The modified AndroidAccessory Library
Dependents: ADKTerm droidcycle uva_nc androidservo ... more
USBHost.h
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 00025 #ifndef USBHOST_H 00026 #define USBHOST_H 00027 00028 //log mode on off 00029 //#define USBHOST_LOG 00030 00031 00032 #ifndef u8 00033 typedef unsigned char u8; 00034 typedef unsigned short u16; 00035 typedef unsigned long u32; 00036 00037 typedef char s8; 00038 typedef short s16; 00039 typedef char s32; 00040 #endif 00041 00042 #define ENDPOINT_CONTROL 0 00043 #define ENDPOINT_ISOCRONOUS 1 00044 #define ENDPOINT_BULK 2 00045 #define ENDPOINT_INTERRUPT 3 00046 00047 #define DESCRIPTOR_TYPE_DEVICE 1 00048 #define DESCRIPTOR_TYPE_CONFIGURATION 2 00049 #define DESCRIPTOR_TYPE_STRING 3 00050 #define DESCRIPTOR_TYPE_INTERFACE 4 00051 #define DESCRIPTOR_TYPE_ENDPOINT 5 00052 00053 #define DESCRIPTOR_TYPE_HID 0x21 00054 #define DESCRIPTOR_TYPE_REPORT 0x22 00055 #define DESCRIPTOR_TYPE_PHYSICAL 0x23 00056 #define DESCRIPTOR_TYPE_HUB 0x29 00057 00058 enum USB_CLASS_CODE 00059 { 00060 CLASS_DEVICE, 00061 CLASS_AUDIO, 00062 CLASS_COMM_AND_CDC_CONTROL, 00063 CLASS_HID, 00064 CLASS_PHYSICAL = 0x05, 00065 CLASS_STILL_IMAGING, 00066 CLASS_PRINTER, 00067 CLASS_MASS_STORAGE, 00068 CLASS_HUB, 00069 CLASS_CDC_DATA, 00070 CLASS_SMART_CARD, 00071 CLASS_CONTENT_SECURITY = 0x0D, 00072 CLASS_VIDEO = 0x0E, 00073 CLASS_DIAGNOSTIC_DEVICE = 0xDC, 00074 CLASS_WIRELESS_CONTROLLER = 0xE0, 00075 CLASS_MISCELLANEOUS = 0xEF, 00076 CLASS_APP_SPECIFIC = 0xFE, 00077 CLASS_VENDOR_SPECIFIC = 0xFF 00078 }; 00079 00080 #define DEVICE_TO_HOST 0x80 00081 #define HOST_TO_DEVICE 0x00 00082 #define REQUEST_TYPE_STANDARD 0x00 00083 #define REQUEST_TYPE_CLASS 0x20 00084 #define REQUEST_TYPE_VENDOR 0x40 00085 #define RECIPIENT_DEVICE 0x00 00086 #define RECIPIENT_INTERFACE 0x01 00087 #define RECIPIENT_ENDPOINT 0x02 00088 #define RECIPIENT_OTHER 0x03 00089 00090 #define GET_STATUS 0 00091 #define CLEAR_FEATURE 1 00092 #define SET_FEATURE 3 00093 #define SET_ADDRESS 5 00094 #define GET_DESCRIPTOR 6 00095 #define SET_DESCRIPTOR 7 00096 #define GET_CONFIGURATION 8 00097 #define SET_CONFIGURATION 9 00098 #define GET_INTERFACE 10 00099 #define SET_INTERFACE 11 00100 #define SYNCH_FRAME 11 00101 00102 /* HID Request Codes */ 00103 #define HID_REQUEST_GET_REPORT 0x01 00104 #define HID_REQUEST_GET_IDLE 0x02 00105 #define HID_REQUEST_GET_PROTOCOL 0x03 00106 #define HID_REQUEST_SET_REPORT 0x09 00107 #define HID_REQUEST_SET_IDLE 0x0A 00108 #define HID_REQUEST_SET_PROTOCOL 0x0B 00109 00110 /* HID Report Types */ 00111 #define HID_REPORT_INPUT 0x01 00112 #define HID_REPORT_OUTPUT 0x02 00113 #define HID_REPORT_FEATURE 0x03 00114 00115 00116 // -5 is nak 00117 /* 00118 0010 ACK Handshake 00119 1010 NAK Handshake 00120 1110 STALL Handshake 00121 0110 NYET (No Response Yet) 00122 */ 00123 00124 #define IO_PENDING -100 00125 #define ERR_ENDPOINT_NONE_LEFT -101 00126 #define ERR_ENDPOINT_NOT_FOUND -102 00127 #define ERR_DEVICE_NOT_FOUND -103 00128 #define ERR_DEVICE_NONE_LEFT -104 00129 #define ERR_HUB_INIT_FAILED -105 00130 #define ERR_INTERFACE_NOT_FOUND -106 00131 00132 typedef struct 00133 { 00134 u8 bLength; 00135 u8 bDescriptorType; 00136 u16 bcdUSB; 00137 u8 bDeviceClass; 00138 u8 bDeviceSubClass; 00139 u8 bDeviceProtocol; 00140 u8 bMaxPacketSize; 00141 u16 idVendor; 00142 u16 idProduct; 00143 u16 bcdDevice; // version 00144 u8 iManufacturer; 00145 u8 iProduct; 00146 u8 iSerialNumber; 00147 u8 bNumConfigurations; 00148 } DeviceDescriptor; // 16 bytes 00149 00150 typedef struct 00151 { 00152 u8 bLength; 00153 u8 bDescriptorType; 00154 u16 wTotalLength; 00155 u8 bNumInterfaces; 00156 u8 bConfigurationValue; // Value to use as an argument to select this configuration 00157 u8 iConfiguration; // Index of String Descriptor describing this configuration 00158 u8 bmAttributes; // Bitmap D7 Reserved, set to 1. (USB 1.0 Bus Powered),D6 Self Powered,D5 Remote Wakeup,D4..0 = 0 00159 u8 bMaxPower; // Maximum Power Consumption in 2mA units 00160 } ConfigurationDescriptor; 00161 00162 typedef struct 00163 { 00164 u8 bLength; 00165 u8 bDescriptorType; 00166 u8 bInterfaceNumber; 00167 u8 bAlternateSetting; 00168 u8 bNumEndpoints; 00169 u8 bInterfaceClass; 00170 u8 bInterfaceSubClass; 00171 u8 bInterfaceProtocol; 00172 u8 iInterface; // Index of String Descriptor Describing this interface 00173 } InterfaceDescriptor; 00174 00175 typedef struct 00176 { 00177 u8 bLength; 00178 u8 bDescriptorType; 00179 u8 bEndpointAddress; // Bits 0:3 endpoint, Bits 7 Direction 0 = Out, 1 = In (Ignored for Control Endpoints) 00180 u8 bmAttributes; // Bits 0:1 00 = Control, 01 = Isochronous, 10 = Bulk, 11 = Interrupt 00181 u16 wMaxPacketSize; 00182 u8 bInterval; // Interval for polling endpoint data transfers. 00183 } EndpointDescriptor; 00184 00185 typedef struct { 00186 u8 bLength; 00187 u8 bDescriptorType; 00188 u16 bcdHID; 00189 u8 bCountryCode; 00190 u8 bNumDescriptors; 00191 u8 bDescriptorType2; 00192 u16 wDescriptorLength; 00193 } HIDDescriptor; 00194 00195 //============================================================================ 00196 //============================================================================ 00197 00198 00199 void USBInit(); 00200 void USBLoop(); 00201 u8* USBGetBuffer(u32* len); 00202 00203 // Optional callback for transfers, called at interrupt time 00204 typedef void (*USBCallback)(int device, int endpoint, int status, u8* data, int len, void* userData); 00205 00206 // Transfers 00207 int USBControlTransfer(int device, int request_type, int request, int value, int index, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00208 int USBInterruptTransfer(int device, int ep, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00209 int USBBulkTransfer(int device, int ep, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00210 00211 // Standard Device methods 00212 int GetDescriptor(int device, int descType, int descIndex, u8* data, int length); 00213 int GetString(int device, int index, char* dst, int length); 00214 int SetAddress(int device, int new_addr); 00215 int SetConfiguration(int device, int configNum); 00216 int SetInterface(int device, int ifNum, int altNum); 00217 00218 // Implemented to notify app of the arrival of a device 00219 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc); 00220 00221 #endif
Generated on Tue Jul 12 2022 15:55:45 by
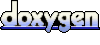