
motor spins
Fork of analoghalls5 by
Embed:
(wiki syntax)
Show/hide line numbers
statusupdater.cpp
00001 #include "includes.h" 00002 #include "core.h" 00003 #include "sensors.h" 00004 #include "meta.h" 00005 #include "lut.h" 00006 00007 unsigned long StatusUpdater::_time; 00008 00009 StatusUpdater::StatusUpdater(Inverter *inverter, Motor *motor, User *user) { 00010 _inverter = inverter; 00011 _motor = motor; 00012 _user = user; 00013 _fast_sample_rate = 5000; 00014 _slow_sample_rate = 10; 00015 00016 _time_ticker.attach_us(&time_upd_isr, 50); 00017 } 00018 00019 void StatusUpdater::Config(int fast_sample_rate, int slow_sample_rate) { 00020 _fast_sample_rate = fast_sample_rate; 00021 _slow_sample_rate = slow_sample_rate; 00022 } 00023 00024 void StatusUpdater::time_upd_isr() { 00025 _time+= 50; 00026 } 00027 00028 float StatusUpdater::LutSin(float theta) { 00029 if (theta < 0.0f) theta += 360.0f; 00030 if (theta >= 360.0f) theta -= 360.0f; 00031 return sinetab[(int) theta]; 00032 } 00033 00034 float StatusUpdater::LutCos(float theta) { 00035 return LutSin(90.0f - theta); 00036 } 00037 00038 void StatusUpdater::Start() { 00039 _time = 0; 00040 int fast_us = 1000000 / _fast_sample_rate; 00041 int slow_us = 1000000 / _slow_sample_rate; 00042 00043 int last_fast = 0; 00044 int last_slow = 0; 00045 00046 for(;;) { 00047 if (_time - last_fast > fast_us) { 00048 00049 _motor->UpdateState(); 00050 _inverter->UpdateVbus(); 00051 last_fast = _time; 00052 00053 pc->printf("%f %f %f %f %f\n\r", _motor->angle, _motor->I_c, _motor->I_b, test_alpha, test_beta); 00054 //pc->printf("%f %f %f %f\n\r", _motor->angle); 00055 00056 } 00057 00058 if (_time - last_slow > slow_us) { 00059 00060 _user->UpdateState(); 00061 _motor->UpdateTemp(); 00062 _inverter->UpdateTemp(); 00063 last_slow = _time; 00064 00065 //pc->printf("%f %f\n\r", _inverter->va, _inverter->vb); 00066 } 00067 } 00068 }
Generated on Fri Jul 15 2022 12:15:05 by
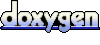