
EthernetInterface, TCP Echo Server, support multi session
Dependencies: EthernetInterface mbed-rtos mbed
Fork of TCPEchoServer by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "EthernetInterface.h" 00004 00005 #define ECHO_SERVER_PORT 10000 00006 00007 #define THREAD_MAX 5 00008 Thread *threads[THREAD_MAX]; 00009 Thread *xthread; 00010 00011 void child (void const *arg) { 00012 int i; 00013 char buf[256]; 00014 TCPSocketConnection *client = (TCPSocketConnection*)arg; 00015 00016 for (;;) { 00017 printf("Connection from %s\r\n", client->get_address()); 00018 // client->set_blocking(false, 1500); // Timeout after (1.5)s 00019 for (;;) { 00020 i = client->receive(buf, sizeof(buf)); 00021 if (i < 0) break; 00022 if (i == 0) continue; 00023 buf[i] = 0; 00024 printf("Recv '%s'\r\n", buf); 00025 if (client->send(buf, i) < 0) break; 00026 } 00027 00028 client->close(); 00029 printf("Close %s\r\n", client->get_address()); 00030 00031 Thread::signal_wait(1); 00032 } 00033 } 00034 00035 void closer (void const *arg) { 00036 TCPSocketConnection *client = (TCPSocketConnection*)arg; 00037 00038 for (;;) { 00039 client->close(); 00040 printf("Close %s\r\n", client->get_address()); 00041 00042 Thread::signal_wait(1); 00043 } 00044 } 00045 00046 void parent () { 00047 int i, t = 0; 00048 TCPSocketConnection clients[THREAD_MAX]; 00049 TCPSocketConnection xclient; 00050 00051 for (i = 0; i < THREAD_MAX; i ++) { 00052 threads[i] = NULL; 00053 } 00054 xthread = NULL; 00055 00056 TCPSocketServer server; 00057 server.bind(ECHO_SERVER_PORT); 00058 server.listen(); 00059 00060 printf("Wait for new connection...\r\n"); 00061 for (;;) { 00062 if (t >= 0) { 00063 server.accept(clients[t]); 00064 // fork child process 00065 if (threads[t]) { 00066 threads[t]->signal_set(1); 00067 } else { 00068 threads[t] = new Thread(child, (void*)&clients[t]); 00069 } 00070 printf("Forked %d\r\n", t); 00071 } else { 00072 server.accept(xclient); 00073 // closer process 00074 if (xthread) { 00075 xthread->signal_set(1); 00076 } else { 00077 xthread = new Thread(closer, (void*)&xclient); 00078 } 00079 printf("Connection full\r\n"); 00080 } 00081 00082 t = -1; 00083 for (i = 0; i < THREAD_MAX; i ++) { 00084 /* 00085 if (threads[i]) { 00086 if (threads[i]->get_state() == Thread::Inactive) { 00087 // terminate process 00088 // delete threads[i]; 00089 // threads[i] = NULL; 00090 printf("Terminated %d\r\n", i); 00091 } 00092 } 00093 */ 00094 if (threads[i] == NULL || threads[i]->get_state() == Thread::WaitingAnd) { 00095 if (t < 0) t = i; // next empty thread 00096 } 00097 } 00098 } 00099 } 00100 00101 00102 int main () { 00103 EthernetInterface eth; 00104 printf("TCP Echo Server with Fork...\r\n"); 00105 eth.init(); //Use DHCP 00106 if (eth.connect()) return -1; 00107 printf("IP Address is %s\r\n", eth.getIPAddress()); 00108 00109 parent(); 00110 }
Generated on Sun Jul 24 2022 11:40:39 by
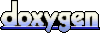