
Final Project for ECE-4180
Dependencies: mbed Servo mbed-rtos 4DGL-uLCD-SE PinDetect
main.cpp
00001 #include "mbed.h" 00002 #include "uLCD_4DGL.h" 00003 #include "PinDetect.h" 00004 #include "rtos.h" 00005 #include "Servo.h" 00006 00007 uLCD_4DGL uLCD(p9,p10,p11); // serial tx, serial rx, reset pin; 00008 Serial pc(USBTX, USBRX); // tx, rx 00009 00010 // pb 00011 PinDetect button1(p7,PullDown); 00012 PinDetect button2(p8,PullDown); 00013 00014 // sensors 00015 AnalogIn waterSensor(p20); 00016 AnalogIn moistureSensor(p19); 00017 AnalogIn lightSensor(p18); 00018 00019 // outputs 00020 PwmOut speaker(p21); 00021 PwmOut led(p22); 00022 PwmOut led1(LED1); 00023 // motors 00024 Servo Shade(p23); 00025 Servo Pipe(p24); 00026 00027 00028 Mutex myMut; 00029 volatile int button1_push = 0; 00030 volatile int button2_push = 0; 00031 volatile float setWaterLevel = 0.5; 00032 volatile float setMositLevel = 0.5; 00033 volatile float setLightLevel = 0.5; 00034 volatile float ShadePosition = 0.0; 00035 volatile float ShadePosition = 0.0; 00036 00037 volatile int counting = 0; // keep track of how many seconds has passed 00038 00039 // sensor readings 00040 volatile float water = 0.0; 00041 volatile float light = 0.0; 00042 volatile float moist = 0.0; 00043 00044 // callback functinos for 2 pushbuttons 00045 void Button1_Callback (void) {button1_push = 1;} 00046 void Button2_Callback (void) {button2_push = 1;} 00047 00048 // function for the buttons thread: adjust pre-set light levels 00049 void buttons_function(void const *argument){ 00050 while(1){ 00051 if (button1_push && button2_push) 00052 { 00053 uLCD.cls(); 00054 uLCD.printf("DON'T PRESS THE BUTTON AT THE SAME TIME!\n\r"); 00055 button1_push = button2_push = 0; 00056 uLCD.cls(); 00057 } 00058 if (button1_push) 00059 { 00060 setLightLevel -= .1; 00061 button1_push = 0; 00062 } 00063 if (button2_push) 00064 { 00065 setLightLevel += .1; 00066 button2_push = 0; 00067 } 00068 if (setLightLevel<0) setLightLevel = 0; 00069 if (setLightLevel>1) setLightLevel = 1; 00070 00071 myMut.lock(); 00072 uLCD.locate(0,10); 00073 uLCD.printf("SetLightLevel: %1.1f\n\r", setLightLevel); 00074 myMut.unlock(); 00075 Thread::wait(500); 00076 } 00077 } 00078 00079 // move the servos, also light an LED 00080 void motors_function(void const *argument){ 00081 while(1){ 00082 shadePosition = 1-light; // raise the shade when light 00083 Shade = shadePosition); 00084 led.write(shadePosition) 00085 00086 pipePosition = soil<0.6? 1:0; 00087 pipe = pipePosition; 00088 Thread::wait(1000); 00089 } 00090 } 00091 00092 int main() { 00093 uLCD.printf("\n\rstart printing\n"); 00094 uLCD.cls(); 00095 speaker.period(1.0/800.0); 00096 00097 button1.attach_deasserted(&Button1_Callback); 00098 button1.setSampleFrequency(); 00099 button2.attach_deasserted(&Button2_Callback); 00100 button2.setSampleFrequency(); 00101 00102 Thread buttons(buttons_function); 00103 Thread motors(motors_function); 00104 00105 while(1) { 00106 water = waterSensor.read(); 00107 moist = moistureSensor.read(); 00108 light = lightSensor.read(); 00109 myMut.lock(); 00110 uLCD.locate(0,2); 00111 uLCD.printf("%d:\n\r",counting); 00112 uLCD.printf("water: %f\n\r",water); 00113 uLCD.printf("moist: %f\n\r",moist); 00114 uLCD.printf("light: %f\n\r",light); 00115 myMut.unlock(); 00116 counting++; 00117 00118 // sound the alarm if water level too low 00119 speaker = water<setWaterLevel? 0.5:0; 00120 00121 Thread::wait(1000); 00122 } 00123 }
Generated on Wed Aug 17 2022 08:19:59 by
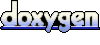