
Kansai Electric Power usage meter (Denki-yohou)
Dependencies: mbed mbed-rtos EthernetInterface
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "TinyHTTP_b.h" 00004 00005 // Kansai Electric Power 00006 #define HTTP_HOST "www.kepco.co.jp" 00007 #define HTTP_URI "/yamasou/juyo1_kansai.csv" 00008 00009 Serial pc(USBTX, USBRX); 00010 EthernetInterface eth; 00011 00012 DigitalOut led1(LED1); 00013 PwmOut led2(LED2), led3(LED3), led4(LED4); 00014 00015 volatile int denki_flg = 0; 00016 volatile int denki_capacity = 0; 00017 volatile int denki_usage = 0; 00018 volatile int year, month, day, hour, minute; 00019 00020 00021 void callback_denkiyohou (char *buf, int len) { 00022 static int n = 0; 00023 static char data[100]; 00024 int i; 00025 00026 for (i = 0; i < len; i ++) { 00027 if (buf[i] == '\r') continue; 00028 00029 if (denki_flg <= 1) { 00030 // header 00031 if (buf[i] == '\n') { 00032 n = 0; 00033 denki_flg ++; 00034 } else { 00035 denki_flg = 0; 00036 } 00037 continue; 00038 } 00039 00040 // body 00041 if (buf[i] == '\n') { 00042 data[n] = 0; 00043 switch (denki_flg) { 00044 case 2: 00045 // update 00046 break; 00047 case 4: 00048 // capacity 00049 if (data[0] >= '0' && data[0] <= '9') { 00050 denki_capacity = atoi(data); 00051 } 00052 break; 00053 case 7: 00054 // yosou1 00055 break; 00056 case 10: 00057 // yosou2 00058 break; 00059 default: 00060 // text 00061 if (data[0] == 'D' && data[1] == 'A') { 00062 denki_flg = denki_flg < 100 ? 100 : 200; 00063 } 00064 break; 00065 } 00066 if (denki_flg > 200 && data[n - 1] >= '0' && data[n - 1] <= '9') { 00067 sscanf(data, "%d/%d/%d,%d:%d,%d", &year, &month, &day, &hour, &minute, &denki_usage); 00068 } 00069 n = 0; 00070 denki_flg ++; 00071 } else { 00072 // data 00073 if (n < sizeof(data) - 1) { 00074 data[n] = buf[i]; 00075 n ++; 00076 } 00077 } 00078 00079 } 00080 } 00081 00082 int main() { 00083 Timer timer; 00084 int flg = 1, r; 00085 float denki_percentage = 0; 00086 00087 pc.baud(115200); 00088 eth.init(); //Use DHCP 00089 if (eth.connect()) { 00090 return -1; 00091 } 00092 00093 pc.printf("Denki-yohou: %s\r\n", HTTP_HOST); 00094 00095 timer.start(); 00096 while(1) { 00097 led1 = 1; 00098 00099 if (flg || timer.read() >= 180) { // 3min 00100 timer.reset(); 00101 denki_flg = 0; 00102 r = httpRequest(METHOD_GET, HTTP_HOST, 80, HTTP_URI, NULL, NULL, &callback_denkiyohou); 00103 if (r == 0) { 00104 denki_percentage = (float)denki_usage / (float)denki_capacity * 100.0; 00105 00106 pc.printf("%04d-%02d-%02d %02d:%02d :", year, month, day, hour, minute); 00107 pc.printf(" %d0 MW / %d0 MW", denki_usage, denki_capacity); 00108 pc.printf(" (%0.1f %%)\r\n", denki_percentage); 00109 00110 led2 = denki_percentage >= 70 ? (denki_percentage >= 77.5 ? 1 : 0.5) : 0; 00111 led3 = denki_percentage >= 85 ? (denki_percentage >= 90 ? 1 : 0.5) : 0; 00112 led4 = denki_percentage >= 95 ? (denki_percentage >= 97 ? 1 : 0.5) : 0; 00113 } else { 00114 pc.printf("http error\r\n"); 00115 } 00116 flg = 0; 00117 } 00118 00119 wait(0.1); 00120 led1 = 0; 00121 wait(0.9); 00122 } 00123 }
Generated on Tue Jul 12 2022 20:05:55 by
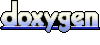