
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ClockControl/ClockControl.h" 00003 #include "CoreMark/core_portme.h" 00004 00005 int main() { 00006 while(1) { 00007 00008 00009 printf("mbed Clock Control test\r\n"); 00010 printf("(c) 2010 Michael Wei\r\n"); 00011 00012 unsigned short newM = 12; 00013 unsigned short newN = 1; 00014 00015 printf("Value of M? (default = 12):\r\n"); 00016 scanf("%d", &newM); 00017 00018 printf("Value of N? (default = 1):\r\n"); 00019 scanf("%d", &newN); 00020 00021 setSystemFrequency(0x3, 0x1, newM, newN); 00022 00023 Serial pc(USBTX, USBRX); // tx, rx 00024 00025 //This code by Simon Ford: http://mbed.org/forum/mbed/topic/229/?page=1#comment-1225 00026 00027 int Fin = 12000000; // 12MHz XTAL 00028 pc.printf("PLL Registers:\r\n"); 00029 pc.printf(" - PLL0CFG = 0x%08X\r\n", LPC_SC->PLL0CFG); 00030 pc.printf(" - CLKCFG = 0x%08X\r\n", LPC_SC->CCLKCFG); 00031 00032 int M = (LPC_SC->PLL0CFG & 0xFFFF) + 1; 00033 int N = (LPC_SC->PLL0CFG >> 16) + 1; 00034 int CCLKDIV = LPC_SC->CCLKCFG + 1; 00035 00036 pc.printf("Clock Variables:\r\n"); 00037 pc.printf(" - Fin = %d\r\n", Fin); 00038 pc.printf(" - M = %d\r\n", M); 00039 pc.printf(" - N = %d\r\n", N); 00040 pc.printf(" - CCLKDIV = %d\r\n", CCLKDIV); 00041 00042 int Fcco = (2 * M * 12000000) / N; 00043 int CCLK = Fcco / CCLKDIV; 00044 00045 pc.printf("Clock Results:\r\n"); 00046 pc.printf(" - Fcco = %d\r\n", Fcco); 00047 00048 printf(" - CCLK = %d\r\n", CCLK); 00049 00050 printf("Run CoreMark? (Y/N)\n"); 00051 char buf[8]; 00052 scanf("%s", buf); 00053 if (buf[0] == 'y' || buf[0] == 'Y') 00054 { 00055 mainCoreMark(); 00056 } 00057 00058 wait(1); 00059 NVIC_SystemReset(); 00060 } 00061 }
Generated on Fri Jul 15 2022 12:36:20 by
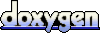