modified by ohneta
Dependents: HelloESP8266Interface_mine
Fork of NetworkSocketAPI by
SocketInterface.h
00001 /* SocketInterface Base Class 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef SOCKETINTERFACE_H 00018 #define SOCKETINTERFACE_H 00019 00020 #include "stdint.h" 00021 00022 /** This enum defines the possible socket domain types. 00023 */ 00024 typedef enum { 00025 SOCK_IPV4, /*!< IPv4 */ 00026 SOCK_IPV6, /*!< IPV6 */ 00027 } socket_domain_t; 00028 00029 /** This enum defines the possible socket types. 00030 */ 00031 typedef enum { 00032 SOCK_STREAM, /*!< Reliable stream-oriented service or Stream Sockets */ 00033 SOCK_DGRAM, /**< Datagram service or Datagram Sockets */ 00034 SOCK_SEQPACKET, /*!< Reliable sequenced packet service */ 00035 SOCK_RAW /*!< Raw protocols atop the network layer */ 00036 } socket_type_t; 00037 00038 /** This enum defines the ip protocols 00039 */ 00040 typedef enum { 00041 SOCK_TCP, /*!< Socket connection over TCP */ 00042 SOCK_UDP, /*!< Socket connection over UDP */ 00043 } socket_protocol_t; 00044 00045 /** Base class that defines an endpoint (TCP/UDP/Server/Client Socket) 00046 */ 00047 class Endpoint 00048 { 00049 public: 00050 00051 /** Get the ip address of a host by DNS lookup 00052 @param name The name of a host you need an ip address for 00053 @return The ip address of the host otherwise NULL 00054 */ 00055 virtual const char *getHostByName(const char *name) const = 0; 00056 00057 /** Set the address of this endpoint 00058 @param addr The endpoint address 00059 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00060 */ 00061 virtual void setAddress(const char* addr) = 0; 00062 00063 /** Set the port of this endpoint 00064 @param port The endpoint port 00065 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00066 */ 00067 virtual void setPort(uint16_t port) = 0; 00068 00069 /** Set the address and port of this endpoint 00070 @param addr The endpoint address (supplied as an ip address). 00071 @param port The endpoint port 00072 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00073 */ 00074 virtual void setAddressPort(const char* addr, uint16_t port) = 0; 00075 00076 /** Get the IP address of this endpoint 00077 @return The IP address of this endpoint. 00078 */ 00079 virtual const char *getAddress(void) const = 0; 00080 00081 /** Get the port of this endpoint 00082 @return The port of this socket 00083 */ 00084 virtual uint16_t getPort(void) const = 0; 00085 protected: 00086 const char* _addr; 00087 uint16_t _port; 00088 }; 00089 00090 /** SocketInterface class. 00091 * This is a common interface that is shared between all sockets that connect 00092 * using the NetworkInterface. 00093 */ 00094 class SocketInterface : public Endpoint 00095 { 00096 public: 00097 /** In server mode, set which port to listen on 00098 @param port The endpoint port 00099 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00100 */ 00101 virtual int32_t bind(uint16_t port) const = 0; 00102 00103 /** In server mode, start listening to a port 00104 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00105 */ 00106 virtual int32_t listen(void) const = 0; 00107 00108 /** In server mode, accept an incoming connection 00109 @param endpoint The endpoint we are listening to 00110 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00111 */ 00112 virtual int32_t accept() const = 0; 00113 00114 /** In client mode, open a connection to a remote host 00115 @param endpoint The endpoint we want to connect to 00116 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00117 */ 00118 virtual int32_t open() = 0; 00119 00120 /** In client or server mode send data 00121 @param data A buffer of data to send 00122 @param amount The amount of data to send 00123 @param timeout_ms The longest amount of time this send can take 00124 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00125 */ 00126 virtual int32_t send(const void *data, uint32_t amount, uint32_t timeout_ms = 15000) = 0; 00127 00128 /** In client or server mode receive data 00129 @param data a buffer to store the data in 00130 @param amount The amount of data to receive 00131 @param timeout_ms The longest time to wait for the data 00132 @return The amount of data received 00133 */ 00134 virtual uint32_t recv(void *data, uint32_t amount, uint32_t timeout_ms = 15000) = 0; 00135 00136 /** In client or server mode, close an open connection 00137 @param endpoint The endpoint we want to connect to 00138 @return 0 on success, -1 on failure (when an hostname cannot be resolved by DNS). 00139 */ 00140 virtual int32_t close() const = 0; 00141 00142 /** Get the socket's unique handle 00143 @return socket's handle number 00144 */ 00145 virtual uint32_t getHandle() const = 0; 00146 00147 protected: 00148 socket_protocol_t _type; 00149 uint32_t _handle; 00150 }; 00151 00152 #endif
Generated on Tue Jul 12 2022 18:45:36 by
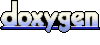