
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
ClockControl.cpp
00001 #include "ClockControl.h" 00002 00003 void setPLL0Frequency(unsigned char clkSrc, unsigned short M, unsigned char N) 00004 { 00005 LPC_SC->CLKSRCSEL = clkSrc; 00006 LPC_SC->PLL0CFG = (((unsigned int)N-1) << 16) | M-1; 00007 LPC_SC->PLL0CON = 0x01; 00008 LPC_SC->PLL0FEED = 0xAA; 00009 LPC_SC->PLL0FEED = 0x55; 00010 while (!(LPC_SC->PLL0STAT & (1<<26))); 00011 00012 LPC_SC->PLL0CON = 0x03; 00013 LPC_SC->PLL0FEED = 0xAA; 00014 LPC_SC->PLL0FEED = 0x55; 00015 } 00016 00017 void setPLL1Frequency(unsigned char clkSrc, unsigned short M, unsigned char N) 00018 { 00019 LPC_SC->CLKSRCSEL = clkSrc; 00020 LPC_SC->PLL1CFG = (((unsigned int)N-1) << 16) | M-1; 00021 LPC_SC->PLL1CON = 0x01; 00022 LPC_SC->PLL1FEED = 0xAA; 00023 LPC_SC->PLL1FEED = 0x55; 00024 while (!(LPC_SC->PLL1STAT & (1<<26))); 00025 00026 LPC_SC->PLL1CON = 0x03; 00027 LPC_SC->PLL1FEED = 0xAA; 00028 LPC_SC->PLL1FEED = 0x55; 00029 } 00030 00031 unsigned int setSystemFrequency(unsigned char clkDivider, unsigned char clkSrc, unsigned short M, unsigned char N) 00032 { 00033 setPLL0Frequency(clkSrc, M, N); 00034 LPC_SC->CCLKCFG = clkDivider - 1; 00035 SystemCoreClockUpdate(); 00036 return SystemCoreClock; 00037 }
Generated on Fri Jul 15 2022 12:36:20 by
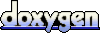