
updated for mbed os 5.4
Fork of Task641 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "string.h" 00004 #include <stdio.h> 00005 #include <ctype.h> 00006 00007 #define SWITCH1_RELEASE 1 00008 00009 void thread1(); 00010 void thread2(); 00011 void switchISR(); 00012 00013 //Analogue inputs 00014 AnalogIn adcIn(A0); 00015 00016 //Digital outputs 00017 DigitalOut onBoardLED(LED1); 00018 DigitalOut redLED(D7); 00019 DigitalOut yellowLED(D6); 00020 DigitalOut greenLED(D5); 00021 00022 //Digital inputs 00023 DigitalIn onBoardSwitch(USER_BUTTON); 00024 DigitalIn sw1(D4); //CONSIDER CHANGING THIS TO AN INTERRUPT 00025 DigitalIn sw2(D3); 00026 00027 //Threads 00028 Thread *t1; 00029 00030 //Class type 00031 class message_t { 00032 public: 00033 float adcValue; 00034 int sw1State; 00035 int sw2State; 00036 00037 //Constructor 00038 message_t(float f, int s1, int s2) { 00039 adcValue = f; 00040 sw1State = s1; 00041 sw2State = s2; 00042 } 00043 }; 00044 00045 //Memory Pool - with capacity for 16 message_t types 00046 MemoryPool<message_t, 16> mpool; 00047 00048 //Message queue - matched to the memory pool 00049 Queue<message_t, 16> queue; 00050 00051 // Call this on precise intervals 00052 void adcISR() { 00053 00054 //Read sample - make a copy 00055 float sample = adcIn; 00056 //Grab switch state 00057 uint32_t switch1State = sw1; 00058 uint32_t switch2State = sw2; 00059 00060 //Allocate a block from the memory pool 00061 message_t *message = mpool.alloc(); 00062 if (message == NULL) { 00063 //Out of memory 00064 redLED = 1; 00065 return; 00066 } 00067 00068 //Fill in the data 00069 message->adcValue = sample; 00070 message->sw1State = switch1State; 00071 message->sw2State = switch2State; 00072 00073 //Write to queue 00074 osStatus stat = queue.put(message); //Note we are sending the "pointer" 00075 00076 //Check if succesful 00077 if (stat == osErrorResource) { 00078 redLED = 1; 00079 printf("queue->put() Error code: %4Xh, Resource not available\r\n", stat); 00080 mpool.free(message); 00081 return; 00082 } 00083 } 00084 00085 //Normal priority thread (consumer) 00086 void thread1() 00087 { 00088 while (true) { 00089 //Block on the queue 00090 osEvent evt = queue.get(); 00091 00092 //Check status 00093 if (evt.status == osEventMessage) { 00094 message_t *pMessage = (message_t*)evt.value.p; //This is the pointer (address) 00095 //Make a copy 00096 message_t msg(pMessage->adcValue, pMessage->sw1State, pMessage->sw2State); 00097 //We are done with this, so give back the memory to the pool 00098 mpool.free(pMessage); 00099 00100 //Echo to the terminal 00101 printf("ADC Value: %.2f\t", msg.adcValue); 00102 printf("SW1: %u\t", msg.sw1State); 00103 printf("SW2: %u\n\r", msg.sw2State); 00104 } 00105 00106 00107 } //end while 00108 } 00109 00110 00111 // Main thread 00112 int main() { 00113 redLED = 0; 00114 yellowLED = 0; 00115 greenLED = 0; 00116 00117 //Start message 00118 printf("Welcome\n"); 00119 00120 //Hook up timer interrupt 00121 Ticker timer; 00122 timer.attach(&adcISR, 0.1); 00123 00124 //Threads 00125 t1 = new Thread(); 00126 t1->start(thread1); 00127 00128 printf("Main Thread\n"); 00129 while (true) { 00130 Thread::wait(5000); 00131 puts("Main Thread Alive"); 00132 } 00133 } 00134 00135
Generated on Wed Jul 13 2022 19:40:36 by
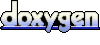