NUCLEO-F401RE + BlueNRG shield client test (TI Sensortag reading)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "tag_math.h" 00003 #include "stm32f4xx_hal.h" 00004 #include "bluenrg_shield_bsp.h" 00005 #include "osal.h" 00006 #include "sample_service.h" 00007 #include <stdio.h> 00008 00009 #define BDADDR_SIZE 6 00010 00011 /* SPI handler declaration */ 00012 SPI_HandleTypeDef SpiHandle; 00013 00014 Serial pc(SERIAL_TX, SERIAL_RX); 00015 00016 extern volatile uint8_t set_connectable; 00017 extern volatile int connected; 00018 extern volatile uint8_t notification_enabled; 00019 00020 void User_Process(void); 00021 00022 00023 /******************************************************************************/ 00024 /******************************************************************************/ 00025 int main(void) 00026 { 00027 tHalUint8 CLIENT_BDADDR[] = {0xbb, 0x00, 0x00, 0xE1, 0x80, 0x02}; 00028 00029 tHalUint8 bdaddr[BDADDR_SIZE]; 00030 00031 uint16_t service_handle, dev_name_char_handle, appearance_char_handle; 00032 int ret; 00033 00034 /* Hardware init*/ 00035 HAL_Init(); 00036 00037 /* Configure the system clock */ 00038 SystemClock_Config(); 00039 00040 /* Initialize the BlueNRG SPI driver */ 00041 BNRG_SPI_Init(); 00042 00043 /* Initialize the BlueNRG HCI */ 00044 HCI_Init(); 00045 00046 /* Reset BlueNRG hardware */ 00047 BlueNRG_RST(); 00048 00049 Osal_MemCpy(bdaddr, CLIENT_BDADDR, sizeof(CLIENT_BDADDR)); 00050 00051 ret = aci_hal_write_config_data(CONFIG_DATA_PUBADDR_OFFSET, 00052 CONFIG_DATA_PUBADDR_LEN, 00053 bdaddr); 00054 if(ret){ 00055 pc.printf("Setting BD_ADDR failed.\n"); 00056 } 00057 00058 ret = aci_gatt_init(); 00059 if(ret){ 00060 pc.printf("GATT_Init failed.\n"); 00061 } 00062 00063 ret = aci_gap_init(GAP_CENTRAL_ROLE, &service_handle, &dev_name_char_handle, &appearance_char_handle); 00064 00065 if(ret != BLE_STATUS_SUCCESS){ 00066 pc.printf("GAP_Init failed.\n"); 00067 } 00068 00069 ret = aci_gap_set_auth_requirement(MITM_PROTECTION_REQUIRED, 00070 OOB_AUTH_DATA_ABSENT, 00071 NULL, 00072 7, 00073 16, 00074 USE_FIXED_PIN_FOR_PAIRING, 00075 123456, 00076 BONDING); 00077 00078 00079 if (ret != BLE_STATUS_SUCCESS) { 00080 pc.printf("BLE Stack Initialization failed.\n"); 00081 } 00082 00083 /* Set output power level */ 00084 ret = aci_hal_set_tx_power_level(1,4); 00085 00086 /* Infinite loop */ 00087 while (1) 00088 { 00089 HCI_Process(); 00090 User_Process(); 00091 } 00092 00093 } 00094 00095 /******************************************************************************/ 00096 /******************************************************************************/ 00097 00098 void User_Process(void) 00099 { 00100 static uint32_t cnt; 00101 static uint8_t acc_en_sent = 0; 00102 tHalUint8 data[2]; 00103 //uint8_t data_length; 00104 00105 if(set_connectable){ 00106 Make_Connection(); 00107 set_connectable = FALSE; 00108 } 00109 00110 /*if(connected && !notification_enabled) { 00111 enableNotification(); 00112 }*/ 00113 00114 if((connected)&&(!acc_en_sent)){ 00115 00116 HAL_Delay(100); 00117 data[0] = 0x01; 00118 sendData(0x0029, data, 1); 00119 00120 HAL_Delay(100); 00121 data[0] = 0x01; 00122 sendData(0x0031, data, 1); 00123 00124 HAL_Delay(100); 00125 data[0] = 0x01; 00126 sendData(0x003C, data, 1); 00127 00128 HAL_Delay(100); 00129 data[0] = 0x10; 00130 sendData(0x0034, data, 1); 00131 00132 00133 acc_en_sent = 1; 00134 cnt = HAL_GetTick(); 00135 } 00136 00137 if (HAL_GetTick() > (cnt + 2000)) 00138 { 00139 cnt = HAL_GetTick(); 00140 if (connected && acc_en_sent) 00141 { 00142 00143 pc.printf("T = %f; ", calcTmpTarget(readValue(0x25, NULL))); 00144 uint8_t* temp = readValue(0x2D, NULL); 00145 pc.printf("Ax = %d; Ay = %d; Az = %d ", (signed char) temp[0], (signed char) temp[1], (signed char) temp[2]); 00146 temp = readValue(0x38, NULL); 00147 pc.printf("H = %f; \r\n", calcHumRel(temp)); 00148 00149 00150 //GATT services scan 00151 /*for (unsigned char j = 1; j < 0x88; j++) 00152 { 00153 pc.printf("\n %.2X: ", j); 00154 uint8_t* temp = readValue(j+1, &data_length); 00155 00156 00157 for(int i = 0; i < data_length; i++) { 00158 pc.printf("%.2X:", temp[i]); 00159 } 00160 00161 for(int i = 0; i < data_length; i++) { 00162 pc.printf("%c", temp[i]); 00163 } 00164 00165 } 00166 */ 00167 00168 } 00169 } 00170 00171 } 00172 /******************************************************************************/ 00173 /******************************************************************************/ 00174 00175 /*********************** END OF FILE ******************************************/
Generated on Tue Jul 12 2022 20:44:37 by
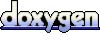