
fds
Dependencies: BLE_API mbed nRF51822
main.cpp
00001 #include "ble/BLE.h" 00002 #include "mbed.h" 00003 00004 BLE ble; 00005 Timeout timeout; 00006 00007 #define TXRX_BUF_LEN 20 00008 Serial pc(USBTX, USBRX); 00009 00010 //**********TAG Personalization*********************** 00011 //**************************************************** 00012 char TAG_ADDRESS[]="D2:FB:BE:68:EF:5A"; 00013 //char TAG_ADDRESS[]="EE:04:D4:1A:9C:16"; 00014 //char TAG_ADDRESS[]="F6:99:64:A9:FD:6D"; 00015 //char TAG_ADDRESS[]="D6:F7:8B:78:5D:BD"; 00016 //char TAG_ADDRESS[]="D7:8F:65:5C:A0:B9"; 00017 int password=135; 00018 //**************************************************** 00019 00020 00021 00022 int stealthModeActive=0; 00023 uint8_t chars_value[TXRX_BUF_LEN] = {0,}; 00024 00025 // The Nordic UART Service 00026 static const uint8_t cityRiskServiceUuid[] = {0x71, 0x3D, 0, 0, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00027 static const uint8_t cityRiskCharacteristcUuid[] = {0x71, 0x3D, 0, 2, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00028 static const uint8_t uart_base_uuid_rev[] = {0x1E, 0x94, 0x8D, 0xF1, 0x48, 0x31, 0x94, 0xBA, 0x75, 0x4C, 0x3E, 0x50, 0, 0, 0x3D, 0x71}; 00029 00030 00031 GattCharacteristic cityRiskCharacteristic(cityRiskCharacteristcUuid, chars_value, 3, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE); 00032 00033 GattCharacteristic *cityRiskChars[] = {&cityRiskCharacteristic}; 00034 00035 GattService cityRiskService(cityRiskServiceUuid, cityRiskChars, sizeof(cityRiskChars) / sizeof(GattCharacteristic *)); 00036 00037 00038 //static void scanCallBack(const Gap::AdvertisementCallbackParams_t *params); 00039 00040 /************************************************************************************* 00041 *Function Name: autodisconnect 00042 ************************************************************************************** 00043 * Summary: Function is called to disconnect automatically 00044 * 00045 * 00046 * Parameters: 00047 * None 00048 * 00049 * Return: 00050 * None 00051 * 00052 ************************************************************************************/ 00053 void autodisconnect(){ 00054 00055 if (ble.getGapState().connected){ 00056 ble.gap().disconnect(Gap::LOCAL_HOST_TERMINATED_CONNECTION); 00057 // pc.printf("Autodisconnect\n"); 00058 } 00059 } 00060 /************************************************************************************* 00061 *Function Name: beaconInit 00062 ************************************************************************************** 00063 * Summary: Setup peripheral profile 00064 * 00065 * 00066 * Parameters: 00067 * None 00068 * 00069 * Return: 00070 * None 00071 * 00072 ************************************************************************************/ 00073 void beaconInit (void){ 00074 ble.gap().startAdvertising(); 00075 // pc.printf("beacon Init\n"); 00076 } 00077 00078 /************************************************************************************* 00079 *Function Name: ble_advdata_decode 00080 ************************************************************************************** 00081 * Summary: Used by the scan call back function to declare a successful scan. 00082 * 00083 * 00084 * Parameters: 00085 * BLE_GAP_AD_TYPE_SHORT_LOCAL_NAME, params->advertisingDataLen, 00086 * (uint8_t *)params->advertisingData, &len, adv_name : Parameters the adverising device sends 00087 * and the scan retrieves 00088 * 00089 * Return: 00090 * NRF Succes or not 00091 * 00092 ************************************************************************************/ 00093 int ble_advdata_decode(uint8_t type, uint8_t advdata_len, uint8_t *p_advdata, uint8_t *len, uint8_t *p_field_data){ 00094 00095 uint8_t index=0; 00096 uint8_t field_length, field_type; 00097 00098 while(index<advdata_len){ 00099 field_length = p_advdata[index]; 00100 field_type = p_advdata[index+1]; 00101 if(field_type == type){ 00102 memcpy(p_field_data, &p_advdata[index+2], (field_length-1)); 00103 *len = field_length - 1; 00104 return 1; 00105 } 00106 index += field_length + 1; 00107 } 00108 return 0; 00109 } 00110 /************************************************************************************* 00111 *Function Name: scanCallBack 00112 ************************************************************************************** 00113 * Summary: Start scan for a device. When a spesific device defined by a certain name 00114 * is scanned, the device changes from Central to Peripheral. 00115 * 00116 * 00117 * Parameters: 00118 * Gap::AdvertisementCallbackParams_t *params: The Params returned by the scan. The 00119 * Params are the ones the peripheral device advertises. 00120 * 00121 * Return: 00122 * None 00123 * 00124 ************************************************************************************/ 00125 static void scanCallBack(const Gap::AdvertisementCallbackParams_t *params){ 00126 00127 uint8_t len; 00128 uint8_t adv_name[31]; 00129 // pc.printf("packetscanned\n"); 00130 if( 1 == ble_advdata_decode(0x09, params->advertisingDataLen, (uint8_t *)params->advertisingData, &len, adv_name) ){ 00131 // pc.printf("stage1\n"); 00132 //pc.printf("%x",&adv_name,"\n"); 00133 if (memcmp(TAG_ADDRESS, adv_name, len) == 0x00){ 00134 ble.stopScan(); 00135 stealthModeActive=0; 00136 beaconInit(); 00137 // pc.printf("activation\n"); 00138 } 00139 } 00140 } 00141 /************************************************************************************* 00142 *Function Name: stealthModeInit 00143 ************************************************************************************** 00144 * Summary: Initiate central profile 00145 * 00146 * 00147 * Parameters: 00148 * None 00149 * 00150 * Return: 00151 * None 00152 * 00153 ************************************************************************************/ 00154 void stealthModeInit(void){ 00155 ble.gap().startScan(scanCallBack); 00156 pc.printf("stealthmode\n"); 00157 } 00158 00159 00160 00161 /************************************************************************************** 00162 *Function Name: writtenHandle * 00163 *************************************************************************************** 00164 * Summary: GATT call back handle. When a write service is offered by the device this * 00165 * function handles the data the client sends. * 00166 * * 00167 * * 00168 * Parameters: * 00169 * GattWriteCallbackParams *Handler: The Params returned by a write action of the * 00170 * client. * 00171 * * 00172 * Return: * 00173 * None * 00174 * * 00175 **************************************************************************************/ 00176 void gattServerWriteCallBack(const GattWriteCallbackParams *Handler) { 00177 uint8_t index; 00178 uint8_t buf[TXRX_BUF_LEN]; 00179 uint16_t bytesRead; 00180 int code; 00181 /*if (Handler->handle == cityRiskCharacteristic.getValueAttribute().getHandle()){ 00182 00183 }*/ 00184 pc.printf("writecallback\n"); 00185 ble.readCharacteristicValue(cityRiskCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00186 /*for( index=0; index<bytesRead; index++){ 00187 code=buf[index]; 00188 pc.printf(code,"\n"); 00189 }*/ 00190 code=Handler->data[0]; 00191 // pc.printf("%d",code,"\n"); 00192 00193 if (code==password){ 00194 stealthModeActive=1; 00195 // pc.printf("Success\n"); 00196 } 00197 00198 //ble.disconnect(Gap::LOCAL_HOST_TERMINATED_CONNECTION); 00199 } 00200 00201 /************************************************************************************* 00202 *Function Name: disconnectionCallBack 00203 ************************************************************************************** 00204 * Summary: Function is called when our device disconnects from another device 00205 * 00206 * 00207 * Parameters: 00208 * Gap::Handle_t handle, Gap::DisconnectionReason_t reason 00209 * 00210 * Return: 00211 * None 00212 * 00213 ************************************************************************************/ 00214 void disconnectionCallBack(const Gap::DisconnectionCallbackParams_t *params) 00215 { 00216 // pc.printf("disconnect\n"); 00217 if (stealthModeActive==0){ 00218 beaconInit(); 00219 } 00220 else{ 00221 stealthModeInit(); 00222 } 00223 } 00224 00225 /************************************************************************************* 00226 *Function Name: ConnectionCallBack 00227 ************************************************************************************** 00228 * Summary: Function is called when our device connects to another device 00229 * 00230 * 00231 * Parameters: 00232 * Gap::ConnectionCallbackParams_t 00233 * 00234 * Return: 00235 * None 00236 * 00237 ************************************************************************************/ 00238 void connectionCallBack( const Gap::ConnectionCallbackParams_t *params ){ 00239 // pc.printf("Connected\n"); 00240 timeout.attach(autodisconnect, 3); 00241 //disconnection time out after 3 sec central device is get disconnected! 00242 } 00243 /************************************************************************************* 00244 *Function Name: setup 00245 ************************************************************************************** 00246 * Summary: Basic setup for the SoC. Setup for central/peripheral roles 00247 * 00248 * 00249 * Parameters: 00250 * None 00251 * 00252 * Return: 00253 * None 00254 * 00255 ************************************************************************************/ 00256 int main(void) 00257 { 00258 ble.init(); 00259 ble.onConnection(connectionCallBack); 00260 ble.onDisconnection(disconnectionCallBack); 00261 ble.onDataWritten(gattServerWriteCallBack); 00262 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00263 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00264 (const uint8_t *)"Beacon02", sizeof("Beacon02") - 1); 00265 //change here complete local name 00266 // ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS,(const uint8_t *)uart_base_uuid_rev, sizeof(uart_base_uuid_rev)); 00267 // to check the operation commenting uart_base_uuid_rev 00268 // set adv_type 00269 //ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::DEVICE_ID); 00270 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00271 // add service 00272 ble.addService(cityRiskService); 00273 ble.gap().setDeviceName((const uint8_t *)"Beacon02"); 00274 // set tx power,valid values are -40, -20, -16, -12, -8, -4, 0, 4 00275 ble.gap().setTxPower(0); 00276 // set adv_interval, in ms 00277 ble.gap().setAdvertisingInterval(100); 00278 // set adv_timeout, in seconds 00279 ble.gap().setAdvertisingTimeout(0); 00280 ble.gap().setScanParams(1000,100,0,false); 00281 beaconInit(); 00282 pc.printf("start\n"); 00283 while (1){ 00284 ble.waitForEvent(); 00285 } 00286 }
Generated on Thu Jul 28 2022 11:01:58 by
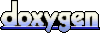