Abstract class for LED driver component. Including "LedPwmOut API" class.
Embed:
(wiki syntax)
Show/hide line numbers
LedPwmOut.h
00001 /** LedPwmOut class for LED driver component 00002 * 00003 * @author Akifumi (Tedd) OKANO, NXP Semiconductors 00004 * @version 0.5 00005 * @date 04-Mar-2015 00006 * 00007 * Released under the Apache 2 license License 00008 */ 00009 00010 #ifndef MBED_LedPwmOut 00011 #define MBED_LedPwmOut 00012 00013 #include "mbed.h" 00014 #include "CompLedDvr.h" 00015 00016 /** LedPwmOut class 00017 * 00018 * @class LedPwmOut 00019 * 00020 * "LedPwmOut" class works like "PwmOut" class of mbed-SDK. 00021 * This class provides API on device's pin level with abstracting the LED controller. 00022 * 00023 * Example: 00024 * @code 00025 * #include "mbed.h" 00026 * #include "PCA9626.h" 00027 * 00028 * PCA9626 led_cntlr( p28, p27, 0xC4 ); // SDA, SCL, Slave_address(option) 00029 * LedPwmOut led( led_cntlr, L0 ); 00030 * 00031 * int main() 00032 * { 00033 * while( 1 ) { 00034 * for( float p = 0.0f; p < 1.0f; p += 0.1f ) { 00035 * led = p; 00036 * wait( 0.1 ); 00037 * } 00038 * } 00039 * } 00040 * @endcode 00041 */ 00042 class LedPwmOut 00043 { 00044 public: 00045 00046 /** Create a LedPwmOutCC instance connected to a pin on the LED driver 00047 * A pin which performs PWM and constant current sink 00048 * 00049 * @param ledp Instance of a device (LED driver) 00050 * @param pin_name Specifying pin by LedPinName like 'L7'. 00051 * 00052 * @note 00053 * Pin names of LED driver are defined like L0, L1, L2.. It is not like "LED0". 00054 * Because we cannot use mbed reserved symbols. 00055 */ 00056 LedPwmOut( CompLedDvr &ledp, LedPinName pin_name ); 00057 00058 /** Destractor 00059 */ 00060 virtual ~LedPwmOut(); 00061 00062 /** Set PWM duty-cycle 00063 * 00064 * @param v Ratio of duty-cycle. '0.0' for 0 %. '1.0' for 99.6 % on PCA9956A and 100 % for PCA9955A. 00065 */ 00066 virtual void pwm( float v ); 00067 00068 /** A shorthand for pwm() 00069 */ 00070 LedPwmOut& operator= ( float rhs ); 00071 00072 private: 00073 CompLedDvr *leddvrp; 00074 LedPinName pin; 00075 00076 void pwm( int pin, float value ); 00077 } 00078 ; 00079 00080 #endif // MBED_LedPwmOut
Generated on Tue Jul 12 2022 18:53:22 by
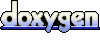