
ActiveGames Week 1 interface
Dependencies: MMA8451QASD USBDeviceASD mbed
Fork of FTF2014_lab3_part2 by
main.cpp
00001 #include "MMA8451Q.h" 00002 #include "mbed.h" 00003 #include "USBMouseKeyboard.h" 00004 00005 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00006 00007 DigitalOut myled(LED1); 00008 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00009 USBMouse joystick; 00010 AnalogIn ainX(PTB0); 00011 AnalogIn ainY(PTB1); 00012 Serial serialPort(PTD3, PTD2); // tx, rx 00013 int ledState = 0; 00014 int x = 128, y = 128, xOld = 0, yOld = 0; 00015 int updateFlag = 0; 00016 00017 void invertLed() { 00018 if(ledState == 0) ledState = 1; 00019 else ledState = 0; 00020 myled = ledState; 00021 } 00022 00023 void rxIrq() { 00024 serialPort.scanf("%d %d",&x,&y); 00025 invertLed(); 00026 updateFlag = 1; 00027 } 00028 00029 int main(void) { 00030 joystick.move(128, 128); 00031 00032 00033 // IF you want to get the data from serial port, uncomment this part 00034 // serial data rate is 9600 bps. send data to PTD2 00035 // give two integers separated by space. First one is X and the second one is Y. 00036 // 128 128 is at center, 0 0 is some corner and 255 255 is the diagonal corner. 00037 00038 serialPort.attach(&rxIrq); 00039 while (true) { 00040 if(updateFlag) { 00041 updateFlag = 0; 00042 xOld = x; 00043 yOld = y; 00044 joystick.move(x, y); 00045 00046 } 00047 else 00048 { 00049 joystick.move(xOld, yOld); 00050 } 00051 wait(0.08); 00052 } 00053 00054 00055 // IF you are using the built-in acceleration sensor of the FRDM-KL25Z, uncomment this part 00056 /* 00057 while (true) { 00058 x = (acc.getAccXX() + 4096)/32; 00059 y = (acc.getAccYY() + 4096)/32; 00060 x = x + 128; 00061 y = y + 128; 00062 wait(0.1); 00063 } 00064 */ 00065 00066 00067 00068 // IF you are using the analog input of the FRDM-KL25Z, uncomment this part 00069 /* 00070 while (true) { 00071 x = ainX.read() * 256; 00072 y = ainY.read() * 256; 00073 x = x + 128; 00074 y = y + 128; 00075 wait(0.1); 00076 } 00077 */ 00078 00079 }
Generated on Mon Jul 18 2022 17:59:27 by
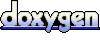