Dependents: ADXL345 TCPspittertobi ADXL345 RS485R_2 ... more
ADXL345_I2C.h
00001 /** 00002 * @author Jose R Padron 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ADXL345, triple axis, digital interface, accelerometer. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.analog.com/static/imported-files/data_sheets/ADXL345.pdf 00033 * 00034 * Things to know: SDO pin must be tied high for address 0x1D or low for address 0x53. 00035 * It cannot be left floating. 00036 00037 */ 00038 00039 #ifndef ADXL345_I2C_H 00040 #define ADXL345_I2C_H 00041 00042 /** 00043 * Includes 00044 */ 00045 #include "mbed.h" 00046 00047 /** 00048 * Defines 00049 */ 00050 //Registers. 00051 #define ADXL345_DEVID_REG 0x00 00052 #define ADXL345_THRESH_TAP_REG 0x1D 00053 #define ADXL345_OFSX_REG 0x1E 00054 #define ADXL345_OFSY_REG 0x1F 00055 #define ADXL345_OFSZ_REG 0x20 00056 #define ADXL345_DUR_REG 0x21 00057 #define ADXL345_LATENT_REG 0x22 00058 #define ADXL345_WINDOW_REG 0x23 00059 #define ADXL345_THRESH_ACT_REG 0x24 00060 #define ADXL345_THRESH_INACT_REG 0x25 00061 #define ADXL345_TIME_INACT_REG 0x26 00062 #define ADXL345_ACT_INACT_CTL_REG 0x27 00063 #define ADXL345_THRESH_FF_REG 0x28 00064 #define ADXL345_TIME_FF_REG 0x29 00065 #define ADXL345_TAP_AXES_REG 0x2A 00066 #define ADXL345_ACT_TAP_STATUS_REG 0x2B 00067 #define ADXL345_BW_RATE_REG 0x2C 00068 #define ADXL345_POWER_CTL_REG 0x2D 00069 #define ADXL345_INT_ENABLE_REG 0x2E 00070 #define ADXL345_INT_MAP_REG 0x2F 00071 #define ADXL345_INT_SOURCE_REG 0x30 00072 #define ADXL345_DATA_FORMAT_REG 0x31 00073 #define ADXL345_DATAX0_REG 0x32 00074 #define ADXL345_DATAX1_REG 0x33 00075 #define ADXL345_DATAY0_REG 0x34 00076 #define ADXL345_DATAY1_REG 0x35 00077 #define ADXL345_DATAZ0_REG 0x36 00078 #define ADXL345_DATAZ1_REG 0x37 00079 #define ADXL345_FIFO_CTL 0x38 00080 #define ADXL345_FIFO_STATUS 0x39 00081 00082 //Data rate codes. 00083 #define ADXL345_3200HZ 0x0F 00084 #define ADXL345_1600HZ 0x0E 00085 #define ADXL345_800HZ 0x0D 00086 #define ADXL345_400HZ 0x0C 00087 #define ADXL345_200HZ 0x0B 00088 #define ADXL345_100HZ 0x0A 00089 #define ADXL345_50HZ 0x09 00090 #define ADXL345_25HZ 0x08 00091 #define ADXL345_12HZ5 0x07 00092 #define ADXL345_6HZ25 0x06 00093 00094 #define ADXL345_ID 0x1D 00095 #define ADXL345_I2C_READ 0x3B 00096 #define ADXL345_I2C_WRITE 0x3A 00097 #define ADXL345_MULTI_BYTE 0x60 00098 00099 #define ADXL345_X 0x00 00100 #define ADXL345_Y 0x01 00101 #define ADXL345_Z 0x02 00102 00103 /** 00104 * ADXL345 triple axis, digital interface, accelerometer. 00105 */ 00106 class ADXL345_I2C { 00107 00108 public: 00109 00110 /** 00111 * Constructor. 00112 * 00113 * @param sda mbed pin to use for sda line of I2C interface. 00114 * @param scl mbed pin to use for SCL line of I2C interface. 00115 * @param cs mbed pin to use for not chip select line of I2C interface. 00116 */ 00117 ADXL345_I2C(PinName sda, PinName scl); 00118 00119 /** 00120 * Read the device ID register on the device. 00121 * 00122 * @return The device ID code 00123 */ 00124 char getDevId(void); 00125 00126 /** 00127 * Read the tap threshold on the device. 00128 * 00129 * @return The tap threshold as an 8-bit number with a scale factor of 00130 * 62.5mg/LSB. 00131 */ 00132 int getTapThreshold(void); 00133 00134 /** 00135 * Set the tap threshold. 00136 * 00137 * @param The tap threshold as an 8-bit number with a scale factor of 00138 * 62.5mg/LSB. 00139 */ 00140 void setTapThreshold(int threshold); 00141 00142 /** 00143 * Get the current offset for a particular axis. 00144 * 00145 * @param axis 0x00 -> X-axis 00146 * 0x01 -> Y-axis 00147 * 0x02 -> Z-axis 00148 * @return The current offset as an 8-bit 2's complement number with scale 00149 * factor 15.6mg/LSB. 00150 */ 00151 int getOffset(int axis); 00152 00153 /** 00154 * Set the offset for a particular axis. 00155 * 00156 * @param axis 0x00 -> X-axis 00157 * 0x01 -> Y-axis 00158 * 0x02 -> Z-axis 00159 * @param offset The offset as an 8-bit 2's complement number with scale 00160 * factor 15.6mg/LSB. 00161 */ 00162 void setOffset(int axis, char offset); 00163 00164 /** 00165 * Get the tap duration required to trigger an event. 00166 * 00167 * @return The max time that an event must be above the tap threshold to 00168 * qualify as a tap event, in microseconds. 00169 */ 00170 int getTapDuration(void); 00171 00172 /** 00173 * Set the tap duration required to trigger an event. 00174 * 00175 * @param duration_us The max time that an event must be above the tap 00176 * threshold to qualify as a tap event, in microseconds. 00177 * Time will be normalized by the scale factor which is 00178 * 625us/LSB. A value of 0 disables the single/double 00179 * tap functions. 00180 */ 00181 void setTapDuration(int duration_us); 00182 00183 /** 00184 * Get the tap latency between the detection of a tap and the time window. 00185 * 00186 * @return The wait time from the detection of a tap event to the start of 00187 * the time window during which a possible second tap event can be 00188 * detected in milliseconds. 00189 */ 00190 float getTapLatency(void); 00191 00192 /** 00193 * Set the tap latency between the detection of a tap and the time window. 00194 * 00195 * @param latency_ms The wait time from the detection of a tap event to the 00196 * start of the time window during which a possible 00197 * second tap event can be detected in milliseconds. 00198 * A value of 0 disables the double tap function. 00199 */ 00200 void setTapLatency(int latency_ms); 00201 00202 /** 00203 * Get the time of window between tap latency and a double tap. 00204 * 00205 * @return The amount of time after the expiration of the latency time 00206 * during which a second valid tap can begin, in milliseconds. 00207 */ 00208 float getWindowTime(void); 00209 00210 /** 00211 * Set the time of the window between tap latency and a double tap. 00212 * 00213 * @param window_ms The amount of time after the expiration of the latency 00214 * time during which a second valid tap can begin, 00215 * in milliseconds. 00216 */ 00217 void setWindowTime(int window_ms); 00218 00219 /** 00220 * Get the threshold value for detecting activity. 00221 * 00222 * @return The threshold value for detecting activity as an 8-bit number. 00223 * Scale factor is 62.5mg/LSB. 00224 */ 00225 int getActivityThreshold(void); 00226 00227 /** 00228 * Set the threshold value for detecting activity. 00229 * 00230 * @param threshold The threshold value for detecting activity as an 8-bit 00231 * number. Scale factor is 62.5mg/LSB. A value of 0 may 00232 * result in undesirable behavior if the activity 00233 * interrupt is enabled. 00234 */ 00235 void setActivityThreshold(int threshold); 00236 00237 /** 00238 * Get the threshold value for detecting inactivity. 00239 * 00240 * @return The threshold value for detecting inactivity as an 8-bit number. 00241 * Scale factor is 62.5mg/LSB. 00242 */ 00243 int getInactivityThreshold(void); 00244 00245 /** 00246 * Set the threshold value for detecting inactivity. 00247 * 00248 * @param threshold The threshold value for detecting inactivity as an 00249 * 8-bit number. Scale factor is 62.5mg/LSB. 00250 */ 00251 void setInactivityThreshold(int threshold); 00252 00253 /** 00254 * Get the time required for inactivity to be declared. 00255 * 00256 * @return The amount of time that acceleration must be less than the 00257 * inactivity threshold for inactivity to be declared, in 00258 * seconds. 00259 */ 00260 int getTimeInactivity(void); 00261 00262 /** 00263 * Set the time required for inactivity to be declared. 00264 * 00265 * @param inactivity The amount of time that acceleration must be less than 00266 * the inactivity threshold for inactivity to be 00267 * declared, in seconds. A value of 0 results in an 00268 * interrupt when the output data is less than the 00269 * threshold inactivity. 00270 */ 00271 void setTimeInactivity(int timeInactivity); 00272 00273 /** 00274 * Get the activity/inactivity control settings. 00275 * 00276 * D7 D6 D5 D4 00277 * +-----------+--------------+--------------+--------------+ 00278 * | ACT ac/dc | ACT_X enable | ACT_Y enable | ACT_Z enable | 00279 * +-----------+--------------+--------------+--------------+ 00280 * 00281 * D3 D2 D1 D0 00282 * +-------------+----------------+----------------+----------------+ 00283 * | INACT ac/dc | INACT_X enable | INACT_Y enable | INACT_Z enable | 00284 * +-------------+----------------+----------------+----------------+ 00285 * 00286 * See datasheet for details. 00287 * 00288 * @return The contents of the ACT_INACT_CTL register. 00289 */ 00290 int getActivityInactivityControl(void); 00291 00292 /** 00293 * Set the activity/inactivity control settings. 00294 * 00295 * D7 D6 D5 D4 00296 * +-----------+--------------+--------------+--------------+ 00297 * | ACT ac/dc | ACT_X enable | ACT_Y enable | ACT_Z enable | 00298 * +-----------+--------------+--------------+--------------+ 00299 * 00300 * D3 D2 D1 D0 00301 * +-------------+----------------+----------------+----------------+ 00302 * | INACT ac/dc | INACT_X enable | INACT_Y enable | INACT_Z enable | 00303 * +-------------+----------------+----------------+----------------+ 00304 * 00305 * See datasheet for details. 00306 * 00307 * @param settings The control byte to write to the ACT_INACT_CTL register. 00308 */ 00309 void setActivityInactivityControl(int settings); 00310 00311 /** 00312 * Get the threshold for free fall detection. 00313 * 00314 * @return The threshold value for free-fall detection, as an 8-bit number, 00315 * with scale factor 62.5mg/LSB. 00316 */ 00317 int getFreefallThreshold(void); 00318 00319 /** 00320 * Set the threshold for free fall detection. 00321 * 00322 * @return The threshold value for free-fall detection, as an 8-bit number, 00323 * with scale factor 62.5mg/LSB. A value of 0 may result in 00324 * undesirable behavior if the free-fall interrupt is enabled. 00325 * Values between 300 mg and 600 mg (0x05 to 0x09) are recommended. 00326 */ 00327 void setFreefallThreshold(int threshold); 00328 00329 /** 00330 * Get the time required to generate a free fall interrupt. 00331 * 00332 * @return The minimum time that the value of all axes must be less than 00333 * the freefall threshold to generate a free-fall interrupt, in 00334 * milliseconds. 00335 */ 00336 int getFreefallTime(void); 00337 00338 /** 00339 * Set the time required to generate a free fall interrupt. 00340 * 00341 * @return The minimum time that the value of all axes must be less than 00342 * the freefall threshold to generate a free-fall interrupt, in 00343 * milliseconds. A value of 0 may result in undesirable behavior 00344 * if the free-fall interrupt is enabled. Values between 100 ms 00345 * and 350 ms (0x14 to 0x46) are recommended. 00346 */ 00347 void setFreefallTime(int freefallTime_ms); 00348 00349 /** 00350 * Get the axis tap settings. 00351 * 00352 * D3 D2 D1 D0 00353 * +----------+--------------+--------------+--------------+ 00354 * | Suppress | TAP_X enable | TAP_Y enable | TAP_Z enable | 00355 * +----------+--------------+--------------+--------------+ 00356 * 00357 * (D7-D4 are 0s). 00358 * 00359 * See datasheet for more details. 00360 * 00361 * @return The contents of the TAP_AXES register. 00362 */ 00363 int getTapAxisControl(void); 00364 00365 /** 00366 * Set the axis tap settings. 00367 * 00368 * D3 D2 D1 D0 00369 * +----------+--------------+--------------+--------------+ 00370 * | Suppress | TAP_X enable | TAP_Y enable | TAP_Z enable | 00371 * +----------+--------------+--------------+--------------+ 00372 * 00373 * (D7-D4 are 0s). 00374 * 00375 * See datasheet for more details. 00376 * 00377 * @param The control byte to write to the TAP_AXES register. 00378 */ 00379 void setTapAxisControl(int settings); 00380 00381 /** 00382 * Get the source of a tap. 00383 * 00384 * @return The contents of the ACT_TAP_STATUS register. 00385 */ 00386 int getTapSource(void); 00387 00388 /** 00389 * Set the power mode. 00390 * 00391 * @param mode 0 -> Normal operation. 00392 * 1 -> Reduced power operation. 00393 */ 00394 void setPowerMode(char mode); 00395 00396 /** 00397 * Set the data rate. 00398 * 00399 * @param rate The rate code (see #defines or datasheet). 00400 */ 00401 void setDataRate(int rate); 00402 00403 /** 00404 * Get the power control settings. 00405 * 00406 * See datasheet for details. 00407 * 00408 * @return The contents of the POWER_CTL register. 00409 */ 00410 int getPowerControl(void); 00411 00412 /** 00413 * Set the power control settings. 00414 * 00415 * See datasheet for details. 00416 * 00417 * @param The control byte to write to the POWER_CTL register. 00418 */ 00419 void setPowerControl(int settings); 00420 00421 /** 00422 * Get the interrupt enable settings. 00423 * 00424 * @return The contents of the INT_ENABLE register. 00425 */ 00426 int getInterruptEnableControl(void); 00427 00428 /** 00429 * Set the interrupt enable settings. 00430 * 00431 * @param settings The control byte to write to the INT_ENABLE register. 00432 */ 00433 void setInterruptEnableControl(int settings); 00434 00435 /** 00436 * Get the interrupt mapping settings. 00437 * 00438 * @return The contents of the INT_MAP register. 00439 */ 00440 int getInterruptMappingControl(void); 00441 00442 /** 00443 * Set the interrupt mapping settings. 00444 * 00445 * @param settings The control byte to write to the INT_MAP register. 00446 */ 00447 void setInterruptMappingControl(int settings); 00448 00449 /** 00450 * Get the interrupt source. 00451 * 00452 * @return The contents of the INT_SOURCE register. 00453 */ 00454 int getInterruptSource(void); 00455 00456 /** 00457 * Get the data format settings. 00458 * 00459 * @return The contents of the DATA_FORMAT register. 00460 */ 00461 int getDataFormatControl(void); 00462 00463 /** 00464 * Set the data format settings. 00465 * 00466 * @param settings The control byte to write to the DATA_FORMAT register. 00467 */ 00468 void setDataFormatControl(int settings); 00469 00470 /** 00471 * Get the output of all three axes. 00472 * 00473 * @param Pointer to a buffer to hold the accelerometer value for the 00474 * x-axis, y-axis and z-axis [in that order]. 00475 */ 00476 void getOutput(int* readings); 00477 00478 00479 /** 00480 * Get acceleration of X axis. 00481 * 00482 *@return Accelerometer value for the x-axis. 00483 */ 00484 int getAx(); 00485 00486 00487 /** 00488 * Get acceleration of Y axis. 00489 * 00490 *@return Accelerometer value for the y-axis 00491 */ 00492 int getAy(); 00493 00494 00495 /** 00496 * Get acceleration of Z axis. 00497 * 00498 *@return Accelerometer value for the z-axis 00499 */ 00500 int getAz(); 00501 00502 00503 00504 00505 00506 /** 00507 * Get the FIFO control settings. 00508 * 00509 * @return The contents of the FIFO_CTL register. 00510 */ 00511 int getFifoControl(void); 00512 00513 /** 00514 * Set the FIFO control settings. 00515 * 00516 * @param The control byte to write to the FIFO_CTL register. 00517 */ 00518 void setFifoControl(int settings); 00519 00520 /** 00521 * Get FIFO status. 00522 * 00523 * @return The contents of the FIFO_STATUS register. 00524 */ 00525 int getFifoStatus(void); 00526 00527 private: 00528 I2C i2c_; 00529 00530 /** 00531 * Read one byte from a register on the device. 00532 * 00533 * @param address Address of the register to read. 00534 * 00535 * @return The contents of the register address. 00536 */ 00537 char oneByteRead(char address); 00538 00539 /** 00540 * Write one byte to a register on the device. 00541 * 00542 * @param address Address of the register to write to. 00543 * @param data The data to write into the register. 00544 */ 00545 void oneByteWrite(char address, char data); 00546 00547 /** 00548 * Read Two consecutive bytes on the device. 00549 * 00550 * @param startAddress The address of the first register to read from. 00551 * @param buffer Pointer to a buffer to store data read from the device. 00552 * 00553 */ 00554 void TwoByteRead(char startAddress, char* buffer); 00555 00556 /** 00557 * Write Two consecutive bytes on the device. 00558 * 00559 * @param startAddress The address of the first register to write to. 00560 * @param buffer Pointer to a buffer which contains the data to write. 00561 * 00562 */ 00563 void TwoByteWrite(char startAddress, char* buffer); 00564 00565 }; 00566 00567 #endif /* ADXL345_I2C_H */
Generated on Tue Jul 12 2022 20:44:24 by
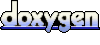