
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
core_portme.c
00001 /* 00002 File : core_portme.c 00003 */ 00004 /* 00005 Author : Shay Gal-On, EEMBC 00006 Legal : TODO! 00007 */ 00008 #include "coremark.h" 00009 #include "core_portme.h" 00010 #include "mbed.h" 00011 00012 00013 #if VALIDATION_RUN 00014 volatile ee_s32 seed1_volatile=0x3415; 00015 volatile ee_s32 seed2_volatile=0x3415; 00016 volatile ee_s32 seed3_volatile=0x66; 00017 #endif 00018 #if PERFORMANCE_RUN 00019 volatile ee_s32 seed1_volatile=0x0; 00020 volatile ee_s32 seed2_volatile=0x0; 00021 volatile ee_s32 seed3_volatile=0x66; 00022 #endif 00023 #if PROFILE_RUN 00024 volatile ee_s32 seed1_volatile=0x8; 00025 volatile ee_s32 seed2_volatile=0x8; 00026 volatile ee_s32 seed3_volatile=0x8; 00027 #endif 00028 volatile ee_s32 seed4_volatile=ITERATIONS; 00029 volatile ee_s32 seed5_volatile=0; 00030 /* Porting : Timing functions 00031 How to capture time and convert to seconds must be ported to whatever is supported by the platform. 00032 e.g. Read value from on board RTC, read value from cpu clock cycles performance counter etc. 00033 Sample implementation for standard time.h and windows.h definitions included. 00034 */ 00035 00036 CORETIMETYPE barebones_clock() { 00037 return clock(); 00038 } 00039 /* Define : TIMER_RES_DIVIDER 00040 Divider to trade off timer resolution and total time that can be measured. 00041 00042 Use lower values to increase resolution, but make sure that overflow does not occur. 00043 If there are issues with the return value overflowing, increase this value. 00044 */ 00045 #define GETMYTIME(_t) (*_t=barebones_clock()) 00046 #define MYTIMEDIFF(fin,ini) ((fin)-(ini)) 00047 #define TIMER_RES_DIVIDER 1 00048 #define SAMPLE_TIME_IMPLEMENTATION 1 00049 #define EE_TICKS_PER_SEC (CLOCKS_PER_SEC / TIMER_RES_DIVIDER) 00050 00051 /** Define Host specific (POSIX), or target specific global time variables. */ 00052 static CORETIMETYPE start_time_val, stop_time_val; 00053 00054 /* Function : start_time 00055 This function will be called right before starting the timed portion of the benchmark. 00056 00057 Implementation may be capturing a system timer (as implemented in the example code) 00058 or zeroing some system parameters - e.g. setting the cpu clocks cycles to 0. 00059 */ 00060 void start_time(void) { 00061 GETMYTIME(&start_time_val ); 00062 } 00063 /* Function : stop_time 00064 This function will be called right after ending the timed portion of the benchmark. 00065 00066 Implementation may be capturing a system timer (as implemented in the example code) 00067 or other system parameters - e.g. reading the current value of cpu cycles counter. 00068 */ 00069 void stop_time(void) { 00070 GETMYTIME(&stop_time_val ); 00071 } 00072 /* Function : get_time 00073 Return an abstract "ticks" number that signifies time on the system. 00074 00075 Actual value returned may be cpu cycles, milliseconds or any other value, 00076 as long as it can be converted to seconds by <time_in_secs>. 00077 This methodology is taken to accomodate any hardware or simulated platform. 00078 The sample implementation returns millisecs by default, 00079 and the resolution is controlled by <TIMER_RES_DIVIDER> 00080 */ 00081 CORE_TICKS get_time(void) { 00082 CORE_TICKS elapsed=(CORE_TICKS)(MYTIMEDIFF(stop_time_val, start_time_val)); 00083 return elapsed; 00084 } 00085 /* Function : time_in_secs 00086 Convert the value returned by get_time to seconds. 00087 00088 The <secs_ret> type is used to accomodate systems with no support for floating point. 00089 Default implementation implemented by the EE_TICKS_PER_SEC macro above. 00090 */ 00091 secs_ret time_in_secs(CORE_TICKS ticks) { 00092 secs_ret retval=((secs_ret)ticks) / (secs_ret)EE_TICKS_PER_SEC; 00093 return retval; 00094 } 00095 00096 ee_u32 default_num_contexts=1; 00097 00098 /* Function : portable_init 00099 Target specific initialization code 00100 Test for some common mistakes. 00101 */ 00102 void portable_init(core_portable *p, int *argc, char *argv[]) 00103 { 00104 //#error "Call board initialization routines in portable init (if needed), in particular initialize UART!\n" 00105 if (sizeof(ee_ptr_int) != sizeof(ee_u8 *)) { 00106 ee_printf("ERROR! Please define ee_ptr_int to a type that holds a pointer!\n"); 00107 } 00108 if (sizeof(ee_u32) != 4) { 00109 ee_printf("ERROR! Please define ee_u32 to a 32b unsigned type!\n"); 00110 } 00111 p->portable_id=1; 00112 } 00113 /* Function : portable_fini 00114 Target specific final code 00115 */ 00116 void portable_fini(core_portable *p) 00117 { 00118 p->portable_id=0; 00119 } 00120 00121
Generated on Fri Jul 15 2022 12:36:20 by
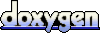