
Mortal Kombat Game ELEC2645
Dependencies: mbed N5110 ShiftReg Joystick
Menu.cpp
00001 #include "Menu.h" 00002 #include "mbed.h" 00003 #include "ShiftReg.h" 00004 ShiftReg shift; // for countdown 00005 00006 Menu::Menu() {} 00007 00008 void Menu::menu_render(N5110 &lcd) { 00009 // this function renders all the tutorial screens and creates a countdown to the fight 00010 draw_uni_logo(lcd); 00011 wait(3.0f); 00012 main_menu(lcd); 00013 wait(3.0f); 00014 created_by(lcd); 00015 wait(3.0f); 00016 tutorial_screen1(lcd); 00017 wait(3.0f); 00018 tutorial_screen3(lcd); 00019 wait(3.0f); 00020 tutorial_screen4(lcd); 00021 wait(3.0f); 00022 tutorial_screen5(lcd); 00023 wait(3.0f); 00024 tutorial_screen6(lcd); 00025 wait(3.0f); 00026 tutorial_screen7(lcd); 00027 wait(3.0f); 00028 tutorial_screen8(lcd); 00029 wait(1.5f); 00030 shift.write(0x4F); 00031 wait(1.0f); 00032 shift.write(0x5B); 00033 wait(1.0f); 00034 shift.write(0x06); 00035 wait(1.0f); 00036 shift.write(0x3F); 00037 wait(0.3f); 00038 tutorial_screen9(lcd); 00039 wait(0.5f); 00040 lcd.refresh(); 00041 } 00042 00043 00044 void Menu::draw_logo(N5110 &lcd, int x, int y) { 00045 // Mortal Kombat Logo 00046 const int logo[17][19] = { 00047 { 1,0,0,0,0,0,0,0,1,1,1,1,0,0,0,0,0,0,1 }, 00048 { 0,0,0,0,0,0,1,1,0,0,0,0,1,1,0,0,0,0,0 }, 00049 { 0,0,0,0,0,1,0,1,1,1,0,0,0,0,1,0,0,0,0 }, 00050 { 0,0,0,0,1,0,1,1,1,1,1,0,0,0,0,1,0,0,0 }, 00051 { 0,0,0,1,0,1,1,0,0,1,1,1,1,0,0,0,1,0,0 }, 00052 { 0,0,1,0,1,1,1,0,1,1,1,1,1,1,0,0,0,1,0 }, 00053 { 0,0,1,0,1,1,1,1,1,1,1,1,0,1,0,0,0,1,0 }, 00054 { 0,0,1,0,1,1,1,0,0,0,1,1,1,1,1,0,0,1,0 }, 00055 { 0,0,1,0,1,1,1,1,0,0,0,0,0,1,1,1,0,1,0 }, 00056 { 0,0,1,0,1,1,1,1,1,1,0,0,0,0,0,0,0,1,0 }, 00057 { 0,0,1,0,0,1,1,1,1,1,1,1,1,1,1,0,0,1,0 }, 00058 { 0,0,1,0,0,1,1,1,1,1,1,1,1,1,1,1,0,1,0 }, 00059 { 0,0,0,1,0,1,1,1,1,1,1,1,1,1,1,1,1,0,0 }, 00060 { 0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,0,0,0 }, 00061 { 0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,0,0,0,0 }, 00062 { 0,0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,0,0,0 }, 00063 { 1,0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,1 }, 00064 }; 00065 // draw the MK Logo 00066 // (x, y, rows, cols, sprite) 00067 lcd.drawSprite(x,y,17,19,(int *)logo); 00068 } 00069 00070 void Menu::main_menu(N5110 &lcd) { 00071 lcd.clear(); 00072 // Printing the first menu screen 00073 lcd.printString("MORTAL KOMBAT",3,1); 00074 lcd.printString("LEEDS EDITION",3,2); 00075 lcd.refresh(); 00076 draw_logo(lcd, 30, 28); // draw logo on (30,28) 00077 // draw menu frame using the whole dimensions of the screen 00078 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00079 lcd.refresh(); // refresh the LCD so the pixels appear 00080 } 00081 00082 void Menu::created_by(N5110 &lcd) { 00083 lcd.clear(); 00084 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00085 lcd.printString("Created by:",2,1); 00086 lcd.printString("OSMAN",25,2); 00087 lcd.printString("FADL ALI",20,3); 00088 lcd.printString("201337691",18,4); 00089 lcd.refresh(); 00090 } 00091 00092 void Menu::tutorial_screen1(N5110 &lcd) { 00093 lcd.clear(); 00094 lcd.printString("Welcome!", 15,0); 00095 lcd.printString("Your mission", 5,2); 00096 lcd.printString("is to", 20,3); 00097 lcd.printString("defeat", 20,4); 00098 lcd.printString("Kotal Khan...", 5,5); 00099 lcd.refresh(); 00100 } 00101 00102 void Menu::tutorial_screen2 (N5110 &lcd) { 00103 lcd.clear(); 00104 lcd.printString("God of Sun!", 5,1); 00105 lcd.printString("God of War!", 5,3); 00106 lcd.printString("God of Blood!", 5,5); 00107 lcd.refresh(); 00108 } 00109 00110 void Menu::tutorial_screen3 (N5110 &lcd) { 00111 lcd.clear(); 00112 lcd.printString("You must", 15,0); 00113 lcd.printString("familiarise", 8,1); 00114 lcd.printString("yourself", 15,2); 00115 lcd.printString("with the", 15,3); 00116 lcd.printString("fight moves", 8,4); 00117 lcd.refresh(); 00118 } 00119 00120 void Menu::tutorial_screen4 (N5110 &lcd) { 00121 lcd.clear(); 00122 lcd.printString("Press A", 18,1); 00123 lcd.printString("to Kick!", 14,2); 00124 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00125 // setting coordinates 00126 _fighter.set_y(34); 00127 _fighter.set_x(34); 00128 _fighter.kick_right(lcd); 00129 lcd.refresh(); 00130 } 00131 00132 void Menu::tutorial_screen5 (N5110 &lcd) { 00133 lcd.clear(); 00134 lcd.printString("Press B", 18,1); 00135 lcd.printString("to Punch!", 14,2); 00136 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00137 _fighter.set_y(34); 00138 _fighter.set_x(34); 00139 _fighter.punch_right(lcd); 00140 lcd.refresh(); 00141 } 00142 00143 void Menu::tutorial_screen6 (N5110 &lcd) { 00144 lcd.clear(); 00145 lcd.printString("Press C", 18,1); 00146 lcd.printString("to Guard!", 14,2); 00147 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00148 _fighter.set_y(34); 00149 _fighter.set_x(34); 00150 _fighter.guard(lcd); 00151 lcd.refresh(); 00152 } 00153 00154 void Menu::tutorial_screen7 (N5110 &lcd) { 00155 lcd.clear(); 00156 lcd.printString("Press D", 18,1); 00157 lcd.printString("to Jump/Fly!", 10,2); 00158 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00159 _fighter.set_y(26); 00160 _fighter.set_x(34); 00161 _fighter.draw(lcd); 00162 lcd.refresh(); 00163 } 00164 00165 void Menu::tutorial_screen8 (N5110 &lcd) { 00166 lcd.clear(); 00167 lcd.printString("Get Ready...", 8,2); 00168 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00169 lcd.refresh(); 00170 } 00171 00172 void Menu::tutorial_screen9 (N5110 &lcd) { 00173 lcd.clear(); 00174 lcd.printString("FIGHT!", 25,2); 00175 lcd.drawRect(0,0,84,48,FILL_TRANSPARENT); 00176 lcd.refresh(); 00177 } 00178 00179 00180 00181 00182 00183 00184 00185 00186 00187 00188 00189 00190 void Menu::draw_uni_logo(N5110 &lcd) { 00191 lcd.clear(); 00192 const int uni_logo[48][84] = { 00193 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00194 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00195 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00196 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00197 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00198 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00199 {1,1,1,1,0,1,1,1,0,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00200 {1,1,1,1,0,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00201 {1,1,1,1,0,1,1,1,0,1,0,0,0,0,1,1,0,1,1,0,1,1,1,1,0,1,1,0,0,0,1,1,0,0,0,1,1,0,0,0,0,1,0,1,0,0,0,1,0,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00202 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,0,1,1,0,1,1,0,1,1,1,0,1,0,1,1,1,0,1,1,1,1,1,0,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00203 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,0,1,1,0,1,1,0,0,0,0,0,1,0,1,1,1,1,0,0,0,1,1,0,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00204 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,1,0,0,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,0,1,0,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00205 {1,1,1,1,1,0,0,0,1,1,0,1,1,1,0,1,0,1,1,1,1,0,0,1,1,1,1,0,0,0,0,1,0,1,1,1,0,0,0,0,1,1,0,1,1,0,0,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00206 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00207 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00208 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00209 {1,1,1,1,1,1,1,1,1,1,0,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,1,0,1,0,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00210 {1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,1,0,1,0,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00211 {1,1,1,1,1,0,0,0,1,0,0,0,1,1,1,1,1,0,1,1,1,1,1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,0,1,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00212 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,0,1,1,1,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00213 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,0,1,1,1,1,0,0,0,0,0,1,0,0,0,0,0,1,0,1,1,1,0,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00214 {1,1,1,1,0,1,1,1,0,1,0,1,1,1,1,1,1,0,1,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,0,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00215 {1,1,1,1,1,0,0,0,1,1,0,1,1,1,1,1,1,0,0,0,0,1,1,0,0,0,0,1,1,0,0,0,0,1,1,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00216 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,1,0,1,1,0,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00217 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00218 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00219 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00220 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00221 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00222 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,1,0,0,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1}, 00223 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1}, 00224 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1}, 00225 {1,1,1,1,0,0,0,0,0,1,1,0,1,1,1,1,1,0,0,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,1,1,1,1,1,0,1,1,1,0,0,0,0,1,1,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1}, 00226 {1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,0,1,0,1,1,1,0,1,0,1,1,1,0,1,1,1,0,0,1,1,1,0,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00227 {1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0,1,0,1,1,1,1,1,1,0,1,0,1,1,0,0,0,0,1,1,1,1,1,0,0,0,0,1,0,0,1,1,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00228 {1,1,1,1,0,0,0,0,0,1,1,0,1,1,1,1,1,0,0,0,0,0,1,1,0,1,1,1,1,1,1,1,1,0,1,1,0,0,0,0,1,1,1,0,1,0,1,1,0,1,1,1,0,1,1,1,1,0,0,0,0,1,0,1,1,0,1,1,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00229 {1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,1,1,0,1,1,1,0,1,1,1,0,1,0,1,1,0,1,1,1,1,1,1,0,1,1,1,1,0,0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00230 {1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,1,1,0,1,1,1,1,1,1,0,1,1,1,0,1,1,0,1,1,1,1,0,1,1,1,0,1,0,0,0,0,0,1,1,1,1,1,0,1,1,1,1,0,0,0,0,1,0,1,1,0,1,1,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00231 {1,1,1,1,0,0,0,0,0,1,1,0,0,0,0,1,1,0,0,0,0,0,1,1,1,0,0,0,1,1,0,0,0,0,0,1,1,0,0,0,1,1,1,1,1,0,1,1,0,0,0,0,1,1,1,1,1,0,0,0,0,1,0,0,1,1,1,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00232 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00233 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00234 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00235 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00236 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00237 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00238 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00239 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1}, 00240 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,1,1,1,1,1,1,1,1,1,1} 00241 }; 00242 lcd.drawSprite(0,0,48,84,(int *)uni_logo); 00243 lcd.refresh(); 00244 } 00245 00246 00247 00248
Generated on Fri Jul 29 2022 14:20:18 by
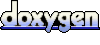