
motor spins
Fork of analoghalls5 by
Embed:
(wiki syntax)
Show/hide line numbers
meta.h
00001 #ifndef __META_H 00002 #define __META_H 00003 00004 #include "includes.h" 00005 #include "core.h" 00006 #include "sensors.h" 00007 00008 class Modulator { 00009 public: 00010 Modulator(Inverter *inverter) {_inverter = inverter;} 00011 virtual void Update(float va, float vb) = 0; 00012 protected: 00013 Inverter* _inverter; 00014 }; 00015 00016 class SinusoidalModulator: public Modulator { 00017 public: 00018 SinusoidalModulator(Inverter *inverter):Modulator(inverter) {} 00019 virtual void Update(float va, float vb); 00020 }; 00021 00022 class PidController { 00023 public: 00024 PidController(float ki, float kp, float kd, float out_max, float out_min); 00025 float Update(float ref, float in); 00026 private: 00027 float _ki, _kp, _kd; 00028 float _last_in, _integral; 00029 float _out_max, _out_min; 00030 }; 00031 00032 class ReferenceSynthesizer { 00033 public: 00034 ReferenceSynthesizer(float max_phase_current) {_max_phase_current = max_phase_current;} 00035 virtual void GetReference(float angle, float *ref_d, float *ref_q) {*ref_d = 0; *ref_q = 0;} 00036 protected: 00037 static float LutSin(float theta); 00038 static float LutCos(float theta); 00039 protected: 00040 float _max_phase_current; 00041 }; 00042 00043 class SynchronousReferenceSynthesizer : public ReferenceSynthesizer { 00044 public: 00045 SynchronousReferenceSynthesizer(float max_phase_current):ReferenceSynthesizer(max_phase_current) {} 00046 virtual void GetReference(float angle, float *ref_d, float *ref_q); 00047 }; 00048 00049 class StatusUpdater { 00050 public: 00051 StatusUpdater(Inverter *inverter, Motor *motor, User *user); 00052 void Config(int fast_sample_rate, int slow_sample_rate); 00053 void Start(); 00054 private: 00055 static void time_upd_isr(); 00056 static float LutSin(float theta); 00057 static float LutCos(float theta); 00058 private: 00059 Inverter *_inverter; 00060 Motor *_motor; 00061 User *_user; 00062 int _fast_sample_rate; 00063 int _slow_sample_rate; 00064 00065 static unsigned long _time; 00066 Ticker _time_ticker; 00067 }; 00068 00069 class LoopDriver { 00070 public: 00071 LoopDriver(Inverter *inverter, Motor *motor, User *user, PidController *pid_d, PidController *pid_q, 00072 Modulator *modulator, float max_phase_current, int update_frequency); 00073 void Start(); 00074 private: 00075 static void Clarke(float a, float b, float *alpha, float *beta); 00076 static void Parke(float alpha, float beta, float theta, float *d, float *q); 00077 static void InverseParke(float d, float q, float theta, float *alpha, float *beta); 00078 private: 00079 static void update(); 00080 private: 00081 static float LutSin(float theta); 00082 static float LutCos(float theta); 00083 private: 00084 static Inverter *_inverter; 00085 static Motor *_motor; 00086 static User *_user; 00087 static PidController *_pid_d, *_pid_q; 00088 static ReferenceSynthesizer *_reference; 00089 static Modulator *_modulator; 00090 00091 Ticker _upd_ticker; 00092 float _max_phase_current; 00093 int _update_frequency; 00094 static int _blinky_toggle; 00095 }; 00096 00097 #endif 00098
Generated on Fri Jul 15 2022 12:15:05 by
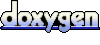