Library for serial logging via macros.
Embed:
(wiki syntax)
Show/hide line numbers
logger.h
00001 #ifndef __LOGGER_H__ 00002 #define __LOGGER_H__ 00003 00004 #include "mbed.h" 00005 00006 #if defined LOG_LEVEL_TRACE 00007 #define TRACE(x, ...) std::printf("[TRACE: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00008 #define DEBUG(x, ...) std::printf("[DEBUG: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00009 #define INFO(x, ...) std::printf("[INFO: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00010 #define WARN(x, ...) std::printf("[WARN: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00011 #define ERROR(x, ...) std::printf("[ERROR: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00012 00013 #elif defined LOG_LEVEL_DEBUG 00014 #define TRACE(x, ...) 00015 #define DEBUG(x, ...) std::printf("[DEBUG: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00016 #define INFO(x, ...) std::printf("[INFO: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00017 #define WARN(x, ...) std::printf("[WARN: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00018 #define ERROR(x, ...) std::printf("[ERROR: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00019 00020 #elif defined LOG_LEVEL_INFO 00021 #define TRACE(x, ...) 00022 #define DEBUG(x, ...) 00023 #define INFO(x, ...) std::printf("[INFO: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00024 #define WARN(x, ...) std::printf("[WARN: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00025 #define ERROR(x, ...) std::printf("[ERROR: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00026 00027 #elif defined LOG_LEVEL_WARN 00028 #define TRACE(x, ...) 00029 #define DEBUG(x, ...) 00030 #define INFO(x, ...) 00031 #define WARN(x, ...) std::printf("[WARN: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00032 #define ERROR(x, ...) std::printf("[ERROR: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00033 00034 #elif defined LOG_LEVEL_ERROR 00035 #define TRACE(x, ...) 00036 #define DEBUG(x, ...) 00037 #define INFO(x, ...) 00038 #define WARN(x, ...) 00039 #define ERROR(x, ...) std::printf("[ERROR: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00040 00041 #else 00042 #define TRACE(x, ...) 00043 #define DEBUG(x, ...) 00044 #define INFO(x, ...) 00045 #define WARN(x, ...) 00046 #define ERROR(x, ...) 00047 #endif 00048 00049 class SerialLogger { 00050 00051 private: 00052 Serial *pc; 00053 00054 public: 00055 SerialLogger() { 00056 pc = new Serial(USBTX, USBRX); 00057 } 00058 00059 void start(int baudRate = 460800) { 00060 pc->baud(baudRate); 00061 } 00062 }; 00063 00064 00065 #endif
Generated on Mon Jul 18 2022 09:42:04 by
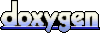