
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
core_util.c
00001 /* 00002 Author : Shay Gal-On, EEMBC 00003 00004 This file is part of EEMBC(R) and CoreMark(TM), which are Copyright (C) 2009 00005 All rights reserved. 00006 00007 EEMBC CoreMark Software is a product of EEMBC and is provided under the terms of the 00008 CoreMark License that is distributed with the official EEMBC COREMARK Software release. 00009 If you received this EEMBC CoreMark Software without the accompanying CoreMark License, 00010 you must discontinue use and download the official release from www.coremark.org. 00011 00012 Also, if you are publicly displaying scores generated from the EEMBC CoreMark software, 00013 make sure that you are in compliance with Run and Reporting rules specified in the accompanying readme.txt file. 00014 00015 EEMBC 00016 4354 Town Center Blvd. Suite 114-200 00017 El Dorado Hills, CA, 95762 00018 */ 00019 #include "coremark.h" 00020 /* Function: get_seed 00021 Get a values that cannot be determined at compile time. 00022 00023 Since different embedded systems and compilers are used, 3 different methods are provided: 00024 1 - Using a volatile variable. This method is only valid if the compiler is forced to generate code that 00025 reads the value of a volatile variable from memory at run time. 00026 Please note, if using this method, you would need to modify core_portme.c to generate training profile. 00027 2 - Command line arguments. This is the preferred method if command line arguments are supported. 00028 3 - System function. If none of the first 2 methods is available on the platform, 00029 a system function which is not a stub can be used. 00030 00031 e.g. read the value on GPIO pins connected to switches, or invoke special simulator functions. 00032 */ 00033 #if (SEED_METHOD==SEED_VOLATILE) 00034 extern volatile ee_s32 seed1_volatile; 00035 extern volatile ee_s32 seed2_volatile; 00036 extern volatile ee_s32 seed3_volatile; 00037 extern volatile ee_s32 seed4_volatile; 00038 extern volatile ee_s32 seed5_volatile; 00039 ee_s32 get_seed_32(int i) { 00040 ee_s32 retval; 00041 switch (i) { 00042 case 1: 00043 retval=seed1_volatile; 00044 break; 00045 case 2: 00046 retval=seed2_volatile; 00047 break; 00048 case 3: 00049 retval=seed3_volatile; 00050 break; 00051 case 4: 00052 retval=seed4_volatile; 00053 break; 00054 case 5: 00055 retval=seed5_volatile; 00056 break; 00057 default: 00058 retval=0; 00059 break; 00060 } 00061 return retval; 00062 } 00063 #elif (SEED_METHOD==SEED_ARG) 00064 ee_s32 parseval(char *valstring) { 00065 ee_s32 retval=0; 00066 ee_s32 neg=1; 00067 int hexmode=0; 00068 if (*valstring == '-') { 00069 neg=-1; 00070 valstring++; 00071 } 00072 if ((valstring[0] == '0') && (valstring[1] == 'x')) { 00073 hexmode=1; 00074 valstring+=2; 00075 } 00076 /* first look for digits */ 00077 if (hexmode) { 00078 while (((*valstring >= '0') && (*valstring <= '9')) || ((*valstring >= 'a') && (*valstring <= 'f'))) { 00079 ee_s32 digit=*valstring-'0'; 00080 if (digit>9) 00081 digit=10+*valstring-'a'; 00082 retval*=16; 00083 retval+=digit; 00084 valstring++; 00085 } 00086 } else { 00087 while ((*valstring >= '0') && (*valstring <= '9')) { 00088 ee_s32 digit=*valstring-'0'; 00089 retval*=10; 00090 retval+=digit; 00091 valstring++; 00092 } 00093 } 00094 /* now add qualifiers */ 00095 if (*valstring=='K') 00096 retval*=1024; 00097 if (*valstring=='M') 00098 retval*=1024*1024; 00099 00100 retval*=neg; 00101 return retval; 00102 } 00103 00104 ee_s32 get_seed_args(int i, int argc, char *argv[]) { 00105 if (argc>i) 00106 return parseval(argv[i]); 00107 return 0; 00108 } 00109 00110 #elif (SEED_METHOD==SEED_FUNC) 00111 /* If using OS based function, you must define and implement the functions below in core_portme.h and core_portme.c ! */ 00112 ee_s32 get_seed_32(int i) { 00113 ee_s32 retval; 00114 switch (i) { 00115 case 1: 00116 retval=portme_sys1(); 00117 break; 00118 case 2: 00119 retval=portme_sys2(); 00120 break; 00121 case 3: 00122 retval=portme_sys3(); 00123 break; 00124 case 4: 00125 retval=portme_sys4(); 00126 break; 00127 case 5: 00128 retval=portme_sys5(); 00129 break; 00130 default: 00131 retval=0; 00132 break; 00133 } 00134 return retval; 00135 } 00136 #endif 00137 00138 /* Function: crc* 00139 Service functions to calculate 16b CRC code. 00140 00141 */ 00142 ee_u16 crcu8(ee_u8 data, ee_u16 crc ) 00143 { 00144 ee_u8 i=0,x16=0,carry=0; 00145 00146 for (i = 0; i < 8; i++) 00147 { 00148 x16 = (ee_u8)((data & 1) ^ ((ee_u8)crc & 1)); 00149 data >>= 1; 00150 00151 if (x16 == 1) 00152 { 00153 crc ^= 0x4002; 00154 carry = 1; 00155 } 00156 else 00157 carry = 0; 00158 crc >>= 1; 00159 if (carry) 00160 crc |= 0x8000; 00161 else 00162 crc &= 0x7fff; 00163 } 00164 return crc; 00165 } 00166 ee_u16 crcu16(ee_u16 newval, ee_u16 crc) { 00167 crc=crcu8( (ee_u8) (newval) ,crc); 00168 crc=crcu8( (ee_u8) ((newval)>>8) ,crc); 00169 return crc; 00170 } 00171 ee_u16 crcu32(ee_u32 newval, ee_u16 crc) { 00172 crc=crc16((ee_s16) newval ,crc); 00173 crc=crc16((ee_s16) (newval>>16) ,crc); 00174 return crc; 00175 } 00176 ee_u16 crc16(ee_s16 newval, ee_u16 crc) { 00177 return crcu16((ee_u16)newval, crc); 00178 } 00179 00180 ee_u8 check_data_types() { 00181 ee_u8 retval=0; 00182 if (sizeof(ee_u8) != 1) { 00183 ee_printf("ERROR: ee_u8 is not an 8b datatype!\n"); 00184 retval++; 00185 } 00186 if (sizeof(ee_u16) != 2) { 00187 ee_printf("ERROR: ee_u16 is not a 16b datatype!\n"); 00188 retval++; 00189 } 00190 if (sizeof(ee_s16) != 2) { 00191 ee_printf("ERROR: ee_s16 is not a 16b datatype!\n"); 00192 retval++; 00193 } 00194 if (sizeof(ee_s32) != 4) { 00195 ee_printf("ERROR: ee_s32 is not a 32b datatype!\n"); 00196 retval++; 00197 } 00198 if (sizeof(ee_u32) != 4) { 00199 ee_printf("ERROR: ee_u32 is not a 32b datatype!\n"); 00200 retval++; 00201 } 00202 if (sizeof(ee_ptr_int) != sizeof(int *)) { 00203 ee_printf("ERROR: ee_ptr_int is not a datatype that holds an int pointer!\n"); 00204 retval++; 00205 } 00206 if (retval>0) { 00207 ee_printf("ERROR: Please modify the datatypes in core_portme.h!\n"); 00208 } 00209 return retval; 00210 }
Generated on Fri Jul 15 2022 12:36:20 by
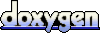