
TinyJS on mbed. TinyJS is very simple JavaScript engine.
Embed:
(wiki syntax)
Show/hide line numbers
TinyJS_MathFunctions.cpp
00001 /* 00002 * TinyJS 00003 * 00004 * A single-file Javascript-alike engine 00005 * 00006 * - Math and Trigonometry functions 00007 * 00008 * Authored By O.Z.L.B. <ozlbinfo@gmail.com> 00009 * 00010 * Copyright (C) 2011 O.Z.L.B. 00011 * 00012 * Permission is hereby granted, free of charge, to any person obtaining a copy of 00013 * this software and associated documentation files (the "Software"), to deal in 00014 * the Software without restriction, including without limitation the rights to 00015 * use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies 00016 * of the Software, and to permit persons to whom the Software is furnished to do 00017 * so, subject to the following conditions: 00018 00019 * The above copyright notice and this permission notice shall be included in all 00020 * copies or substantial portions of the Software. 00021 00022 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00023 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00024 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00025 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00026 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00027 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00028 * SOFTWARE. 00029 */ 00030 00031 #include <math.h> 00032 #include <cstdlib> 00033 #include <sstream> 00034 #include "TinyJS_MathFunctions.h" 00035 00036 using namespace std; 00037 00038 #define k_E exp(1.0) 00039 #define k_PI 3.1415926535897932384626433832795 00040 00041 #define F_ABS(a) ((a)>=0 ? (a) : (-(a))) 00042 #define F_MIN(a,b) ((a)>(b) ? (b) : (a)) 00043 #define F_MAX(a,b) ((a)>(b) ? (a) : (b)) 00044 #define F_SGN(a) ((a)>0 ? 1 : ((a)<0 ? -1 : 0 )) 00045 #define F_RNG(a,min,max) ((a)<(min) ? min : ((a)>(max) ? max : a )) 00046 #define F_ROUND(a) ((a)>0 ? (int) ((a)+0.5) : (int) ((a)-0.5) ) 00047 00048 //CScriptVar shortcut macro 00049 #define scIsInt(a) ( c->getParameter(a)->isInt() ) 00050 #define scIsDouble(a) ( c->getParameter(a)->isDouble() ) 00051 #define scGetInt(a) ( c->getParameter(a)->getInt() ) 00052 #define scGetDouble(a) ( c->getParameter(a)->getDouble() ) 00053 #define scReturnInt(a) ( c->getReturnVar()->setInt(a) ) 00054 #define scReturnDouble(a) ( c->getReturnVar()->setDouble(a) ) 00055 00056 #ifdef _MSC_VER 00057 namespace 00058 { 00059 double asinh( const double &value ) 00060 { 00061 double returned; 00062 00063 if(value>0) 00064 returned = log(value + sqrt(value * value + 1)); 00065 else 00066 returned = -log(-value + sqrt(value * value + 1)); 00067 00068 return(returned); 00069 } 00070 00071 double acosh( const double &value ) 00072 { 00073 double returned; 00074 00075 if(value>0) 00076 returned = log(value + sqrt(value * value - 1)); 00077 else 00078 returned = -log(-value + sqrt(value * value - 1)); 00079 00080 return(returned); 00081 } 00082 } 00083 #endif 00084 00085 //Math.abs(x) - returns absolute of given value 00086 void scMathAbs(CScriptVar *c, void *userdata) { 00087 if ( scIsInt("a") ) { 00088 scReturnInt( F_ABS( scGetInt("a") ) ); 00089 } else if ( scIsDouble("a") ) { 00090 scReturnDouble( F_ABS( scGetDouble("a") ) ); 00091 } 00092 } 00093 00094 //Math.round(a) - returns nearest round of given value 00095 void scMathRound(CScriptVar *c, void *userdata) { 00096 if ( scIsInt("a") ) { 00097 scReturnInt( F_ROUND( scGetInt("a") ) ); 00098 } else if ( scIsDouble("a") ) { 00099 scReturnDouble( F_ROUND( scGetDouble("a") ) ); 00100 } 00101 } 00102 00103 //Math.min(a,b) - returns minimum of two given values 00104 void scMathMin(CScriptVar *c, void *userdata) { 00105 if ( (scIsInt("a")) && (scIsInt("b")) ) { 00106 scReturnInt( F_MIN( scGetInt("a"), scGetInt("b") ) ); 00107 } else { 00108 scReturnDouble( F_MIN( scGetDouble("a"), scGetDouble("b") ) ); 00109 } 00110 } 00111 00112 //Math.max(a,b) - returns maximum of two given values 00113 void scMathMax(CScriptVar *c, void *userdata) { 00114 if ( (scIsInt("a")) && (scIsInt("b")) ) { 00115 scReturnInt( F_MAX( scGetInt("a"), scGetInt("b") ) ); 00116 } else { 00117 scReturnDouble( F_MAX( scGetDouble("a"), scGetDouble("b") ) ); 00118 } 00119 } 00120 00121 //Math.range(x,a,b) - returns value limited between two given values 00122 void scMathRange(CScriptVar *c, void *userdata) { 00123 if ( (scIsInt("x")) ) { 00124 scReturnInt( F_RNG( scGetInt("x"), scGetInt("a"), scGetInt("b") ) ); 00125 } else { 00126 scReturnDouble( F_RNG( scGetDouble("x"), scGetDouble("a"), scGetDouble("b") ) ); 00127 } 00128 } 00129 00130 //Math.sign(a) - returns sign of given value (-1==negative,0=zero,1=positive) 00131 void scMathSign(CScriptVar *c, void *userdata) { 00132 if ( scIsInt("a") ) { 00133 scReturnInt( F_SGN( scGetInt("a") ) ); 00134 } else if ( scIsDouble("a") ) { 00135 scReturnDouble( F_SGN( scGetDouble("a") ) ); 00136 } 00137 } 00138 00139 //Math.PI() - returns PI value 00140 void scMathPI(CScriptVar *c, void *userdata) { 00141 scReturnDouble(k_PI); 00142 } 00143 00144 //Math.toDegrees(a) - returns degree value of a given angle in radians 00145 void scMathToDegrees(CScriptVar *c, void *userdata) { 00146 scReturnDouble( (180.0/k_PI)*( scGetDouble("a") ) ); 00147 } 00148 00149 //Math.toRadians(a) - returns radians value of a given angle in degrees 00150 void scMathToRadians(CScriptVar *c, void *userdata) { 00151 scReturnDouble( (k_PI/180.0)*( scGetDouble("a") ) ); 00152 } 00153 00154 //Math.sin(a) - returns trig. sine of given angle in radians 00155 void scMathSin(CScriptVar *c, void *userdata) { 00156 scReturnDouble( sin( scGetDouble("a") ) ); 00157 } 00158 00159 //Math.asin(a) - returns trig. arcsine of given angle in radians 00160 void scMathASin(CScriptVar *c, void *userdata) { 00161 scReturnDouble( asin( scGetDouble("a") ) ); 00162 } 00163 00164 //Math.cos(a) - returns trig. cosine of given angle in radians 00165 void scMathCos(CScriptVar *c, void *userdata) { 00166 scReturnDouble( cos( scGetDouble("a") ) ); 00167 } 00168 00169 //Math.acos(a) - returns trig. arccosine of given angle in radians 00170 void scMathACos(CScriptVar *c, void *userdata) { 00171 scReturnDouble( acos( scGetDouble("a") ) ); 00172 } 00173 00174 //Math.tan(a) - returns trig. tangent of given angle in radians 00175 void scMathTan(CScriptVar *c, void *userdata) { 00176 scReturnDouble( tan( scGetDouble("a") ) ); 00177 } 00178 00179 //Math.atan(a) - returns trig. arctangent of given angle in radians 00180 void scMathATan(CScriptVar *c, void *userdata) { 00181 scReturnDouble( atan( scGetDouble("a") ) ); 00182 } 00183 00184 //Math.sinh(a) - returns trig. hyperbolic sine of given angle in radians 00185 void scMathSinh(CScriptVar *c, void *userdata) { 00186 scReturnDouble( sinh( scGetDouble("a") ) ); 00187 } 00188 00189 //Math.asinh(a) - returns trig. hyperbolic arcsine of given angle in radians 00190 void scMathASinh(CScriptVar *c, void *userdata) { 00191 scReturnDouble( asinh( scGetDouble("a") ) ); 00192 } 00193 00194 //Math.cosh(a) - returns trig. hyperbolic cosine of given angle in radians 00195 void scMathCosh(CScriptVar *c, void *userdata) { 00196 scReturnDouble( cosh( scGetDouble("a") ) ); 00197 } 00198 00199 //Math.acosh(a) - returns trig. hyperbolic arccosine of given angle in radians 00200 void scMathACosh(CScriptVar *c, void *userdata) { 00201 scReturnDouble( acosh( scGetDouble("a") ) ); 00202 } 00203 00204 //Math.tanh(a) - returns trig. hyperbolic tangent of given angle in radians 00205 void scMathTanh(CScriptVar *c, void *userdata) { 00206 scReturnDouble( tanh( scGetDouble("a") ) ); 00207 } 00208 00209 //Math.atan(a) - returns trig. hyperbolic arctangent of given angle in radians 00210 void scMathATanh(CScriptVar *c, void *userdata) { 00211 scReturnDouble( atan( scGetDouble("a") ) ); 00212 } 00213 00214 //Math.E() - returns E Neplero value 00215 void scMathE(CScriptVar *c, void *userdata) { 00216 scReturnDouble(k_E); 00217 } 00218 00219 //Math.log(a) - returns natural logaritm (base E) of given value 00220 void scMathLog(CScriptVar *c, void *userdata) { 00221 scReturnDouble( log( scGetDouble("a") ) ); 00222 } 00223 00224 //Math.log10(a) - returns logaritm(base 10) of given value 00225 void scMathLog10(CScriptVar *c, void *userdata) { 00226 scReturnDouble( log10( scGetDouble("a") ) ); 00227 } 00228 00229 //Math.exp(a) - returns e raised to the power of a given number 00230 void scMathExp(CScriptVar *c, void *userdata) { 00231 scReturnDouble( exp( scGetDouble("a") ) ); 00232 } 00233 00234 //Math.pow(a,b) - returns the result of a number raised to a power (a)^(b) 00235 void scMathPow(CScriptVar *c, void *userdata) { 00236 scReturnDouble( pow( scGetDouble("a"), scGetDouble("b") ) ); 00237 } 00238 00239 //Math.sqr(a) - returns square of given value 00240 void scMathSqr(CScriptVar *c, void *userdata) { 00241 scReturnDouble( ( scGetDouble("a") * scGetDouble("a") ) ); 00242 } 00243 00244 //Math.sqrt(a) - returns square root of given value 00245 void scMathSqrt(CScriptVar *c, void *userdata) { 00246 scReturnDouble( sqrtf( scGetDouble("a") ) ); 00247 } 00248 00249 // ----------------------------------------------- Register Functions 00250 void registerMathFunctions(CTinyJS *tinyJS) { 00251 00252 // --- Math and Trigonometry functions --- 00253 tinyJS->addNative("function Math.abs(a)", scMathAbs, 0); 00254 tinyJS->addNative("function Math.round(a)", scMathRound, 0); 00255 tinyJS->addNative("function Math.min(a,b)", scMathMin, 0); 00256 tinyJS->addNative("function Math.max(a,b)", scMathMax, 0); 00257 tinyJS->addNative("function Math.range(x,a,b)", scMathRange, 0); 00258 tinyJS->addNative("function Math.sign(a)", scMathSign, 0); 00259 00260 tinyJS->addNative("function Math.PI()", scMathPI, 0); 00261 tinyJS->addNative("function Math.toDegrees(a)", scMathToDegrees, 0); 00262 tinyJS->addNative("function Math.toRadians(a)", scMathToRadians, 0); 00263 tinyJS->addNative("function Math.sin(a)", scMathSin, 0); 00264 tinyJS->addNative("function Math.asin(a)", scMathASin, 0); 00265 tinyJS->addNative("function Math.cos(a)", scMathCos, 0); 00266 tinyJS->addNative("function Math.acos(a)", scMathACos, 0); 00267 tinyJS->addNative("function Math.tan(a)", scMathTan, 0); 00268 tinyJS->addNative("function Math.atan(a)", scMathATan, 0); 00269 tinyJS->addNative("function Math.sinh(a)", scMathSinh, 0); 00270 tinyJS->addNative("function Math.asinh(a)", scMathASinh, 0); 00271 tinyJS->addNative("function Math.cosh(a)", scMathCosh, 0); 00272 tinyJS->addNative("function Math.acosh(a)", scMathACosh, 0); 00273 tinyJS->addNative("function Math.tanh(a)", scMathTanh, 0); 00274 tinyJS->addNative("function Math.atanh(a)", scMathATanh, 0); 00275 00276 tinyJS->addNative("function Math.E()", scMathE, 0); 00277 tinyJS->addNative("function Math.log(a)", scMathLog, 0); 00278 tinyJS->addNative("function Math.log10(a)", scMathLog10, 0); 00279 tinyJS->addNative("function Math.exp(a)", scMathExp, 0); 00280 tinyJS->addNative("function Math.pow(a,b)", scMathPow, 0); 00281 00282 tinyJS->addNative("function Math.sqr(a)", scMathSqr, 0); 00283 tinyJS->addNative("function Math.sqrt(a)", scMathSqrt, 0); 00284 00285 } 00286
Generated on Tue Jul 12 2022 21:01:11 by
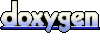