
Coursework template
Dependencies: BMP280 TextLCD BME280
main.cpp
00001 #include "sample_hardware.hpp" 00002 #include "Networkbits.hpp" 00003 00004 // This is a very short demo that demonstrates all the hardware used in the coursework. 00005 // You will need a network connection set up (covered elsewhere). The host PC should have the address 10.0.0.1 00006 00007 //Threads 00008 Thread nwrkThread; 00009 00010 00011 int main() { 00012 //Greeting 00013 printf("Testing\n\n"); 00014 00015 //Power on self test 00016 post(); 00017 00018 //Initialise the SD card (this needs to move) 00019 if ( sd.init() != 0) { 00020 printf("Init failed \n"); 00021 lcd.cls(); 00022 lcd.printf("CANNOT INIT SD"); 00023 errorCode(FATAL); 00024 } 00025 00026 //Create a filing system for SD Card 00027 FATFileSystem fs("sd", &sd); 00028 00029 //Open to WRITE 00030 FILE* fp = fopen("/sd/test.csv","a"); 00031 if (fp == NULL) { 00032 error("Could not open file for write\n"); 00033 lcd.cls(); 00034 lcd.printf("CANNOT OPEN FILE\n\n"); 00035 errorCode(FATAL); 00036 } 00037 00038 //Last message before sampling begins 00039 lcd.cls(); 00040 lcd.printf("READY\n\n"); 00041 00042 00043 //Press either switch to unmount 00044 while ((SW1 == 0) && (SW2 == 0)) { 00045 00046 //Base loop delay 00047 wait(1.0); 00048 00049 //Read environmental sensors 00050 double temp = sensor.getTemperature(); 00051 double pressure = sensor.getPressure(); 00052 00053 //Write new data to LCD (not fast!) 00054 lcd.cls(); 00055 lcd.printf("Temp Pressure\n"); 00056 lcd.printf("%6.1f ",temp); 00057 lcd.printf("%.2f\n",pressure); 00058 00059 //Write to SD (potentially slow) 00060 fprintf(fp, "%6.1f,%.2f\n\r", temp, pressure); 00061 } 00062 00063 //Close File 00064 fclose(fp); 00065 00066 //Close down 00067 sd.deinit(); 00068 printf("Unmounted...\n"); 00069 lcd.cls(); 00070 lcd.printf("Unmounted...\n\n"); 00071 00072 //Flash to indicate goodness 00073 while(true) { 00074 greenLED = 1; 00075 wait(0.5); 00076 greenLED = 0; 00077 wait(0.1); 00078 } 00079 } 00080 00081 00082
Generated on Fri Jul 15 2022 18:25:32 by
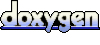