
A simple demonstration of the DISCO-F746NG LCD, TouchScreen and MCP3221 I2C ADC.
Dependencies: BSP_DISCO_F746NG LCD_DISCO_F746NG MCP3221 TS_DISCO_F746NG mbed
Fork of DISCO-F746NG_LCD_demo by
main.cpp
00001 #include "mbed.h" 00002 #include "LCD_DISCO_F746NG.h" 00003 #include "TS_DISCO_F746NG.h" 00004 #include "MCP3221.h" 00005 #include "Peter.h" 00006 00007 #define MCP3221_REF 5.12 /* MCP3221 ADC reference (supply) voltage */ 00008 00009 LCD_DISCO_F746NG lcd; 00010 TS_DISCO_F746NG ts; 00011 DigitalOut led1(LED1); 00012 MCP3221 adc(PB_9, PB_8, MCP3221_REF); // sda, scl, reference (supply) voltage 00013 00014 int main() 00015 { 00016 TS_StateTypeDef TS_State; 00017 uint8_t text[30]; 00018 00019 led1 = 1; 00020 00021 // Display bitmap image 00022 lcd.Clear(LCD_COLOR_WHITE); 00023 lcd.SetBackColor(LCD_COLOR_WHITE); 00024 lcd.DrawBitmap(0, 0, (uint8_t *)peter_file); 00025 00026 // Display circle 00027 lcd.SetTextColor(LCD_COLOR_RED); 00028 lcd.FillCircle(50, 200, 40); 00029 00030 // Set display text colours 00031 lcd.SetBackColor(LCD_COLOR_DARKGREEN); 00032 lcd.SetTextColor(LCD_COLOR_LIGHTGRAY); 00033 00034 while(1) { 00035 00036 // Display touch message 00037 ts.GetState(&TS_State); 00038 if (TS_State.touchDetected) { 00039 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)" TOUCH DETECTED! ", CENTER_MODE); 00040 } else { 00041 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)" NO TOUCH DETECTED ", CENTER_MODE); 00042 } 00043 00044 // Read and scale ADC result 00045 sprintf((char*)text, " ADC=%.3f ", adc.read()); 00046 // sprintf((char*)text, " ADC=%.3f ", 1.234); 00047 lcd.DisplayStringAt(0, LINE(8), (uint8_t *)&text, CENTER_MODE); 00048 00049 led1 = !led1; 00050 } 00051 }
Generated on Sat Jul 23 2022 03:33:38 by
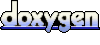