
f429-disc1
Dependencies: LCD_DISCO_F429ZI mbed BSP_DISCO_F429ZI
main.cpp
00001 #include "mbed.h" 00002 #include "LCD_DISCO_F429ZI.h" 00003 #include "stlogo.h" 00004 00005 /** 00006 * @brief Creates uint32_t ARGB color variable from separate uint8_t values 00007 * @param a: Alpha channel 00008 * @param r: Red color value 00009 * @param g: Green color value 00010 * @param b: Blue color value 00011 * @retval Color value bytes combined in uint32_t 00012 */ 00013 uint32_t colorFromSeparateValues(uint8_t a,uint8_t r,uint8_t g, uint8_t b); 00014 00015 LCD_DISCO_F429ZI lcd; 00016 00017 int main(){ 00018 uint32_t xSize = lcd.GetXSize(); 00019 uint32_t ySize = lcd.GetYSize(); 00020 00021 lcd.Clear(LCD_COLOR_BLACK); 00022 lcd.SelectLayer(0); 00023 lcd.DrawBitmap(xSize/2 - 30,ySize/2 - 20,stlogo); 00024 lcd.SelectLayer(1); 00025 lcd.SetTextColor(LCD_COLOR_BLACK); 00026 lcd.FillRect(0,0,xSize, ySize); 00027 lcd.SelectLayer(0); 00028 00029 uint8_t procIdentifier = 0; 00030 uint8_t frontLayerTransparency = 0x00; 00031 00032 while(1){ 00033 if(procIdentifier % 2) { 00034 --frontLayerTransparency; 00035 } 00036 else { 00037 ++frontLayerTransparency; 00038 } 00039 lcd.SetTransparency(1, frontLayerTransparency); 00040 00041 if(frontLayerTransparency == 0x00 || frontLayerTransparency == 0xff){ 00042 ++procIdentifier; 00043 procIdentifier = procIdentifier % 4; 00044 00045 if(procIdentifier == 0){ 00046 lcd.Clear(LCD_COLOR_BLACK); 00047 lcd.DrawBitmap(xSize/2 - 30,ySize/2 - 20,stlogo); 00048 } else if(procIdentifier == 2){ 00049 lcd.SetTextColor(LCD_COLOR_GREEN); 00050 lcd.FillRect(0,0,xSize, ySize); 00051 } 00052 } 00053 wait_ms(5); 00054 } 00055 } 00056 00057 uint32_t colorFromSeparateValues(uint8_t a,uint8_t r,uint8_t g, uint8_t b) { 00058 uint32_t res = a; 00059 res = (res >> 8) + r; 00060 res = (res >> 8) + g; 00061 res = (res >> 8) + b; 00062 return res; 00063 }
Generated on Tue Aug 16 2022 22:32:02 by
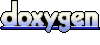