
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
cvt.c
00001 #include <math.h> 00002 #define CVTBUFSIZE 80 00003 static char CVTBUF[CVTBUFSIZE]; 00004 00005 static char *cvt(double arg, int ndigits, int *decpt, int *sign, char *buf, int eflag) 00006 { 00007 int r2; 00008 double fi, fj; 00009 char *p, *p1; 00010 00011 if (ndigits < 0) ndigits = 0; 00012 if (ndigits >= CVTBUFSIZE - 1) ndigits = CVTBUFSIZE - 2; 00013 r2 = 0; 00014 *sign = 0; 00015 p = &buf[0]; 00016 if (arg < 0) 00017 { 00018 *sign = 1; 00019 arg = -arg; 00020 } 00021 arg = modf(arg, &fi); 00022 p1 = &buf[CVTBUFSIZE]; 00023 00024 if (fi != 0) 00025 { 00026 p1 = &buf[CVTBUFSIZE]; 00027 while (fi != 0) 00028 { 00029 fj = modf(fi / 10, &fi); 00030 *--p1 = (int)((fj + .03) * 10) + '0'; 00031 r2++; 00032 } 00033 while (p1 < &buf[CVTBUFSIZE]) *p++ = *p1++; 00034 } 00035 else if (arg > 0) 00036 { 00037 while ((fj = arg * 10) < 1) 00038 { 00039 arg = fj; 00040 r2--; 00041 } 00042 } 00043 p1 = &buf[ndigits]; 00044 if (eflag == 0) p1 += r2; 00045 *decpt = r2; 00046 if (p1 < &buf[0]) 00047 { 00048 buf[0] = '\0'; 00049 return buf; 00050 } 00051 while (p <= p1 && p < &buf[CVTBUFSIZE]) 00052 { 00053 arg *= 10; 00054 arg = modf(arg, &fj); 00055 *p++ = (int) fj + '0'; 00056 } 00057 if (p1 >= &buf[CVTBUFSIZE]) 00058 { 00059 buf[CVTBUFSIZE - 1] = '\0'; 00060 return buf; 00061 } 00062 p = p1; 00063 *p1 += 5; 00064 while (*p1 > '9') 00065 { 00066 *p1 = '0'; 00067 if (p1 > buf) 00068 ++*--p1; 00069 else 00070 { 00071 *p1 = '1'; 00072 (*decpt)++; 00073 if (eflag == 0) 00074 { 00075 if (p > buf) *p = '0'; 00076 p++; 00077 } 00078 } 00079 } 00080 *p = '\0'; 00081 return buf; 00082 } 00083 00084 char *ecvt(double arg, int ndigits, int *decpt, int *sign) 00085 { 00086 return cvt(arg, ndigits, decpt, sign, CVTBUF, 1); 00087 } 00088 00089 char *ecvtbuf(double arg, int ndigits, int *decpt, int *sign, char *buf) 00090 { 00091 return cvt(arg, ndigits, decpt, sign, buf, 1); 00092 } 00093 00094 char *fcvt(double arg, int ndigits, int *decpt, int *sign) 00095 { 00096 return cvt(arg, ndigits, decpt, sign, CVTBUF, 0); 00097 } 00098 00099 char *fcvtbuf(double arg, int ndigits, int *decpt, int *sign, char *buf) 00100 { 00101 return cvt(arg, ndigits, decpt, sign, buf, 0); 00102 }
Generated on Fri Jul 15 2022 12:36:20 by
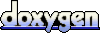