
Firmware for MC33926 evaluation on KL25Z-based EVMB
Fork of Brushed_DC_Motor_Control_MC34931_MC33931 by
main_copy.cpp
00001 #include "mbed.h" 00002 #include "USBHID.h" 00003 00004 // We declare a USBHID device. 00005 // HID In/Out Reports are 64 Bytes long 00006 // Vendor ID (VID): 0x15A2 00007 // Product ID (PID): 0x0138 00008 // Serial Number: 0x0001 00009 USBHID hid(64, 64, 0x15A2, 0x0138, 0x0001, true); 00010 00011 //Setup Digital Outputs for the LEDs on the FRDM 00012 //PwmOut red_led(LED1); 00013 //DigitalOut green_led(LED2); 00014 //DigitalOut blue_led(LED3); 00015 00016 //Setup PWM and Digital Outputs from FRDM-KL25Z to FRDM-17510 00017 PwmOut IN1(PTA5); // Pin IN1 input to MC33926 (FRDM PIN Name) 00018 PwmOut IN2(PTC8); // Pin IN2 input to MC33926 (FRDM PIN Name) 00019 DigitalOut EN(PTE0); // Pin EN input to MC33926 (FRDM PIN Name) 00020 DigitalOut DIS1(PTA2); // Pin D1 input to MC33926 (FRDM PIN Name) 00021 DigitalOut D2B(PTD5); // Pin D2B input to MC33926 (FRDM PIN Name) 00022 DigitalOut SLEW(PTA13); // Pin Slew input to MC33926 (FRDM PIN Name) 00023 DigitalOut INV(PTD0); // Pin INV input to MC33926 (FRDM PIN Name) 00024 DigitalIn SFB(PTB3); // Pin SF_B output from MC33926 to FRDM-KL25Z 00025 AnalogIn CFB(PTB0); // Pin FB output from MC33926 to FRDM-KL25Z 00026 //DigitalOut READY(PTC7); // Pin READY input to Motor Control Board (FRDM PIN Name) 00027 00028 //Variables 00029 int pwm_freq_lo; 00030 int pwm_freq_hi; 00031 int frequencyHz = 500; 00032 int runstop = 0; 00033 int direction = 1; 00034 int braking; // needs to be initialized? 00035 int dutycycle = 75; 00036 int newDataFlag = 0; 00037 int status = 0; 00038 uint16_t CurrFB; 00039 uint16_t CFBArray[101]; 00040 uint32_t CFBTotal; 00041 uint16_t CFBAvg; 00042 uint16_t CFBtemp; 00043 00044 //storage for send and receive data 00045 HID_REPORT send_report; 00046 HID_REPORT recv_report; 00047 00048 bool initflag = true; 00049 00050 // USB COMMANDS 00051 // These are sent from the PC 00052 #define WRITE_LED 0x20 00053 #define WRITE_GEN_EN 0x40 // what is this? What should the GUI kickoff here? 00054 #define WRITE_DUTY_CYCLE 0x50 00055 #define WRITE_PWM_FREQ 0x60 00056 #define WRITE_RUN_STOP 0x70 00057 #define WRITE_DIRECTION 0x71 00058 #define WRITE_BRAKING 0x90 00059 #define WRITE_RESET 0xA0 // what is this? What should the GUI kickoff here? 00060 #define WRITE_D1 0xB1 00061 #define WRITE_EN 0xC1 00062 #define WRITE_D2B 0xD5 00063 #define WRITE_SLEW 0xE5 00064 #define WRITE_INV 0xF5 00065 00066 #define scaleFactor 0.11868 00067 00068 // LOGICAL CONSTANTS 00069 #define OFF 0x00 00070 #define ON 0x01 00071 00072 00073 int main() 00074 { 00075 send_report.length = 64; 00076 recv_report.length = 64; 00077 00078 00079 while(1) 00080 { 00081 //try to read a msg 00082 if(hid.readNB(&recv_report)) 00083 { 00084 switch(recv_report.data[0]) //byte 0 of recv_report.data is command 00085 { 00086 //----------------------------------------------------------------------------------------------------------------- 00087 // COMMAND PARSER 00088 //----------------------------------------------------------------------------------------------------------------- 00089 //////// 00090 case WRITE_LED: 00091 break; 00092 //////// 00093 00094 //////// 00095 case WRITE_DUTY_CYCLE: 00096 dutycycle = recv_report.data[1]; 00097 newDataFlag = 1; 00098 break; 00099 //////// 00100 case WRITE_PWM_FREQ: //PWM frequency can be larger than 1 byte 00101 pwm_freq_lo = recv_report.data[1]; //so we have to re-assemble the number 00102 pwm_freq_hi = recv_report.data[2] * 100; 00103 frequencyHz = pwm_freq_lo + pwm_freq_hi; 00104 newDataFlag = 1; 00105 break; 00106 //////// 00107 case WRITE_RUN_STOP: 00108 newDataFlag = 1; 00109 if(recv_report.data[1] != 0) 00110 { 00111 runstop = 1; 00112 } 00113 else 00114 { 00115 runstop = 0; 00116 } 00117 break; 00118 00119 //////// 00120 case WRITE_DIRECTION: 00121 newDataFlag = 1; 00122 if(recv_report.data[1] == 1) // used to be != 0 00123 { 00124 direction = 1; // corrected allocation for FWD 00125 //direction = 0; // corrected allocation for REV 00126 } 00127 else 00128 { 00129 direction = 0; // corrected allocation for REV 00130 //direction = 1; // corrected allocation for FWD 00131 } 00132 break; 00133 //////// 00134 case WRITE_BRAKING: 00135 newDataFlag = 1; 00136 if(recv_report.data[1] != 0) // used to be == 1 00137 { 00138 braking = 1; // this is HS recirc 00139 } 00140 else 00141 { 00142 braking = 0; // this is LS recirc 00143 } 00144 break; 00145 //////// 00146 case WRITE_D1: 00147 newDataFlag = 1; 00148 if(recv_report.data[1] == 1) 00149 { 00150 DIS1 = 1; // logic hi will disable the part 00151 } 00152 else 00153 { 00154 DIS1 = 0; // logic lo will enable the part 00155 } 00156 break; 00157 //////// 00158 case WRITE_EN: 00159 newDataFlag = 1; 00160 if(recv_report.data[1] == 1) 00161 { 00162 //EN = 0; // this is enable case 00163 EN = 1; // align with the signal being sent from GUI 00164 } 00165 else 00166 { 00167 //EN = 1; // this is disable case 00168 EN = 0; // align with signal being sent from GUI 00169 } 00170 break; 00171 //////// 00172 case WRITE_D2B: 00173 newDataFlag = 1; 00174 if(recv_report.data[1] == 1) 00175 { 00176 D2B = 1; 00177 } 00178 else 00179 { 00180 D2B = 0; 00181 } 00182 break; 00183 00184 //////// 00185 case WRITE_SLEW: 00186 newDataFlag = 1; 00187 if(recv_report.data[1] == 1) 00188 { 00189 SLEW = 1; 00190 } 00191 else 00192 { 00193 SLEW = 0; 00194 } 00195 break; 00196 00197 //////// 00198 case WRITE_INV: 00199 newDataFlag = 1; 00200 if(recv_report.data[1] == 1) 00201 { 00202 INV = 1; 00203 //INV = 0; 00204 } 00205 else 00206 { 00207 INV = 0; 00208 //INV = 1; 00209 } 00210 break; 00211 00212 00213 //////// 00214 default: 00215 break; 00216 }// End Switch recv report data[0] 00217 00218 //----------------------------------------------------------------------------------------------------------------- 00219 // end command parser 00220 //----------------------------------------------------------------------------------------------------------------- 00221 00222 status = SFB; 00223 send_report.data[0] = status; // Echo Command 00224 send_report.data[1] = recv_report.data[1]; // Echo Subcommand 1 00225 send_report.data[2] = recv_report.data[2]; // Echo Subcommand 2 00226 send_report.data[3] = 0x00; 00227 send_report.data[4] = 0x00; 00228 send_report.data[5] = 0x00; 00229 send_report.data[6] = (CFBAvg << 8) >> 8; 00230 send_report.data[7] = CFBAvg >> 8; 00231 00232 //Send the report 00233 hid.send(&send_report); 00234 }// End If(hid.readNB(&recv_report)) 00235 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00236 //End of USB message handling 00237 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00238 00239 CurrFB = CFB.read_u16(); 00240 CFBArray[0] = CurrFB; 00241 CFBTotal = 0; 00242 int i = 0; 00243 for(i=0; i<100; i++) 00244 { 00245 CFBTotal = CFBTotal + CFBArray[i]; 00246 } 00247 CFBAvg = CFBTotal / 100; 00248 00249 for(i=100; i>=0; i--) 00250 { 00251 00252 CFBArray[i+1] = CFBArray[i]; 00253 } 00254 00255 if(newDataFlag != 0) //GUI setting changed 00256 { 00257 newDataFlag = 0; 00258 if(runstop != 0) //Running 00259 { 00260 if(direction == 0) //reverse 00261 { 00262 if(braking == 1) //dynamic 00263 { 00264 IN1.period(1/(float)frequencyHz); 00265 //IN1 = (float)dutycycle/100.0; // this is REV + HS, inverted d 00266 IN1 = 1.0-((float)dutycycle/100.0); // this is REV + HS 00267 IN2 = 1; 00268 } 00269 else //coast 00270 { 00271 IN1 = 0; // this is REV + LS 00272 IN2.period(1/(float)frequencyHz); 00273 IN2 = (float)dutycycle/100.0; 00274 } 00275 } 00276 else //forward 00277 { 00278 if(braking == 1) //dynamic 00279 { 00280 IN1 = 1; 00281 IN2.period(1/(float)frequencyHz); 00282 //IN2 = (float)dutycycle/100.0; // this is FWD + HS, inverted d 00283 IN2 = 1.0-((float)dutycycle/100.0); // this is FWD + HS 00284 } 00285 else //coast 00286 { 00287 IN1.period(1/(float)frequencyHz); 00288 IN1 = (float)dutycycle/100.0; 00289 IN2 = 0; // FWD + LS 00290 } 00291 } 00292 } 00293 else //Stopped 00294 { 00295 if(braking == 1) //braking 00296 { 00297 IN1.period(1); 00298 IN2.period(1); 00299 IN1.write(1); 00300 IN2.write(1); 00301 } 00302 else //coasting 00303 { 00304 IN1.period(1); 00305 IN2.period(1); 00306 IN1.write(0); 00307 IN2.write(0); 00308 00309 } 00310 } 00311 } 00312 } 00313 } 00314
Generated on Sat Jul 23 2022 14:05:14 by
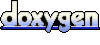