
japanese tweeting sample with newer version libraries
Embed:
(wiki syntax)
Show/hide line numbers
TwitterExample.cpp
00001 /* 00002 Update: 21-06-2010 00003 The basic authentication service for twitter is going down at the end of the week. 00004 To continue using that program, the code has been updated to use http://supertweet.net which acts as an API proxy. 00005 Simply visit the website to setup your twitter account for this API. 00006 See: http://www.supertweet.net/about/documentation 00007 */ 00008 00009 #include "mbed.h" 00010 #include "EthernetNetIf.h" 00011 #include "HTTPClient.h" 00012 #include "TextLCD.h" 00013 00014 TextLCD lcd(p24, p25, p26, p27, p28, p29); // rs, e, d0-d3 00015 //TextLCD lcd( p24, p26, p27, p28, p29, p30 ); // rs, e, d0-d3 00016 00017 EthernetNetIf eth; 00018 00019 int main() { 00020 00021 printf("Init\n"); lcd.locate( 0, 0 ); lcd.printf("Init "); 00022 printf("\r\nSetting up...\r\n"); lcd.locate( 0, 0 ); lcd.printf("Setting up "); 00023 00024 EthernetErr ethErr = eth.setup(); 00025 if(ethErr) 00026 { 00027 printf("Error %d in setup.\n", ethErr); lcd.locate( 0, 0 ); lcd.printf("error %d", ethErr); 00028 return -1; 00029 } 00030 printf("\r\nSetup OK\r\n"); lcd.locate( 0, 0 ); lcd.printf("Setup OK "); 00031 00032 HTTPClient twitter; 00033 00034 HTTPMap msg; 00035 00036 //msg["status"] = "twitter test AAAA"; //A good example of Key/Value pair use with Web APIs 00037 LocalFileSystem local("local"); 00038 char s[256]; 00039 FILE *fp; 00040 00041 printf("\r\nreading a message file.\r\n"); 00042 00043 if(NULL == (fp = fopen("/local/tweet.txt","r")) ) { 00044 printf("\r\nError: The message file cannot be accessed\r\n"); lcd.locate( 0, 0 ); lcd.printf("File access error"); 00045 return -1; 00046 } 00047 00048 fgets(s,256,fp); 00049 fclose(fp); 00050 00051 msg["status"] = s; lcd.locate( 0, 0 ); lcd.printf("File read done "); 00052 twitter.basicAuth("USER_ID", "PASSWORD"); //We use basic authentication, replace with you account's parameters 00053 00054 //No need to retieve data sent back by the server 00055 HTTPResult r = twitter.post("http://api.supertweet.net/1/statuses/update.xml", msg, NULL); 00056 if( r == HTTP_OK ) 00057 { 00058 printf("Tweet sent with success!\n"); lcd.locate( 0, 0 ); lcd.printf("Done "); 00059 } 00060 else 00061 { 00062 printf("Problem during tweeting, return code %d\n", r);lcd.locate( 0, 0 ); lcd.printf("Got error "); 00063 } 00064 00065 return 0; 00066 00067 }
Generated on Wed Jul 13 2022 03:05:34 by
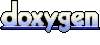