
同時公開のFunctionGenerator-DACと同時利用によりファンクション・ジェネレータを実現する。
Dependencies: BLE_API mbed nRF51822
FunctionGenCommands.h
00001 //--------------------------------------------------------- 00002 /** 00003 * FunctionGenCommands.h 00004 */ 00005 //--------------------------------------------------------- 00006 00007 #define FGC_BUFFERTRANSFER_MAX 16 00008 00009 typedef enum { 00010 // commands 00011 FGCommand_Non = 0x00, 00012 FGCommand_Device, 00013 FGCommand_Output, 00014 FGCommand_Frequency, 00015 FGCommand_WaveformInfo, 00016 FGCommand_WaveformBlock, 00017 FGCommand_WaveformToBuffer, 00018 00019 // status 00020 FGStatus_Device = 0x80 | FGCommand_Device, 00021 FGStatus_Output = 0x80 | FGCommand_Output, 00022 FGStatus_Frequency = 0x80 | FGCommand_Frequency, 00023 00024 // special 00025 FGCommand_Reset = 0xff, 00026 00027 } enumFGCommand; 00028 00029 00030 // Characteristics 00031 #define CHARACTERISTICS_UUID_RESET 0xA100 00032 #define CHARACTERISTICS_UUID_DEVICE 0xA101 00033 #define CHARACTERISTICS_UUID_OUTPUT 0xA102 00034 #define CHARACTERISTICS_UUID_FREQUENCY 0xA103 00035 #define CHARACTERISTICS_UUID_WAVEFORMINFO 0xA110 00036 #define CHARACTERISTICS_UUID_WAVEFORMBLOCK 0xA111 00037 #define CHARACTERISTICS_UUID_WAVEFORMTOBUFFER 0xA112 00038 00039 //--------------------------------------------------------- 00040 #pragma pack(1) 00041 // for BLE waveform transfer format 00042 00043 typedef struct { 00044 uint16_t waveSize; // Waveform全体のデータ長(byte) = blockSize * blockMaxNum 00045 uint8_t bitPerData; // 1データあたりのbit数 (8, 10, 12 or 16bit) 00046 00047 uint8_t blockSize; // 1ブロックあたりのデータ転送サイズ(byte) (通常16bytes) 00048 uint8_t blockMaxNum; // ブロックの最大数 = (waveSize + 1) / blockSize 00049 } WaveformInfo, FGCommandWaveformInfo; 00050 00051 typedef struct { 00052 uint16_t blockNo; // ブロックNo. 0 〜 (WaveformInfo.blockMaxNum - 1) 00053 uint8_t length; // WaveformBlock.bufferの有効な長さ 00054 uint8_t buffer[FGC_BUFFERTRANSFER_MAX]; // 実際のデータ 00055 } WaveformBlock, FGCommandWaveformBlock; 00056 00057 //--------------------------------------------------------- 00058 // for I2C packet format 00059 00060 // header 00061 typedef struct { 00062 uint8_t command; 00063 uint8_t length; 00064 } FGHeader; 00065 00066 // command bodys 00067 typedef struct { 00068 uint8_t device; 00069 } FGCommandDevice; 00070 00071 typedef struct { 00072 uint8_t on; 00073 } FGCommandOutput; 00074 00075 typedef struct { 00076 uint32_t frequency; 00077 } FGCommandFrequency; 00078 00079 //#define FGCommandWaveformInfo WaveformInfo 00080 //#define FGCommandWaveformBlock WaveformInfoBlock 00081 00082 typedef struct { 00083 } FGCommandWaveformToBuffer; 00084 00085 typedef struct { 00086 } FGCommandReset; 00087 00088 // status bodys 00089 typedef struct { 00090 uint8_t device; 00091 } FGStatusDevice; 00092 00093 typedef struct { 00094 uint8_t xxx; 00095 } FGStatusOutput; 00096 00097 typedef struct { 00098 uint8_t xxx; 00099 } FGStatusFrequency; 00100 00101 00102 00103 // command Packet format 00104 typedef struct { 00105 FGHeader header; 00106 union { 00107 FGCommandDevice commandDevice; 00108 FGCommandOutput commandOutput; 00109 FGCommandFrequency commandFrequency; 00110 FGCommandWaveformInfo commandWaveformInfo; 00111 FGCommandWaveformBlock commandWaveformBlock; 00112 FGCommandWaveformToBuffer commandWaveformToBuffer; 00113 00114 FGCommandReset commandReset; 00115 00116 FGStatusDevice statusDevice; 00117 FGStatusOutput statusOutput; 00118 FGStatusFrequency statusFrequency; 00119 } body; 00120 } FGPacket; 00121 00122 #pragma pack()
Generated on Sat Jul 16 2022 03:25:36 by
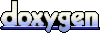