
http://mbed.org/users/okini3939/notebook/RPC_jp/
Embed:
(wiki syntax)
Show/hide line numbers
MyRPC.cpp
00001 /* 00002 * sample from http://mbed.org/forum/mbed/topic/234/?page=1#comment-1067 00003 */ 00004 00005 #include "MyRPC.h" 00006 #ifdef MBED_RPC 00007 #include "rpc.h" 00008 #endif 00009 00010 namespace mbed { 00011 00012 MyRPC::MyRPC(PinName pin, const char *name) : Base(name), _pin(pin) {} 00013 00014 void MyRPC::blink(int n) { 00015 for (int i=0; i<n; i++) { 00016 _pin = 1; 00017 wait(0.2); 00018 _pin = 0; 00019 wait(0.2); 00020 } 00021 } 00022 00023 int MyRPC::number() { 00024 return rand(); 00025 } 00026 00027 #ifdef MBED_RPC 00028 const rpc_method *MyRPC::get_rpc_methods() { 00029 static const rpc_method rpc_methods[] = { 00030 { "blink", rpc_method_caller<MyRPC, int, &MyRPC::blink>}, 00031 { "number", rpc_method_caller<int, MyRPC, &MyRPC::number> }, 00032 RPC_METHOD_SUPER(Base) 00033 }; 00034 return rpc_methods; 00035 } 00036 00037 rpc_class *MyRPC::get_rpc_class() { 00038 static const rpc_function funcs[] = { 00039 "new", rpc_function_caller<const char*, PinName, const char*, &Base::construct<MyRPC,PinName,const char*> >, 00040 RPC_METHOD_END 00041 }; 00042 static rpc_class c = { "MyRPC", funcs, NULL }; 00043 return &c; 00044 } 00045 #endif 00046 00047 } // namespace mbed 00048
Generated on Fri Jul 15 2022 07:42:47 by
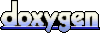