
X-TOUCH to djay bridge
Dependencies: mbed mbed-rtos FATFileSystem
MIDI Class Reference
The main class for MIDI handling.
See member descriptions to know how to use it, or check out the examples supplied with the library.
More...
#include <MIDI.h>
Public Member Functions | |
MIDI (PinName p_tx, PinName p_rx) | |
Default constructor for MIDI. | |
~MIDI () | |
Default destructor for MIDI. | |
void | begin (const byte inChannel=1) |
Call the begin method in the setup() function of the Arduino. | |
void | sendNoteOn (byte NoteNumber, byte Velocity, byte Channel) |
Send a Note On message. | |
void | sendNoteOff (byte NoteNumber, byte Velocity, byte Channel) |
Send a Note Off message (a real Note Off, not a Note On with null velocity) | |
void | sendProgramChange (byte ProgramNumber, byte Channel) |
Send a Program Change message. | |
void | sendControlChange (byte ControlNumber, byte ControlValue, byte Channel) |
Send a Control Change message. | |
void | sendPitchBend (int PitchValue, byte Channel) |
Send a Pitch Bend message using a signed integer value. | |
void | sendPitchBend (unsigned int PitchValue, byte Channel) |
Send a Pitch Bend message using an unsigned integer value. | |
void | sendPitchBend (double PitchValue, byte Channel) |
Send a Pitch Bend message using a floating point value. | |
void | sendPolyPressure (byte NoteNumber, byte Pressure, byte Channel) |
Send a Polyphonic AfterTouch message (applies to only one specified note) | |
void | sendAfterTouch (byte Pressure, byte Channel) |
Send a MonoPhonic AfterTouch message (applies to all notes) | |
void | sendSysEx (int length, const byte *const array, bool ArrayContainsBoundaries=false) |
Generate and send a System Exclusive frame. | |
void | sendTimeCodeQuarterFrame (byte TypeNibble, byte ValuesNibble) |
Send a MIDI Time Code Quarter Frame. | |
void | sendTimeCodeQuarterFrame (byte data) |
Send a MIDI Time Code Quarter Frame. | |
void | sendSongPosition (unsigned int Beats) |
Send a Song Position Pointer message. | |
void | sendSongSelect (byte SongNumber) |
Send a Song Select message. | |
void | sendTuneRequest () |
Send a Tune Request message. | |
void | sendRealTime (kMIDIType Type) |
Send a Real Time (one byte) message. | |
void | send (kMIDIType type, byte param1, byte param2, byte channel) |
Generate and send a MIDI message from the values given. | |
bool | read () |
Read a MIDI message from the serial port using the main input channel (see setInputChannel() for reference). | |
bool | read (const byte Channel) |
Reading/thru-ing method, the same as read() with a given input channel to read on. | |
kMIDIType | getType () const |
Get the last received message's type. | |
byte | getChannel () const |
Get the channel of the message stored in the structure. | |
byte | getData1 () const |
Get the first data byte of the last received message. | |
byte | getData2 () const |
Get the second data byte of the last received message. | |
const byte * | getSysExArray () const |
Get the System Exclusive byte array. | |
unsigned int | getSysExArrayLength () const |
Get the lenght of the System Exclusive array. | |
bool | check () const |
Check if a valid message is stored in the structure. | |
void | setInputChannel (const byte Channel) |
Set the value for the input MIDI channel. | |
void | disconnectCallbackFromType (kMIDIType Type) |
Detach an external function from the given type. | |
void | turnThruOn (kThruFilterMode inThruFilterMode=Full) |
Setter method: turn message mirroring on. | |
void | turnThruOff () |
Setter method: turn message mirroring off. | |
void | setThruFilterMode (const kThruFilterMode inThruFilterMode) |
Set the filter for thru mirroring. | |
Static Public Member Functions | |
static kMIDIType | getTypeFromStatusByte (const byte inStatus) |
Extract an enumerated MIDI type from a status byte. |
Detailed Description
The main class for MIDI handling.
See member descriptions to know how to use it, or check out the examples supplied with the library.
Definition at line 127 of file MIDI.h.
Constructor & Destructor Documentation
MIDI | ( | PinName | p_tx, |
PinName | p_rx | ||
) |
~MIDI | ( | ) |
Member Function Documentation
void begin | ( | const byte | inChannel = 1 ) |
bool check | ( | ) | const |
void disconnectCallbackFromType | ( | kMIDIType | Type ) |
byte getChannel | ( | ) | const |
byte getData1 | ( | ) | const |
byte getData2 | ( | ) | const |
const byte * getSysExArray | ( | ) | const |
Get the System Exclusive byte array.
- See also:
- getSysExArrayLength to get the array's length in bytes.
unsigned int getSysExArrayLength | ( | ) | const |
kMIDIType getType | ( | ) | const |
bool read | ( | const byte | Channel ) |
bool read | ( | ) |
Read a MIDI message from the serial port using the main input channel (see setInputChannel() for reference).
Returned value: true if any valid message has been stored in the structure, false if not. A valid message is a message that matches the input channel.
If the Thru is enabled and the messages matches the filter, it is sent back on the MIDI output.
Generate and send a MIDI message from the values given.
- Parameters:
-
type The message type (see type defines for reference) data1 The first data byte. data2 The second data byte (if the message contains only 1 data byte, set this one to 0). channel The output channel on which the message will be sent (values from 1 to 16). Note: you cannot send to OMNI.
This is an internal method, use it only if you need to send raw data from your code, at your own risks.
Send a Control Change message.
- Parameters:
-
ControlNumber The controller number (0 to 127). See the detailed description here: http://www.somascape.org/midi/tech/spec.html#ctrlnums ControlValue The value for the specified controller (0 to 127). Channel The channel on which the message will be sent (1 to 16).
Send a Note Off message (a real Note Off, not a Note On with null velocity)
- Parameters:
-
NoteNumber Pitch value in the MIDI format (0 to 127). Take a look at the values, names and frequencies of notes here: http://www.phys.unsw.edu.au/jw/notes.html
Velocity Release velocity (0 to 127). Channel The channel on which the message will be sent (1 to 16).
Send a Note On message.
- Parameters:
-
NoteNumber Pitch value in the MIDI format (0 to 127). Take a look at the values, names and frequencies of notes here: http://www.phys.unsw.edu.au/jw/notes.html
Velocity Note attack velocity (0 to 127). A NoteOn with 0 velocity is considered as a NoteOff. Channel The channel on which the message will be sent (1 to 16).
void sendPitchBend | ( | double | PitchValue, |
byte | Channel | ||
) |
Send a Pitch Bend message using a floating point value.
- Parameters:
-
PitchValue The amount of bend to send (in a floating point format), between -1.0f (maximum downwards bend) and +1.0f (max upwards bend), center value is 0.0f. Channel The channel on which the message will be sent (1 to 16).
void sendPitchBend | ( | int | PitchValue, |
byte | Channel | ||
) |
Send a Pitch Bend message using a signed integer value.
- Parameters:
-
PitchValue The amount of bend to send (in a signed integer format), between -8192 (maximum downwards bend) and 8191 (max upwards bend), center value is 0. Channel The channel on which the message will be sent (1 to 16).
void sendPitchBend | ( | unsigned int | PitchValue, |
byte | Channel | ||
) |
Send a Pitch Bend message using an unsigned integer value.
- Parameters:
-
PitchValue The amount of bend to send (in a signed integer format), between 0 (maximum downwards bend) and 16383 (max upwards bend), center value is 8192. Channel The channel on which the message will be sent (1 to 16).
void sendRealTime | ( | kMIDIType | Type ) |
void sendSongPosition | ( | unsigned int | Beats ) |
void sendSongSelect | ( | byte | SongNumber ) |
void sendSysEx | ( | int | length, |
const byte *const | array, | ||
bool | ArrayContainsBoundaries = false |
||
) |
Generate and send a System Exclusive frame.
- Parameters:
-
length The size of the array to send array The byte array containing the data to send ArrayContainsBoundaries When set to 'true', 0xF0 & 0xF7 bytes (start & stop SysEx) will NOT be sent (and therefore must be included in the array). default value is set to 'false' for compatibility with previous versions of the library.
void sendTimeCodeQuarterFrame | ( | byte | data ) |
void sendTuneRequest | ( | ) |
void setInputChannel | ( | const byte | Channel ) |
void setThruFilterMode | ( | const kThruFilterMode | inThruFilterMode ) |
Set the filter for thru mirroring.
- Parameters:
-
inThruFilterMode a filter mode
- See also:
- kThruFilterMode
void turnThruOff | ( | ) |
void turnThruOn | ( | kThruFilterMode | inThruFilterMode = Full ) |
Generated on Wed Jul 13 2022 10:54:56 by
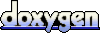