
TinyJS on mbed. TinyJS is very simple JavaScript engine.
CScriptVar Class Reference
Variable class (containing a doubly-linked list of children) More...
#include <TinyJS.h>
Public Member Functions | |
CScriptVar () | |
Create undefined. | |
CScriptVar (const std::string &varData, int varFlags) | |
User defined. | |
CScriptVar (const std::string &str) | |
Create a string. | |
CScriptVar * | getReturnVar () |
If this is a function, get the result value (for use by native functions) | |
void | setReturnVar (CScriptVar *var) |
Set the result value. Use this when setting complex return data as it avoids a deepCopy() | |
CScriptVar * | getParameter (const std::string &name) |
If this is a function, get the parameter with the given name (for use by native functions) | |
CScriptVarLink * | findChild (const std::string &childName) |
Tries to find a child with the given name, may return 0. | |
CScriptVarLink * | findChildOrCreate (const std::string &childName, int varFlags=SCRIPTVAR_UNDEFINED) |
Tries to find a child with the given name, or will create it with the given flags. | |
CScriptVarLink * | findChildOrCreateByPath (const std::string &path) |
Tries to find a child with the given path (separated by dots) | |
CScriptVarLink * | addChildNoDup (const std::string &childName, CScriptVar *child=NULL) |
add a child overwriting any with the same name | |
void | removeLink (CScriptVarLink *link) |
Remove a specific link (this is faster than finding via a child) | |
CScriptVar * | getArrayIndex (int idx) |
The the value at an array index. | |
void | setArrayIndex (int idx, CScriptVar *value) |
Set the value at an array index. | |
int | getArrayLength () |
If this is an array, return the number of items in it (else 0) | |
int | getChildren () |
Get the number of children. | |
std::string | getParsableString () |
get Data as a parsable javascript string | |
bool | isBasic () |
Is this *not* an array/object/etc. | |
CScriptVar * | mathsOp (CScriptVar *b, int op) |
do a maths op with another script variable | |
void | copyValue (CScriptVar *val) |
copy the value from the value given | |
CScriptVar * | deepCopy () |
deep copy this node and return the result | |
void | trace (std::string indentStr="", const std::string &name="") |
Dump out the contents of this using trace. | |
std::string | getFlagsAsString () |
For debugging - just dump a string version of the flags. | |
void | getJSON (std::ostringstream &destination, const std::string linePrefix="") |
Write out all the JS code needed to recreate this script variable to the stream (as JSON) | |
void | getJSON (std::string &destination, const std::string linePrefix="") |
Write out all the JS code needed to recreate this script variable to the stream (as JSON) | |
void | setCallback (JSCallback callback, void *userdata) |
Set the callback for native functions. | |
CScriptVar * | ref () |
For memory management/garbage collection. | |
void | unref () |
Remove a reference, and delete this variable if required. | |
int | getRefs () |
Get the number of references to this script variable. | |
Protected Member Functions | |
void | init () |
initialisation of data members | |
void | copySimpleData (CScriptVar *val) |
Copy the basic data and flags from the variable given, with no children. | |
Protected Attributes | |
int | refs |
The number of references held to this - used for garbage collection. | |
std::string | data |
The contents of this variable if it is a string. | |
long | intData |
The contents of this variable if it is an int. | |
double | doubleData |
The contents of this variable if it is a double. | |
int | flags |
the flags determine the type of the variable - int/double/string/etc | |
JSCallback | jsCallback |
Callback for native functions. | |
void * | jsCallbackUserData |
user data passed as second argument to native functions |
Detailed Description
Variable class (containing a doubly-linked list of children)
Definition at line 210 of file TinyJS.h.
Constructor & Destructor Documentation
CScriptVar | ( | ) |
Create undefined.
Definition at line 754 of file TinyJS.cpp.
CScriptVar | ( | const std::string & | varData, |
int | varFlags | ||
) |
User defined.
CScriptVar | ( | const std::string & | str ) |
Create a string.
Member Function Documentation
CScriptVarLink * addChildNoDup | ( | const std::string & | childName, |
CScriptVar * | child = NULL |
||
) |
add a child overwriting any with the same name
Definition at line 885 of file TinyJS.cpp.
void copySimpleData | ( | CScriptVar * | val ) | [protected] |
Copy the basic data and flags from the variable given, with no children.
Should be used internally only - by copyValue and deepCopy
Definition at line 1214 of file TinyJS.cpp.
void copyValue | ( | CScriptVar * | val ) |
copy the value from the value given
Definition at line 1221 of file TinyJS.cpp.
CScriptVar * deepCopy | ( | ) |
deep copy this node and return the result
Definition at line 1245 of file TinyJS.cpp.
CScriptVarLink* findChild | ( | const std::string & | childName ) |
Tries to find a child with the given name, may return 0.
CScriptVarLink* findChildOrCreate | ( | const std::string & | childName, |
int | varFlags = SCRIPTVAR_UNDEFINED |
||
) |
Tries to find a child with the given name, or will create it with the given flags.
CScriptVarLink * findChildOrCreateByPath | ( | const std::string & | path ) |
Tries to find a child with the given path (separated by dots)
Definition at line 855 of file TinyJS.cpp.
CScriptVar * getArrayIndex | ( | int | idx ) |
The the value at an array index.
Definition at line 935 of file TinyJS.cpp.
int getArrayLength | ( | ) |
If this is an array, return the number of items in it (else 0)
Definition at line 959 of file TinyJS.cpp.
int getChildren | ( | ) |
Get the number of children.
Definition at line 974 of file TinyJS.cpp.
string getFlagsAsString | ( | ) |
For debugging - just dump a string version of the flags.
Definition at line 1278 of file TinyJS.cpp.
void getJSON | ( | std::ostringstream & | destination, |
const std::string | linePrefix = "" |
||
) |
Write out all the JS code needed to recreate this script variable to the stream (as JSON)
void getJSON | ( | std::string & | destination, |
const std::string | linePrefix = "" |
||
) |
Write out all the JS code needed to recreate this script variable to the stream (as JSON)
CScriptVar * getParameter | ( | const std::string & | name ) |
If this is a function, get the parameter with the given name (for use by native functions)
Definition at line 834 of file TinyJS.cpp.
string getParsableString | ( | ) |
get Data as a parsable javascript string
Definition at line 1290 of file TinyJS.cpp.
int getRefs | ( | ) |
Get the number of references to this script variable.
Definition at line 1426 of file TinyJS.cpp.
CScriptVar * getReturnVar | ( | ) |
If this is a function, get the result value (for use by native functions)
Definition at line 825 of file TinyJS.cpp.
void init | ( | ) | [protected] |
initialisation of data members
Definition at line 814 of file TinyJS.cpp.
CScriptVar * mathsOp | ( | CScriptVar * | b, |
int | op | ||
) |
do a maths op with another script variable
Definition at line 1071 of file TinyJS.cpp.
CScriptVar * ref | ( | ) |
For memory management/garbage collection.
Add reference to this variable
Definition at line 1414 of file TinyJS.cpp.
void removeLink | ( | CScriptVarLink * | link ) |
Remove a specific link (this is faster than finding via a child)
Definition at line 911 of file TinyJS.cpp.
void setArrayIndex | ( | int | idx, |
CScriptVar * | value | ||
) |
Set the value at an array index.
Definition at line 943 of file TinyJS.cpp.
void setCallback | ( | JSCallback | callback, |
void * | userdata | ||
) |
Set the callback for native functions.
Definition at line 1409 of file TinyJS.cpp.
void setReturnVar | ( | CScriptVar * | var ) |
Set the result value. Use this when setting complex return data as it avoids a deepCopy()
Definition at line 829 of file TinyJS.cpp.
void trace | ( | std::string | indentStr = "" , |
const std::string & | name = "" |
||
) |
Dump out the contents of this using trace.
void unref | ( | ) |
Remove a reference, and delete this variable if required.
Definition at line 1419 of file TinyJS.cpp.
Field Documentation
std::string data [protected] |
double doubleData [protected] |
int flags [protected] |
long intData [protected] |
JSCallback jsCallback [protected] |
void* jsCallbackUserData [protected] |
Generated on Tue Jul 12 2022 21:01:11 by
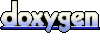