
This program can detect the type(mbed or LPCXpresso) of platform. The sample recognizes mbed or LPCXpresso.
PlatformInfo.cpp
00001 #include "PlatformInfo.h" 00002 #define REG_HCSR 0xe000edf0 00003 #define REG_DEMCR 0xE000EDFC 00004 00005 #include "mbed.h" 00006 namespace MiMic 00007 { 00008 int PlatformInfo::_pftype=PF_UNKNOWN; 00009 void PlatformInfo::check() 00010 { 00011 #if PlatformInfo_DETECTION_MODE==PlatformInfo_DETECTION_MODE_MBED 00012 _pftype=PF_MBED; 00013 return; 00014 #elif PlatformInfo_DETECTION_MODE==PlatformInfo_DETECTION_MODE_LPCXPRESSO 00015 _pftype=PF_LPCXPRESSO; 00016 return; 00017 #elif PlatformInfo_DETECTION_MODE==PlatformInfo_DETECTION_MODE_AUTO 00018 //LPCXpresso is return S_RESET_ST==1 when standalone. 00019 wait_ms(200); 00020 unsigned int v; 00021 v=*(unsigned int*)REG_HCSR; 00022 //check Debug Halting Control and Status::S_RESET_ST sticky bit 00023 if((v & 0x02000000)!=0){ 00024 //may be LPC-Standalone 00025 _pftype=PF_LPCXPRESSO; 00026 return; 00027 } 00028 v=(*(unsigned int*)REG_DEMCR); 00029 if((v & 0x01000000)==0x0){ 00030 //may be mbed 00031 _pftype=PF_MBED; 00032 return; 00033 } 00034 _pftype=PF_LPCXPRESSO; 00035 return; 00036 #else 00037 #error "ERROR!" 00038 #endif 00039 } 00040 int PlatformInfo::getPlatformType() 00041 { 00042 if(_pftype==PF_UNKNOWN){ 00043 check(); 00044 } 00045 return _pftype; 00046 } 00047 }
Generated on Sat Aug 6 2022 05:24:45 by
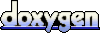