modified by ohneta
Dependents: HelloESP8266Interface_mine
Fork of ESP8266Interface by
ESP8266Interface.cpp
00001 /* ESP8266Interface Example 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "ESP8266Interface.h" 00018 00019 ESP8266Interface::ESP8266Interface(PinName tx, PinName rx) : esp8266(tx, rx) 00020 { 00021 uuidCounter = 0; 00022 std::fill_n(availableID, sizeof(availableID)/sizeof(int), -1); 00023 preferred_dnsip = NULL; 00024 } 00025 00026 int32_t ESP8266Interface::init(void) 00027 { 00028 if (!esp8266.startup()) { 00029 return -1; 00030 } 00031 if (!esp8266.reset()) { 00032 return -1; 00033 } 00034 if (!esp8266.wifiMode(3)) { 00035 return -1; 00036 } 00037 if (!esp8266.multipleConnections(true)) { 00038 return -1; 00039 } 00040 return 0; 00041 } 00042 00043 int32_t ESP8266Interface::init(const char *ip, const char *mask, const char *gateway) 00044 { 00045 return -1; 00046 } 00047 00048 int32_t ESP8266Interface::connect(uint32_t timeout_ms) 00049 { 00050 return -1; 00051 } 00052 00053 int32_t ESP8266Interface::connect(const char *ap, const char *pass_phrase, wifi_security_t security, uint32_t timeout_ms) 00054 { 00055 esp8266.setTimeout(timeout_ms); 00056 if (!esp8266.dhcp(1, true)) { 00057 return -1; 00058 } 00059 if (!esp8266.connect(ap, pass_phrase)) { 00060 return -1; 00061 } 00062 return 0; 00063 } 00064 00065 int32_t ESP8266Interface::disconnect(void) 00066 { 00067 if (!esp8266.disconnect()) { 00068 return -1; 00069 } 00070 00071 for(int i=0; i<numSockets; i++) { 00072 SocketInterface *socket = sockets[availableID[i]]; 00073 if (availableID[i] != -1) { 00074 deallocateSocket(socket); 00075 } 00076 } 00077 return 0; 00078 } 00079 00080 char *ESP8266Interface::getIPAddress(void) 00081 { 00082 if(!esp8266.getIPAddress(ip)) { 00083 return NULL; 00084 } 00085 return ip; 00086 } 00087 00088 char *ESP8266Interface::getGateway(void) const 00089 { 00090 return 0; 00091 } 00092 00093 char *ESP8266Interface::getNetworkMask(void) const 00094 { 00095 return 0; 00096 } 00097 00098 char *ESP8266Interface::getMACAddress(void) const 00099 { 00100 return 0; 00101 } 00102 00103 int32_t ESP8266Interface::isConnected(void) 00104 { 00105 return (getIPAddress() == NULL) ? -1 : 0; 00106 } 00107 00108 SocketInterface *ESP8266Interface::allocateSocket(socket_protocol_t socketProtocol) 00109 { 00110 int id = -1; 00111 //Look through the array of available sockets for an unused ID 00112 for(int i=0; i<numSockets; i++) { 00113 if (availableID[i] == -1) { 00114 id = i; 00115 availableID[i] = uuidCounter; 00116 break; 00117 } 00118 } 00119 if (id == -1) { 00120 return NULL;//tried to allocate more than the maximum 5 sockets 00121 } 00122 ESP8266Socket *socket = new ESP8266Socket(uuidCounter++, esp8266, socketProtocol, (uint8_t)id); 00123 sockets[socket->getHandle()] = socket; 00124 return socket; 00125 } 00126 00127 int ESP8266Interface::deallocateSocket(SocketInterface *socket) 00128 { 00129 int id = (int)static_cast<ESP8266Socket*>(socket)->getID(); 00130 availableID[id] = -1; 00131 00132 std::map<uint32_t, SocketInterface*>::iterator it; 00133 00134 // Check if socket is owned by WiFiRadioInterface 00135 it = sockets.find(socket->getHandle()); 00136 00137 if (it != sockets.end()) { 00138 // If so, erase it from the internal socket map and deallocate the socket 00139 sockets.erase(it); 00140 delete socket; 00141 } else { 00142 // Socket is not owned by WiFiRadioInterface, so return -1 error 00143 return -1; 00144 } 00145 return 0; 00146 } 00147 void ESP8266Interface::getHostByName(const char *name, char* hostIP) 00148 { 00149 SocketInterface* sock = this->allocateSocket(SOCK_UDP); 00150 DnsQuery dns(sock, name, hostIP, this->preferred_dnsip); 00151 this->deallocateSocket(sock); 00152 } 00153 00154 void ESP8266Interface::setPreferredDns(const char *preferredDnsIP) 00155 { 00156 this->preferred_dnsip = preferredDnsIP; 00157 } 00158 00159 ESP8266Socket::ESP8266Socket(uint32_t handle, ESP8266 &driver, socket_protocol_t type, uint8_t id) 00160 { 00161 _handle = handle; 00162 _driver = &driver; 00163 _type = type; 00164 _id = id; 00165 } 00166 00167 const char *ESP8266Socket::getHostByName(const char *name) const 00168 { 00169 return 0; 00170 } 00171 00172 void ESP8266Socket::setAddress(const char* addr) 00173 { 00174 _addr = addr; 00175 } 00176 00177 void ESP8266Socket::setPort(uint16_t port) 00178 { 00179 _port = port; 00180 } 00181 00182 void ESP8266Socket::setAddressPort(const char* addr, uint16_t port) 00183 { 00184 _addr = addr; 00185 _port = port; 00186 } 00187 00188 const char *ESP8266Socket::getAddress(void) const 00189 { 00190 return _addr; 00191 } 00192 00193 uint16_t ESP8266Socket::getPort(void) const 00194 { 00195 return _port; 00196 } 00197 00198 00199 int32_t ESP8266Socket::bind(uint16_t port) const 00200 { 00201 return -1; 00202 } 00203 00204 int32_t ESP8266Socket::listen(void) const 00205 { 00206 return -1; 00207 } 00208 00209 int32_t ESP8266Socket::accept() const 00210 { 00211 return -1; 00212 } 00213 00214 int32_t ESP8266Socket::open() 00215 { 00216 00217 string sock_type = (SOCK_UDP == _type) ? "UDP" : "TCP"; 00218 if (!_driver->openSocket(sock_type, _id, _port, _addr)) { 00219 return -1; 00220 } 00221 return 0; 00222 00223 } 00224 00225 int32_t ESP8266Socket::send(const void *data, uint32_t amount, uint32_t timeout_ms) 00226 { 00227 _driver->setTimeout((int)timeout_ms); 00228 if(!_driver->sendData(_id, data, amount)) { 00229 return -1; 00230 } 00231 return 0; 00232 } 00233 00234 uint32_t ESP8266Socket::recv(void *data, uint32_t amount, uint32_t timeout_ms) 00235 { 00236 _driver->setTimeout((int)timeout_ms); 00237 return _driver->recv(data, amount); 00238 } 00239 00240 int32_t ESP8266Socket::close() const 00241 { 00242 if (!_driver->close(_id)) { 00243 return -1;//closing socket not succesful 00244 } 00245 return 0; 00246 } 00247 00248 uint32_t ESP8266Socket::getHandle()const 00249 { 00250 return _handle; 00251 } 00252 00253 uint8_t ESP8266Socket::getID() 00254 { 00255 return _id; 00256 }
Generated on Tue Jul 12 2022 22:17:07 by
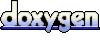