Version 1.0 - Library for Parallax OFN module 27903
Fork of OFN by
ofn.h
00001 00002 //@Authors: Guru Das Srinagesh, Neha Kadam 00003 /* Copyright (c) March, 2015 MIT License 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00008 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in all copies or 00012 * substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00015 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00016 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00017 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00019 */ 00020 00021 00022 /* 00023 * Library for Parallax Optical Finger Navigation Module 27903 00024 * Link to datasheet: http://www.parallax.com/sites/default/files/downloads/27903-OFN-Module-Documentation-v1.1.pdf 00025 * 00026 */ 00027 #ifndef OFN_H 00028 #define OFN_H 00029 00030 #include "mbed.h" 00031 00032 #define DEVICE_ADDR 0x33 00033 #define PRODUCT_ID_ADDR 0x00 00034 #define REVISION_ID_ADDR 0x01 00035 00036 #define SOFT_RESET 0x3A 00037 #define OFN_ORIENTATION_CTRL 0x77 00038 00039 #define ORIENT_DEFAULT 1 00040 #define ORIENT_ROTATED_CLKWISE 0 00041 /** 00042 * Class for the Parallax OFN module 27903 00043 * 00044 */ 00045 class OFN { 00046 00047 public: 00048 00049 /** 00050 * Constructor. 00051 * 00052 * @param sda mbed pin to use for SDA line of I2C interface. 00053 * @param scl mbed pin to use for SCL line of I2C interface. 00054 */ 00055 OFN(PinName sda, PinName scl); 00056 00057 /** 00058 * 00059 * Function to check if motion has been detected 00060 * Checks the 7th bit of MOTION register and 00061 * Returns true if motion detected else returns false 00062 */ 00063 bool checkMotion(); 00064 00065 /** 00066 * 00067 * Function to get displacement in the X direction 00068 * Returns an integer value 00069 */ 00070 int readX(); 00071 00072 /** 00073 * 00074 * Function to get displacement in the X direction 00075 * Returns an integer value 00076 */ 00077 int readY(); 00078 00079 /** 00080 * 00081 * Function to read the Orientation register 00082 * Returns an integer value 00083 * 1 - default orientation 00084 * 0 - rotated by 90 degrees 00085 */ 00086 int readOrientation(); 00087 00088 private: 00089 00090 I2C i2c; 00091 00092 char MOTREG_ADDR; // To contain address of Motion Register 00093 char DELTA_X_ADDR; // To contain address of Delta_X Register 00094 char DELTA_Y_ADDR; // To contain address of Delta_Y Register 00095 int MotReg; // To contain value of Motion Register 00096 bool moved; // To contain value of state of Motion 00097 00098 char raw_delta_X[1]; // To contain raw sensor reading of Delta_X 00099 int delta_X; // To contain correct reading of Delta_X 00100 00101 char raw_delta_Y[1]; // To contain raw sensor reading of Delta_Y 00102 int delta_Y; // To contain correct reading of Delta_Y 00103 }; 00104 00105 #endif /* OFN_H */
Generated on Tue Jul 12 2022 19:51:47 by
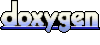