Embed:
(wiki syntax)
Show/hide line numbers
ILinterpreter.h
Go to the documentation of this file.
00001 /* 00002 * Instruction List interpreter library 00003 * Copyright (c) 2011 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 /** @file 00008 * @brief Instruction List interpreter 00009 */ 00010 00011 #ifndef ILinterpreter_H 00012 #define ILinterpreter_H 00013 00014 #include "mbed.h" 00015 00016 #define IL_NUM 100 00017 #define IL_RELAY_NUM 10 00018 #define IL_TIMER_NUM 10 00019 #define IL_COUNTER_NUM 10 00020 #define IL_STACK 10 00021 00022 enum eMNEMONIC { 00023 MNE_NULL, 00024 MNE_DEF, 00025 MNE_LD, MNE_LDI, MNE_LDP, MNE_LDF, 00026 MNE_ALD, MNE_ALDI, MNE_ALDP, MNE_ALDF, 00027 MNE_OR, MNE_ORI, MNE_ORP, MNE_ORF, 00028 MNE_AND, MNE_ANI, MNE_ANDP, MNE_ANDF, 00029 MNE_ORB, MNE_ANB, 00030 MNE_INV, 00031 MNE_MPS, MNE_MRD, MNE_MPP, 00032 MNE_OUT, MNE_SET, MNE_RST, 00033 MNE_END, 00034 }; 00035 00036 enum eEXPRESSION { 00037 EXP_NULL, 00038 EXP_EQ, EXP_NE, 00039 EXP_LE, EXP_LT, 00040 EXP_GE, EXP_GT, 00041 EXP_MOD, EXP_NMOD, 00042 }; 00043 00044 struct tIL { 00045 enum eMNEMONIC mnemonic; 00046 char key; 00047 char keynum; 00048 enum eEXPRESSION expression; 00049 float value; 00050 }; 00051 00052 struct tInOut { 00053 time_t sec; 00054 int relay[IL_RELAY_NUM]; 00055 int timer_flg[IL_TIMER_NUM]; 00056 unsigned int timer_set[IL_TIMER_NUM], timer_cnt[IL_TIMER_NUM]; 00057 unsigned int count_set[IL_COUNTER_NUM], count_cnt[IL_COUNTER_NUM], count_rev[IL_COUNTER_NUM]; 00058 }; 00059 00060 00061 /** ILinterpreter class 00062 */ 00063 class ILinterpreter { 00064 public: 00065 ILinterpreter (); 00066 00067 /** exec IL sequence 00068 * @retval 0 success 00069 * @retval -1 error 00070 */ 00071 int exec (); 00072 00073 /** set call back function 00074 * @param pf_i input function (input relay) 00075 * @param pf_o output function (output relay) 00076 * @return pointer of tInOut (internal relay) 00077 */ 00078 struct tInOut* attach (float (*pf_i)(char, int, eEXPRESSION, int), void (*pf_o)(char, int, int, eMNEMONIC)); 00079 00080 /** timer interval (call 10Hz) 00081 */ 00082 void pool (); 00083 00084 /** load IL file 00085 * @param file file name 00086 * @retval 0 success 00087 * @retval -1 error 00088 */ 00089 int load (char *file); 00090 00091 protected: 00092 int il_count; 00093 struct tIL il[IL_NUM]; 00094 struct tInOut inout, inout_old; 00095 int stack[IL_STACK]; 00096 int addr; 00097 00098 int input (tInOut *io, int i, int old = 0); 00099 void output (int i, int reg, eMNEMONIC mne); 00100 void load_exp (int i, char *buf); 00101 int push (int dat); 00102 int pop (int *dat); 00103 int read (int *dat); 00104 00105 float (*cb_input)(char key, int keynum, eEXPRESSION exp, int old); 00106 void (*cb_output)(char key, int keynum, int reg, eMNEMONIC mne); 00107 00108 private: 00109 00110 }; 00111 00112 #endif
Generated on Thu Jul 21 2022 02:05:17 by
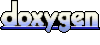