
Weather Meters (Sparkfun) http://mbed.org/users/okini3939/notebook/weatherduino-on-mbed/
Embed:
(wiki syntax)
Show/hide line numbers
WeatherMeters.h
00001 /* 00002 * mbed library to use a Sparkfun Weather Meters 00003 * Copyright (c) 2010 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 #ifndef WeatherMeters_H 00008 #define WeatherMeters_H 00009 00010 #include "mbed.h" 00011 00012 enum Weather_mode { 00013 Weather_manual = 0, 00014 Weather_auto = 1 00015 }; 00016 00017 class WeatherMeters : public Base { 00018 public: 00019 WeatherMeters(PinName p_anemometer, PinName p_windvane, PinName p_raingauge, Weather_mode w_mode = Weather_auto); 00020 00021 float get_windspeed(); 00022 float get_windvane(); 00023 float get_raingauge(); 00024 void update(); 00025 00026 protected: 00027 void int_anemometer (); 00028 void int_raingauge (); 00029 void int_timer (); 00030 00031 InterruptIn int01, int02; 00032 AnalogIn ain01; 00033 Ticker ticker01; 00034 int windspeed, raingauge; 00035 float windvane; 00036 int mode; 00037 00038 private: 00039 00040 int count_anemo, count_rain, time_anemo, time_rain; 00041 int buf_anemo[12], buf_rain[12]; 00042 }; 00043 00044 #endif
Generated on Thu Jul 21 2022 09:22:11 by
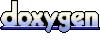