
Dependencies: ChaNFSSD mbed ChaNFS
USBMouse.h
00001 /* USBMouse.h */ 00002 /* USB device example: relative mouse */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef USBMOUSE_H 00006 #define USBMOUSE_H 00007 00008 #include "USBHID.h" 00009 00010 #define REPORT_ID_MOUSE 2 00011 00012 /* Common usage */ 00013 00014 enum MOUSE_BUTTON 00015 { 00016 MOUSE_LEFT = 1, 00017 MOUSE_RIGHT = 2, 00018 MOUSE_MIDDLE = 4, 00019 }; 00020 00021 /* X and Y limits */ 00022 /* These values do not directly map to screen pixels */ 00023 /* Zero may be interpreted as meaning 'no movement' */ 00024 #define X_MIN_ABS (1) /*!< Minimum value on x-axis */ 00025 #define Y_MIN_ABS (1) /*!< Minimum value on y-axis */ 00026 #define X_MAX_ABS (0x7fff) /*!< Maximum value on x-axis */ 00027 #define Y_MAX_ABS (0x7fff) /*!< Maximum value on y-axis */ 00028 00029 #define X_MIN_REL (-127) /*!< The maximum value that we can move to the left on the x-axis */ 00030 #define Y_MIN_REL (-127) /*!< The maximum value that we can move up on the y-axis */ 00031 #define X_MAX_REL (127) /*!< The maximum value that we can move to the right on the x-axis */ 00032 #define Y_MAX_REL (127) /*!< The maximum value that we can move down on the y-axis */ 00033 00034 enum MOUSE_TYPE 00035 { 00036 ABS_MOUSE, 00037 REL_MOUSE, 00038 }; 00039 00040 /** 00041 * 00042 * USBMouse example 00043 * @code 00044 * #include "mbed.h" 00045 * #include "USBMouse.h" 00046 * 00047 * USBMouse mouse; 00048 * 00049 * int main(void) 00050 * { 00051 * while (1) 00052 * { 00053 * mouse.move(20, 0); 00054 * wait(0.5); 00055 * } 00056 * } 00057 * 00058 * @endcode 00059 * 00060 * 00061 * @code 00062 * #include "mbed.h" 00063 * #include "USBMouse.h" 00064 * #include <math.h> 00065 * 00066 * USBMouse mouse(ABS_MOUSE); 00067 * 00068 * int main(void) 00069 * { 00070 * uint16_t x_center = (X_MAX_ABS - X_MIN_ABS)/2; 00071 * uint16_t y_center = (Y_MAX_ABS - Y_MIN_ABS)/2; 00072 * uint16_t x_screen = 0; 00073 * uint16_t y_screen = 0; 00074 * 00075 * uint32_t x_origin = x_center; 00076 * uint32_t y_origin = y_center; 00077 * uint32_t radius = 5000; 00078 * uint32_t angle = 0; 00079 * 00080 * while (1) 00081 * { 00082 * x_screen = x_origin + cos((double)angle*3.14/180.0)*radius; 00083 * y_screen = y_origin + sin((double)angle*3.14/180.0)*radius; 00084 * 00085 * mouse.move(x_screen, y_screen); 00086 * angle += 3; 00087 * wait(0.01); 00088 * } 00089 * } 00090 * 00091 * @endcode 00092 */ 00093 class USBMouse: public USBHID 00094 { 00095 public: 00096 00097 /** 00098 * Constructor 00099 * 00100 * @param mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) 00101 * @param vendor_id Your vendor_id (default: 0x1234) 00102 * @param product_id Your product_id (default: 0x0001) 00103 * @param product_release Your preoduct_release (default: 0x0001) 00104 * 00105 */ 00106 USBMouse(MOUSE_TYPE mouse_type = REL_MOUSE, uint16_t vendor_id = 0x1234, uint16_t product_id = 0x0001, uint16_t product_release = 0x0001): 00107 USBHID(0, 0, vendor_id, product_id, product_release) 00108 { 00109 button = 0; 00110 this->mouse_type = mouse_type; 00111 }; 00112 00113 /** 00114 * Write a state of the mouse 00115 * 00116 * @param x x-axis position 00117 * @param y y-axis position 00118 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00119 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00120 * @returns true if there is no error, false otherwise 00121 */ 00122 bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00123 00124 00125 /** 00126 * Move the cursor to (x, y) 00127 * 00128 * @param x-axis position 00129 * @param y-axis position 00130 * @returns true if there is no error, false otherwise 00131 */ 00132 bool move(int16_t x, int16_t y); 00133 00134 /** 00135 * Press one or several buttons 00136 * 00137 * @param button button state (ex: press(MOUSE_LEFT)) 00138 * @returns true if there is no error, false otherwise 00139 */ 00140 bool press(uint8_t button); 00141 00142 /** 00143 * Release one or several buttons 00144 * 00145 * @param button button state (ex: release(MOUSE_LEFT)) 00146 * @returns true if there is no error, false otherwise 00147 */ 00148 bool release(uint8_t button); 00149 00150 /** 00151 * Double click (MOUSE_LEFT) 00152 * 00153 * @returns true if there is no error, false otherwise 00154 */ 00155 bool doubleClick(); 00156 00157 /** 00158 * Click 00159 * 00160 * @param button state of the buttons ( ex: clic(MOUSE_LEFT)) 00161 * @returns true if there is no error, false otherwise 00162 */ 00163 bool click(uint8_t button); 00164 00165 /** 00166 * Scrolling 00167 * 00168 * @param z value of the wheel (>0 to go down, <0 to go up) 00169 * @returns true if there is no error, false otherwise 00170 */ 00171 bool scroll(int8_t z); 00172 00173 /* 00174 * To define the report descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00175 * 00176 * @returns pointer to the report descriptor 00177 */ 00178 virtual uint8_t * reportDesc(); 00179 00180 private: 00181 MOUSE_TYPE mouse_type; 00182 uint8_t button; 00183 bool mouseSend(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00184 }; 00185 00186 #endif
Generated on Wed Jul 13 2022 01:14:17 by
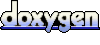