
Coursework template
Dependencies: BMP280 TextLCD BME280
sample_hardware.cpp
00001 #include "mbed.h" 00002 #include "sample_hardware.hpp" 00003 #include "Networkbits.hpp" 00004 00005 #define RED_DONE 1 00006 #define YELLOW_DONE 2 00007 00008 //Digital outputs 00009 DigitalOut onBoardLED(LED1); 00010 DigitalOut redLED(PE_15); 00011 DigitalOut yellowLED(PB_10); 00012 DigitalOut greenLED(PB_11); 00013 00014 //Inputs 00015 DigitalIn onBoardSwitch(USER_BUTTON); 00016 DigitalIn SW1(PE_12); 00017 DigitalIn SW2(PE_14); 00018 //Serial pc(USBTX, USBRX); 00019 AnalogIn adcIn(PA_0); 00020 00021 //Environmental Sensor driver 00022 #ifdef BME 00023 BME280 sensor(D14, D15); 00024 #else 00025 BMP280 sensor(D14, D15); 00026 #endif 00027 00028 //LCD Driver (provided via mbed repository) 00029 //RS D9 00030 //E D8 00031 //D7,6,4,2 are the 4 bit for d4-7 00032 TextLCD lcd(D9, D8, D7, D6, D4, D2); // rs, e, d4-d7 00033 00034 //SD Card 00035 SDBlockDevice sd(PB_5, D12, D13, D10); // mosi, miso, sclk, cs 00036 00037 //POWER ON SELF TEST 00038 void post() 00039 { 00040 //POWER ON TEST (POT) 00041 puts("**********STARTING POWER ON SELF TEST (POST)**********"); 00042 00043 //Test LEDs 00044 puts("ALL LEDs should be blinking"); 00045 for (unsigned int n=0; n<10; n++) { 00046 redLED = 1; 00047 yellowLED = 1; 00048 greenLED = 1; 00049 wait(0.05); 00050 redLED = 0; 00051 yellowLED = 0; 00052 greenLED = 0; 00053 wait(0.05); 00054 } 00055 00056 //Output the switch states (hold them down to test) 00057 printf("SW1: %d\tSW2: %d\n\r", SW1.read(), SW2.read()); 00058 printf("USER: %d\n\r", onBoardSwitch.read()); 00059 00060 //Output the ADC 00061 printf("ADC: %f\n\r", adcIn.read()); 00062 00063 //Read Sensors (I2C) 00064 float temp = sensor.getTemperature(); 00065 float pressure = sensor.getPressure(); 00066 #ifdef BME 00067 float humidity = sensor.getHumidity(); 00068 #endif 00069 00070 //Display in PuTTY 00071 printf("Temperature: %5.1f\n", temp); 00072 printf("Pressure: %5.1f\n", pressure); 00073 #ifdef BME 00074 printf("Pressure: %5.1f\n", humidity); 00075 #endif 00076 00077 //Display on LCD 00078 redLED = 1; 00079 lcd.cls(); 00080 lcd.printf("LCD TEST..."); 00081 wait(0.5); 00082 redLED = 0; 00083 00084 //Network test (if BOTH switches are held down) 00085 networktest(); 00086 00087 puts("**********POST END**********"); 00088 00089 } 00090 00091 void errorCode(ELEC350_ERROR_CODE err) 00092 { 00093 switch (err) { 00094 case OK: 00095 greenLED = 1; 00096 wait(1.0); 00097 greenLED = 0; 00098 return; 00099 case FATAL: 00100 while(1) { 00101 redLED = 1; 00102 wait(0.1); 00103 redLED = 0; 00104 wait(0.1); 00105 } 00106 }; 00107 }
Generated on Fri Jul 15 2022 18:25:32 by
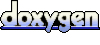