SNMP Agent http://mbed.org/users/okini3939/notebook/agentbed-library/
Dependents: WeatherPlatform_20110408 WeatherPlatform WeatherStation
Agentbed.h
00001 /* 00002 Agentbed library 00003 Modified for mbed, 2010 Suga. 00004 00005 00006 Agentuino.cpp - An Arduino library for a lightweight SNMP Agent. 00007 Copyright (C) 2010 Eric C. Gionet <lavco_eg@hotmail.com> 00008 All rights reserved. 00009 00010 This library is free software; you can redistribute it and/or 00011 modify it under the terms of the GNU Lesser General Public 00012 License as published by the Free Software Foundation; either 00013 version 2.1 of the License, or (at your option) any later version. 00014 00015 This library is distributed in the hope that it will be useful, 00016 but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00018 Lesser General Public License for more details. 00019 00020 You should have received a copy of the GNU Lesser General Public 00021 License along with this library; if not, write to the Free Software 00022 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00023 */ 00024 00025 #ifndef Agentbed_h 00026 #define Agentbed_h 00027 00028 #define SNMP_DEFAULT_PORT 161 00029 #define SNMP_MIN_OID_LEN 2 00030 #define SNMP_MAX_OID_LEN 64 // 128 00031 #define SNMP_MAX_NAME_LEN 20 00032 #define SNMP_MAX_VALUE_LEN 64 // 128 ??? should limit this 00033 #define SNMP_MAX_PACKET_LEN SNMP_MAX_VALUE_LEN + SNMP_MAX_OID_LEN + 25 //??? 00034 #define SNMP_FREE(s) do { if (s) { free((void *)s); s=NULL; } } while(0) 00035 //Frees a pointer only if it is !NULL and sets its value to NULL. 00036 00037 #include "mbed.h" 00038 #include "EthernetNetIf.h" 00039 #include "UDPSocket.h" 00040 #include <stdlib.h> 00041 00042 extern "C" { 00043 // callback function 00044 typedef void (*onPduReceiveCallback)(void); 00045 } 00046 00047 //typedef long long int64_t; 00048 typedef unsigned long long uint64_t; 00049 //typedef long int32_t; 00050 //typedef unsigned long uint32_t; 00051 //typedef unsigned char uint8_t; 00052 //typedef short int16_t; 00053 //typedef unsigned short uint16_t; 00054 typedef unsigned char byte; 00055 00056 typedef union uint64_u { 00057 uint64_t uint64; 00058 byte data[8]; 00059 } uint64_u; 00060 00061 typedef union int32_u { 00062 int32_t int32; 00063 byte data[4]; 00064 } int32_u; 00065 00066 typedef union uint32_u{ 00067 uint32_t uint32; 00068 byte data[4]; 00069 } uint32_u; 00070 00071 typedef union int16_u { 00072 int16_t int16; 00073 byte data[2]; 00074 } int16_u; 00075 00076 //typedef union uint16_u { 00077 // uint16_t uint16; 00078 // byte data[2]; 00079 //}; 00080 00081 typedef union float_u{ 00082 float float_t; 00083 byte data[4]; 00084 } float_u; 00085 00086 enum ASN_BER_BASE_TYPES { 00087 // ASN/BER base types 00088 ASN_BER_BASE_UNIVERSAL = 0x0, 00089 ASN_BER_BASE_APPLICATION = 0x40, 00090 ASN_BER_BASE_CONTEXT = 0x80, 00091 ASN_BER_BASE_PUBLIC = 0xC0, 00092 ASN_BER_BASE_PRIMITIVE = 0x0, 00093 ASN_BER_BASE_CONSTRUCTOR = 0x20 00094 }; 00095 00096 enum SNMP_PDU_TYPES { 00097 // PDU choices 00098 SNMP_PDU_GET = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 0, 00099 SNMP_PDU_GET_NEXT = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 1, 00100 SNMP_PDU_RESPONSE = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 2, 00101 SNMP_PDU_SET = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 3, 00102 SNMP_PDU_TRAP = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 4 00103 }; 00104 00105 enum SNMP_TRAP_TYPES { 00106 // Trap generic types: 00107 SNMP_TRAP_COLD_START = 0, 00108 SNMP_TRAP_WARM_START = 1, 00109 SNMP_TRAP_LINK_DOWN = 2, 00110 SNMP_TRAP_LINK_UP = 3, 00111 SNMP_TRAP_AUTHENTICATION_FAIL = 4, 00112 SNMP_TRAP_EGP_NEIGHBORLOSS = 5, 00113 SNMP_TRAP_ENTERPRISE_SPECIFIC = 6 00114 }; 00115 00116 enum SNMP_ERR_CODES { 00117 SNMP_ERR_NO_ERROR = 0, 00118 SNMP_ERR_TOO_BIG = 1, 00119 SNMP_ERR_NO_SUCH_NAME = 2, 00120 SNMP_ERR_BAD_VALUE = 3, 00121 SNMP_ERR_READ_ONLY = 4, 00122 SNMP_ERR_GEN_ERROR = 5, 00123 00124 SNMP_ERR_NO_ACCESS = 6, 00125 SNMP_ERR_WRONG_TYPE = 7, 00126 SNMP_ERR_WRONG_LENGTH = 8, 00127 SNMP_ERR_WRONG_ENCODING = 9, 00128 SNMP_ERR_WRONG_VALUE = 10, 00129 SNMP_ERR_NO_CREATION = 11, 00130 SNMP_ERR_INCONSISTANT_VALUE = 12, 00131 SNMP_ERR_RESOURCE_UNAVAILABLE = 13, 00132 SNMP_ERR_COMMIT_FAILED = 14, 00133 SNMP_ERR_UNDO_FAILED = 15, 00134 SNMP_ERR_AUTHORIZATION_ERROR = 16, 00135 SNMP_ERR_NOT_WRITABLE = 17, 00136 SNMP_ERR_INCONSISTEN_NAME = 18 00137 }; 00138 00139 enum SNMP_API_STAT_CODES { 00140 SNMP_API_STAT_SUCCESS = 0, 00141 SNMP_API_STAT_MALLOC_ERR = 1, 00142 SNMP_API_STAT_NAME_TOO_BIG = 2, 00143 SNMP_API_STAT_OID_TOO_BIG = 3, 00144 SNMP_API_STAT_VALUE_TOO_BIG = 4, 00145 SNMP_API_STAT_PACKET_INVALID = 5, 00146 SNMP_API_STAT_PACKET_TOO_BIG = 6 00147 }; 00148 00149 // 00150 // http://oreilly.com/catalog/esnmp/chapter/ch02.html Table 2-1: SMIv1 Datatypes 00151 00152 enum SNMP_SYNTAXES { 00153 // SNMP ObjectSyntax values 00154 SNMP_SYNTAX_SEQUENCE = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_CONSTRUCTOR | 0x10, 00155 // These values are used in the "syntax" member of VALUEs 00156 SNMP_SYNTAX_BOOL = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 1, 00157 SNMP_SYNTAX_INT = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 2, 00158 SNMP_SYNTAX_BITS = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 3, 00159 SNMP_SYNTAX_OCTETS = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 4, 00160 SNMP_SYNTAX_NULL = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 5, 00161 SNMP_SYNTAX_OID = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 6, 00162 SNMP_SYNTAX_INT32 = SNMP_SYNTAX_INT, 00163 SNMP_SYNTAX_IP_ADDRESS = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 0, 00164 SNMP_SYNTAX_COUNTER = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 1, 00165 SNMP_SYNTAX_GAUGE = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 2, 00166 SNMP_SYNTAX_TIME_TICKS = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 3, 00167 SNMP_SYNTAX_OPAQUE = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 4, 00168 SNMP_SYNTAX_NSAPADDR = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 5, 00169 SNMP_SYNTAX_COUNTER64 = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 6, 00170 SNMP_SYNTAX_UINT32 = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 7, 00171 SNMP_SYNTAX_OPAQUE_FLOAT = 0x78, 00172 }; 00173 00174 typedef struct SNMP_OID { 00175 byte data[SNMP_MAX_OID_LEN]; // ushort array insted?? 00176 size_t size; 00177 // 00178 void fromString(const char *buffer) { 00179 } 00180 // 00181 void toString(char *buffer) { 00182 buffer[0] = '1'; 00183 buffer[1] = '.'; 00184 buffer[2] = '3'; 00185 buffer[3] = '\0'; 00186 // 00187 // tmp buffer - short (Int16) 00188 //char *buff = (char *)malloc(sizeof(char)*16); // I ya ya keeps hanging the MCU 00189 char buff[16]; 00190 byte hsize = size - 1; 00191 byte hpos = 1; 00192 uint16_t subid; 00193 // 00194 while ( hsize > 0 ) { 00195 subid = 0; 00196 uint16_t b = 0; 00197 do { 00198 uint16_t next = data[hpos++]; 00199 b = next & 0xFF; 00200 subid = (subid << 7) + (b & ~0x80); 00201 hsize--; 00202 } while ( (hsize > 0) && ((b & 0x80) != 0) ); 00203 //utoa(subid, buff, 10); 00204 sprintf(buff, "%d", subid); 00205 strcat(buffer, "."); 00206 strcat(buffer, buff); 00207 } 00208 // 00209 // free buff 00210 //SNMP_FREE(buff); 00211 }; 00212 } SNMP_OID; 00213 00214 // union for values? 00215 // 00216 typedef struct SNMP_VALUE { 00217 byte data[SNMP_MAX_VALUE_LEN]; 00218 size_t size; 00219 SNMP_SYNTAXES syntax; 00220 // 00221 byte i; // for encoding/decoding functions 00222 // 00223 // clear's buffer and sets size to 0 00224 void clear(void) { 00225 memset(data, 0, SNMP_MAX_VALUE_LEN); 00226 size = 0; 00227 } 00228 // 00229 // 00230 // ASN.1 decoding functions 00231 // 00232 // decode's an octet string, object-identifier, opaque syntax to string 00233 SNMP_ERR_CODES decode(char *value, size_t max_size) { 00234 if ( syntax == SNMP_SYNTAX_OCTETS || syntax == SNMP_SYNTAX_OID 00235 || syntax == SNMP_SYNTAX_OPAQUE ) { 00236 if ( strlen(value) - 1 < max_size ) { 00237 if ( syntax == SNMP_SYNTAX_OID ) { 00238 value[0] = '1'; 00239 value[1] = '.'; 00240 value[2] = '3'; 00241 value[3] = '\0'; 00242 // 00243 // tmp buffer - ushort (UInt16) 00244 //char *buff = (char *)malloc(sizeof(char)*16); // I ya ya..keep hanging the MCU 00245 char buff[16]; 00246 byte hsize = size - 1; 00247 byte hpos = 1; 00248 uint16_t subid; 00249 // 00250 while ( hsize > 0 ) { 00251 subid = 0; 00252 uint16_t b = 0; 00253 do { 00254 uint16_t next = data[hpos++]; 00255 b = next & 0xFF; 00256 subid = (subid << 7) + (b & ~0x80); 00257 hsize--; 00258 } while ( (hsize > 0) && ((b & 0x80) != 0) ); 00259 //utoa(subid, buff, 10); 00260 sprintf(buff, "%d", subid); 00261 strcat(value, "."); 00262 strcat(value, buff); 00263 } 00264 // 00265 // free buff 00266 //SNMP_FREE(buff); 00267 } else { 00268 for ( i = 0; i < size; i++ ) { 00269 value[i] = (char)data[i]; 00270 } 00271 value[size] = '\0'; 00272 } 00273 return SNMP_ERR_NO_ERROR; 00274 } else { 00275 clear(); 00276 return SNMP_ERR_TOO_BIG; 00277 } 00278 } else { 00279 clear(); 00280 return SNMP_ERR_WRONG_TYPE; 00281 } 00282 } 00283 // 00284 // decode's an int syntax to int16 ?? is this applicable 00285 SNMP_ERR_CODES decode(int16_t *value) { 00286 if ( syntax == SNMP_SYNTAX_INT ) { 00287 int16_u tmp; 00288 tmp.data[1] = data[0]; 00289 tmp.data[0] = data[1]; 00290 *value = tmp.int16; 00291 return SNMP_ERR_NO_ERROR; 00292 } else { 00293 clear(); 00294 return SNMP_ERR_WRONG_TYPE; 00295 } 00296 } 00297 // 00298 // decode's an int32 syntax to int32 00299 SNMP_ERR_CODES decode(int32_t *value) { 00300 if ( syntax == SNMP_SYNTAX_INT32 ) { 00301 int32_u tmp; 00302 tmp.data[3] = data[0]; 00303 tmp.data[2] = data[1]; 00304 tmp.data[1] = data[2]; 00305 tmp.data[0] = data[3]; 00306 *value = tmp.int32; 00307 return SNMP_ERR_NO_ERROR; 00308 } else { 00309 clear(); 00310 return SNMP_ERR_WRONG_TYPE; 00311 } 00312 } 00313 // 00314 // decode's an uint32, counter, time-ticks, gauge syntax to uint32 00315 SNMP_ERR_CODES decode(uint32_t *value) { 00316 if ( syntax == SNMP_SYNTAX_COUNTER || syntax == SNMP_SYNTAX_TIME_TICKS 00317 || syntax == SNMP_SYNTAX_GAUGE || syntax == SNMP_SYNTAX_UINT32 ) { 00318 uint32_u tmp; 00319 tmp.data[3] = data[0]; 00320 tmp.data[2] = data[1]; 00321 tmp.data[1] = data[2]; 00322 tmp.data[0] = data[3]; 00323 *value = tmp.uint32; 00324 return SNMP_ERR_NO_ERROR; 00325 } else { 00326 clear(); 00327 return SNMP_ERR_WRONG_TYPE; 00328 } 00329 } 00330 // 00331 // decode's an ip-address, NSAP-address syntax to an ip-address byte array 00332 SNMP_ERR_CODES decode(byte *value) { 00333 memset(data, 0, SNMP_MAX_VALUE_LEN); 00334 if ( syntax == SNMP_SYNTAX_IP_ADDRESS || syntax == SNMP_SYNTAX_NSAPADDR ) { 00335 if ( sizeof(value) > 4 ) { 00336 clear(); 00337 return SNMP_ERR_TOO_BIG; 00338 } else { 00339 size = 4; 00340 data[0] = value[3]; 00341 data[1] = value[2]; 00342 data[2] = value[1]; 00343 data[3] = value[0]; 00344 return SNMP_ERR_NO_ERROR; 00345 } 00346 } else { 00347 clear(); 00348 return SNMP_ERR_WRONG_TYPE; 00349 } 00350 } 00351 // 00352 // decode's a boolean syntax to boolean 00353 SNMP_ERR_CODES decode(bool *value) { 00354 if ( syntax == SNMP_SYNTAX_BOOL ) { 00355 *value = (data[0] != 0); 00356 return SNMP_ERR_NO_ERROR; 00357 } else { 00358 clear(); 00359 return SNMP_ERR_WRONG_TYPE; 00360 } 00361 } 00362 // 00363 // 00364 // ASN.1 encoding functions 00365 // 00366 // encode's a octet string to a string, opaque syntax 00367 // encode object-identifier here?? 00368 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const char *value) { 00369 memset(data, 0, SNMP_MAX_VALUE_LEN); 00370 if ( syn == SNMP_SYNTAX_OCTETS || syn == SNMP_SYNTAX_OPAQUE ) { 00371 if ( strlen(value) - 1 < SNMP_MAX_VALUE_LEN ) { 00372 syntax = syn; 00373 size = strlen(value); 00374 for ( i = 0; i < size; i++ ) { 00375 data[i] = (byte)value[i]; 00376 } 00377 return SNMP_ERR_NO_ERROR; 00378 } else { 00379 clear(); 00380 return SNMP_ERR_TOO_BIG; 00381 } 00382 } else { 00383 clear(); 00384 return SNMP_ERR_WRONG_TYPE; 00385 } 00386 } 00387 // 00388 // encode's an int16 to int, opaque syntax 00389 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const int16_t value) { 00390 memset(data, 0, SNMP_MAX_VALUE_LEN); 00391 if ( syn == SNMP_SYNTAX_INT || syn == SNMP_SYNTAX_OPAQUE ) { 00392 int16_u tmp; 00393 size = 2; 00394 syntax = syn; 00395 tmp.int16 = value; 00396 data[0] = tmp.data[1]; 00397 data[1] = tmp.data[0]; 00398 return SNMP_ERR_NO_ERROR; 00399 } else { 00400 clear(); 00401 return SNMP_ERR_WRONG_TYPE; 00402 } 00403 } 00404 // 00405 // encode's an int32 to int32, opaque syntax 00406 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const int32_t value) { 00407 memset(data, 0, SNMP_MAX_VALUE_LEN); 00408 if ( syn == SNMP_SYNTAX_INT32 || syn == SNMP_SYNTAX_OPAQUE ) { 00409 int32_u tmp; 00410 size = 4; 00411 syntax = syn; 00412 tmp.int32 = value; 00413 data[0] = tmp.data[3]; 00414 data[1] = tmp.data[2]; 00415 data[2] = tmp.data[1]; 00416 data[3] = tmp.data[0]; 00417 return SNMP_ERR_NO_ERROR; 00418 } else { 00419 clear(); 00420 return SNMP_ERR_WRONG_TYPE; 00421 } 00422 } 00423 // 00424 // encode's an uint32 to uint32, counter, time-ticks, gauge, opaque syntax 00425 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const uint32_t value) { 00426 memset(data, 0, SNMP_MAX_VALUE_LEN); 00427 if ( syn == SNMP_SYNTAX_COUNTER || syn == SNMP_SYNTAX_TIME_TICKS 00428 || syn == SNMP_SYNTAX_GAUGE || syn == SNMP_SYNTAX_UINT32 00429 || syn == SNMP_SYNTAX_OPAQUE ) { 00430 uint32_u tmp; 00431 size = 4; 00432 syntax = syn; 00433 tmp.uint32 = value; 00434 data[0] = tmp.data[3]; 00435 data[1] = tmp.data[2]; 00436 data[2] = tmp.data[1]; 00437 data[3] = tmp.data[0]; 00438 return SNMP_ERR_NO_ERROR; 00439 } else { 00440 clear(); 00441 return SNMP_ERR_WRONG_TYPE; 00442 } 00443 } 00444 // 00445 // encode's an ip-address byte array to ip-address, NSAP-address, opaque syntax 00446 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const byte *value) { 00447 memset(data, 0, SNMP_MAX_VALUE_LEN); 00448 if ( syn == SNMP_SYNTAX_IP_ADDRESS || syn == SNMP_SYNTAX_NSAPADDR 00449 || syn == SNMP_SYNTAX_OPAQUE ) { 00450 if ( sizeof(value) > 4 ) { 00451 clear(); 00452 return SNMP_ERR_TOO_BIG; 00453 } else { 00454 size = 4; 00455 syntax = syn; 00456 data[0] = value[3]; 00457 data[1] = value[2]; 00458 data[2] = value[1]; 00459 data[3] = value[0]; 00460 return SNMP_ERR_NO_ERROR; 00461 } 00462 } else { 00463 clear(); 00464 return SNMP_ERR_WRONG_TYPE; 00465 } 00466 } 00467 // 00468 // encode's a boolean to boolean, opaque syntax 00469 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const bool value) { 00470 memset(data, 0, SNMP_MAX_VALUE_LEN); 00471 if ( syn == SNMP_SYNTAX_BOOL || syn == SNMP_SYNTAX_OPAQUE ) { 00472 size = 1; 00473 syntax = syn; 00474 data[0] = value ? 0xff : 0; 00475 return SNMP_ERR_NO_ERROR; 00476 } else { 00477 clear(); 00478 return SNMP_ERR_WRONG_TYPE; 00479 } 00480 } 00481 // 00482 // encode's an uint64 to counter64, opaque syntax 00483 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const uint64_t value) { 00484 memset(data, 0, SNMP_MAX_VALUE_LEN); 00485 if ( syn == SNMP_SYNTAX_COUNTER64 || syn == SNMP_SYNTAX_OPAQUE ) { 00486 uint64_u tmp; 00487 size = 8; 00488 syntax = syn; 00489 tmp.uint64 = value; 00490 data[0] = tmp.data[7]; 00491 data[1] = tmp.data[6]; 00492 data[2] = tmp.data[5]; 00493 data[3] = tmp.data[4]; 00494 data[4] = tmp.data[3]; 00495 data[5] = tmp.data[2]; 00496 data[6] = tmp.data[1]; 00497 data[7] = tmp.data[0]; 00498 return SNMP_ERR_NO_ERROR; 00499 } else { 00500 clear(); 00501 return SNMP_ERR_WRONG_TYPE; 00502 } 00503 } 00504 // 00505 // encode's an int32 to int32, opaque syntax 00506 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const float value) { 00507 memset(data, 0, SNMP_MAX_VALUE_LEN); 00508 if ( syn == SNMP_SYNTAX_OPAQUE_FLOAT ) { 00509 float_u tmp; 00510 size = 4; 00511 syntax = syn; 00512 tmp.float_t = value; 00513 data[0] = tmp.data[3]; 00514 data[1] = tmp.data[2]; 00515 data[2] = tmp.data[1]; 00516 data[3] = tmp.data[0]; 00517 return SNMP_ERR_NO_ERROR; 00518 } else { 00519 clear(); 00520 return SNMP_ERR_WRONG_TYPE; 00521 } 00522 } 00523 // 00524 // encode's a null to null, opaque syntax 00525 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn) { 00526 clear(); 00527 if ( syn == SNMP_SYNTAX_NULL || syn == SNMP_SYNTAX_OPAQUE ) { 00528 size = 0; 00529 syntax = syn; 00530 return SNMP_ERR_NO_ERROR; 00531 } else { 00532 return SNMP_ERR_WRONG_TYPE; 00533 } 00534 } 00535 } SNMP_VALUE; 00536 00537 typedef struct SNMP_PDU { 00538 SNMP_PDU_TYPES type; 00539 int32_t version; 00540 int32_t requestId; 00541 SNMP_ERR_CODES error; 00542 int32_t errorIndex; 00543 SNMP_OID OID; 00544 SNMP_VALUE VALUE; 00545 } SNMP_PDU; 00546 00547 class AgentbedClass { 00548 public: 00549 // Agent functions 00550 SNMP_API_STAT_CODES begin(EthernetNetIf *eth); 00551 SNMP_API_STAT_CODES begin(char *getCommName, char *setCommName, uint16_t port, EthernetNetIf *eth); 00552 void listen(UDPSocketEvent); 00553 SNMP_API_STAT_CODES requestPdu(SNMP_PDU *pdu); 00554 SNMP_API_STAT_CODES responsePdu(SNMP_PDU *pdu); 00555 void onPduReceive(onPduReceiveCallback pduReceived); 00556 void freePdu(SNMP_PDU *pdu); 00557 00558 // Helper functions 00559 00560 private: 00561 byte _packet[SNMP_MAX_PACKET_LEN]; 00562 uint16_t _packetSize; 00563 uint16_t _packetPos; 00564 SNMP_PDU_TYPES _dstType; 00565 char *_getCommName; 00566 size_t _getSize; 00567 char *_setCommName; 00568 size_t _setSize; 00569 onPduReceiveCallback _callback; 00570 UDPSocket *udpsock; 00571 Host _dst; 00572 }; 00573 00574 //extern AgentbedClass Agentbed; 00575 00576 #endif
Generated on Wed Jul 13 2022 23:20:05 by
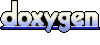