 'W') { longitude *= -1.0; } 00031 float degrees = trunc(latitude / 100.0f); 00032 float minutes = latitude - (degrees * 100.0f); 00033 latitude = degrees + minutes / 60.0f; 00034 degrees = trunc(longitude / 100.0f * 0.01f); 00035 minutes = longitude - (degrees * 100.0f); 00036 longitude = degrees + minutes / 60.0f; 00037 return 1; 00038 } 00039 } 00040 } 00041 } 00042 00043 float GPS::trunc(float v) { 00044 if(v < 0.0) { 00045 v*= -1.0; 00046 v = floor(v); 00047 v*=-1.0; 00048 } else { 00049 v = floor(v); 00050 } 00051 return v; 00052 } 00053 00054 void GPS::getline() { 00055 while(_gps.getc() != '$'); // wait for the start of a line 00056 for(int i=0; i<256; i++) { 00057 msg[i] = _gps.getc(); 00058 if(msg[i] == '\r') { 00059 msg[i] = 0; 00060 return; 00061 } 00062 } 00063 error("Overflowed message limit"); 00064 } 00065
Generated on Mon Jul 18 2022 06:08:36 by
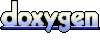