Dependents: ADXL345 TCPspittertobi ADXL345 RS485R_2 ... more
ADXL345_I2C.cpp
00001 /** 00002 * @author Jose R. Padron 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ADXL345, triple axis, digital interface, accelerometer. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.analog.com/static/imported-files/data_sheets/ADXL345.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 #include "ADXL345_I2C.h" 00039 00040 ADXL345_I2C::ADXL345_I2C(PinName sda, 00041 PinName scl) : i2c_(sda,scl) { 00042 00043 00044 //100KHz, as specified by the datasheet. 00045 i2c_.frequency(100000); 00046 wait_us(500); 00047 00048 } 00049 00050 char ADXL345_I2C::getDevId(void) { 00051 00052 return oneByteRead(ADXL345_DEVID_REG); 00053 00054 } 00055 00056 int ADXL345_I2C::getTapThreshold(void) { 00057 00058 return oneByteRead(ADXL345_THRESH_TAP_REG); 00059 00060 } 00061 00062 void ADXL345_I2C::setTapThreshold(int threshold) { 00063 00064 oneByteWrite(ADXL345_THRESH_TAP_REG, threshold); 00065 00066 } 00067 00068 int ADXL345_I2C::getOffset(int axis) { 00069 00070 int address = 0; 00071 00072 if (axis == ADXL345_X) { 00073 address = ADXL345_OFSX_REG; 00074 } else if (axis == ADXL345_Y) { 00075 address = ADXL345_OFSY_REG; 00076 } else if (axis == ADXL345_Z) { 00077 address = ADXL345_OFSZ_REG; 00078 } 00079 00080 return oneByteRead(address); 00081 00082 } 00083 00084 void ADXL345_I2C::setOffset(int axis, char offset) { 00085 00086 char address = 0; 00087 00088 if (axis == ADXL345_X) { 00089 address = ADXL345_OFSX_REG; 00090 } else if (axis == ADXL345_Y) { 00091 address = ADXL345_OFSY_REG; 00092 } else if (axis == ADXL345_Z) { 00093 address = ADXL345_OFSZ_REG; 00094 } 00095 00096 return oneByteWrite(address, offset); 00097 00098 } 00099 00100 int ADXL345_I2C::getTapDuration(void) { 00101 00102 return oneByteRead(ADXL345_DUR_REG)*625; 00103 00104 } 00105 00106 void ADXL345_I2C::setTapDuration(int duration_us) { 00107 00108 int tapDuration = duration_us / 625; 00109 00110 oneByteWrite(ADXL345_DUR_REG, tapDuration); 00111 00112 } 00113 00114 float ADXL345_I2C::getTapLatency(void) { 00115 00116 return oneByteRead(ADXL345_LATENT_REG)*1.25; 00117 00118 } 00119 00120 void ADXL345_I2C::setTapLatency(int latency_ms) { 00121 00122 int tapLatency = latency_ms / 1.25; 00123 00124 oneByteWrite(ADXL345_LATENT_REG, tapLatency); 00125 00126 } 00127 00128 float ADXL345_I2C::getWindowTime(void) { 00129 00130 return oneByteRead(ADXL345_WINDOW_REG)*1.25; 00131 00132 } 00133 00134 void ADXL345_I2C::setWindowTime(int window_ms) { 00135 00136 int windowTime = window_ms / 1.25; 00137 00138 oneByteWrite(ADXL345_WINDOW_REG, windowTime); 00139 00140 } 00141 00142 int ADXL345_I2C::getActivityThreshold(void) { 00143 00144 return oneByteRead(ADXL345_THRESH_ACT_REG); 00145 00146 } 00147 00148 void ADXL345_I2C::setActivityThreshold(int threshold) { 00149 00150 oneByteWrite(ADXL345_THRESH_ACT_REG, threshold); 00151 00152 } 00153 00154 int ADXL345_I2C::getInactivityThreshold(void) { 00155 00156 return oneByteRead(ADXL345_THRESH_INACT_REG); 00157 00158 } 00159 00160 void ADXL345_I2C::setInactivityThreshold(int threshold) { 00161 00162 return oneByteWrite(ADXL345_THRESH_INACT_REG, threshold); 00163 00164 } 00165 00166 int ADXL345_I2C::getTimeInactivity(void) { 00167 00168 return oneByteRead(ADXL345_TIME_INACT_REG); 00169 00170 } 00171 00172 void ADXL345_I2C::setTimeInactivity(int timeInactivity) { 00173 00174 oneByteWrite(ADXL345_TIME_INACT_REG, timeInactivity); 00175 00176 } 00177 00178 int ADXL345_I2C::getActivityInactivityControl(void) { 00179 00180 return oneByteRead(ADXL345_ACT_INACT_CTL_REG); 00181 00182 } 00183 00184 void ADXL345_I2C::setActivityInactivityControl(int settings) { 00185 00186 oneByteWrite(ADXL345_ACT_INACT_CTL_REG, settings); 00187 00188 } 00189 00190 int ADXL345_I2C::getFreefallThreshold(void) { 00191 00192 return oneByteRead(ADXL345_THRESH_FF_REG); 00193 00194 } 00195 00196 void ADXL345_I2C::setFreefallThreshold(int threshold) { 00197 00198 oneByteWrite(ADXL345_THRESH_FF_REG, threshold); 00199 00200 } 00201 00202 int ADXL345_I2C::getFreefallTime(void) { 00203 00204 return oneByteRead(ADXL345_TIME_FF_REG)*5; 00205 00206 } 00207 00208 void ADXL345_I2C::setFreefallTime(int freefallTime_ms) { 00209 00210 int freefallTime = freefallTime_ms / 5; 00211 00212 oneByteWrite(ADXL345_TIME_FF_REG, freefallTime); 00213 00214 } 00215 00216 int ADXL345_I2C::getTapAxisControl(void) { 00217 00218 return oneByteRead(ADXL345_TAP_AXES_REG); 00219 00220 } 00221 00222 void ADXL345_I2C::setTapAxisControl(int settings) { 00223 00224 oneByteWrite(ADXL345_TAP_AXES_REG, settings); 00225 00226 } 00227 00228 int ADXL345_I2C::getTapSource(void) { 00229 00230 return oneByteRead(ADXL345_ACT_TAP_STATUS_REG); 00231 00232 } 00233 00234 void ADXL345_I2C::setPowerMode(char mode) { 00235 00236 //Get the current register contents, so we don't clobber the rate value. 00237 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00238 00239 registerContents = (mode << 4) | registerContents; 00240 00241 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00242 00243 } 00244 00245 int ADXL345_I2C::getPowerControl(void) { 00246 00247 return oneByteRead(ADXL345_POWER_CTL_REG); 00248 00249 } 00250 00251 void ADXL345_I2C::setPowerControl(int settings) { 00252 00253 oneByteWrite(ADXL345_POWER_CTL_REG, settings); 00254 00255 } 00256 00257 int ADXL345_I2C::getInterruptEnableControl(void) { 00258 00259 return oneByteRead(ADXL345_INT_ENABLE_REG); 00260 00261 } 00262 00263 void ADXL345_I2C::setInterruptEnableControl(int settings) { 00264 00265 oneByteWrite(ADXL345_INT_ENABLE_REG, settings); 00266 00267 } 00268 00269 int ADXL345_I2C::getInterruptMappingControl(void) { 00270 00271 return oneByteRead(ADXL345_INT_MAP_REG); 00272 00273 } 00274 00275 void ADXL345_I2C::setInterruptMappingControl(int settings) { 00276 00277 oneByteWrite(ADXL345_INT_MAP_REG, settings); 00278 00279 } 00280 00281 int ADXL345_I2C::getInterruptSource(void){ 00282 00283 return oneByteRead(ADXL345_INT_SOURCE_REG); 00284 00285 } 00286 00287 int ADXL345_I2C::getDataFormatControl(void){ 00288 00289 return oneByteRead(ADXL345_DATA_FORMAT_REG); 00290 00291 } 00292 00293 void ADXL345_I2C::setDataFormatControl(int settings){ 00294 00295 oneByteWrite(ADXL345_DATA_FORMAT_REG, settings); 00296 00297 } 00298 00299 void ADXL345_I2C::setDataRate(int rate) { 00300 00301 //Get the current register contents, so we don't clobber the power bit. 00302 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00303 00304 registerContents &= 0x10; 00305 registerContents |= rate; 00306 00307 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00308 00309 } 00310 00311 int ADXL345_I2C::getAx(){ 00312 00313 char buffer[2]; 00314 00315 TwoByteRead(ADXL345_DATAX0_REG, buffer); 00316 00317 return ((int)buffer[1] << 8 | (int)buffer[0]); 00318 } 00319 00320 00321 int ADXL345_I2C::getAy(){ 00322 00323 char buffer[2]; 00324 00325 TwoByteRead(ADXL345_DATAY0_REG, buffer); 00326 00327 return ((int)buffer[1] << 8 | (int)buffer[0]); 00328 } 00329 00330 int ADXL345_I2C::getAz(){ 00331 00332 char buffer[2]; 00333 00334 TwoByteRead(ADXL345_DATAZ0_REG, buffer); 00335 00336 return ((int)buffer[1] << 8 | (int)buffer[0]); 00337 } 00338 00339 00340 00341 void ADXL345_I2C::getOutput(int* readings){ 00342 00343 char buffer[2]; 00344 00345 TwoByteRead(ADXL345_DATAX0_REG, buffer); 00346 readings[0] = (int)buffer[1] << 8 | (int)buffer[0]; 00347 TwoByteRead(ADXL345_DATAY0_REG, buffer); 00348 readings[1] = (int)buffer[1] << 8 | (int)buffer[0]; 00349 TwoByteRead(ADXL345_DATAZ0_REG, buffer); 00350 readings[2] = (int)buffer[1] << 8 | (int)buffer[0]; 00351 00352 } 00353 00354 int ADXL345_I2C::getFifoControl(void){ 00355 00356 return oneByteRead(ADXL345_FIFO_CTL); 00357 00358 } 00359 00360 void ADXL345_I2C::setFifoControl(int settings){ 00361 00362 oneByteWrite(ADXL345_FIFO_STATUS, settings); 00363 00364 } 00365 00366 int ADXL345_I2C::getFifoStatus(void){ 00367 00368 return oneByteRead(ADXL345_FIFO_STATUS); 00369 00370 } 00371 00372 char ADXL345_I2C::oneByteRead(char address) { 00373 00374 00375 char rx[1]; 00376 char tx[1]; 00377 00378 // nCS_ = 1; 00379 tx[0]=address; 00380 00381 00382 i2c_.write(ADXL345_I2C_WRITE, tx,1); 00383 i2c_.read(ADXL345_I2C_READ,rx,1); 00384 00385 //nCS_ = 0; 00386 return rx[0]; 00387 00388 } 00389 00390 void ADXL345_I2C::oneByteWrite(char address, char data) { 00391 // nCS_ = 1; 00392 char tx[2]; 00393 00394 tx[0]=address; 00395 tx[1]=data; 00396 00397 i2c_.write(ADXL345_I2C_WRITE,tx,2); 00398 00399 //nCS_ = 0; 00400 } 00401 00402 void ADXL345_I2C::TwoByteRead(char startAddress, char* buffer) { 00403 00404 00405 // nCS_ = 1; 00406 //Send address to start reading from. 00407 char tx[1]; 00408 tx[0]=startAddress; 00409 i2c_.write(ADXL345_I2C_WRITE,tx,1); 00410 i2c_.read(ADXL345_I2C_READ,buffer,2); 00411 00412 //nCS_ = 0; 00413 } 00414 00415 void ADXL345_I2C::TwoByteWrite(char startAddress, char* buffer) { 00416 00417 //nCS_ = 1; 00418 //Send address to start reading from. 00419 char tx[1]; 00420 tx[0]=startAddress; 00421 i2c_.write(ADXL345_I2C_WRITE,tx,1); 00422 i2c_.write(ADXL345_I2C_WRITE,buffer,2); 00423 00424 00425 //nCS_ = 0; 00426 00427 }
Generated on Tue Jul 12 2022 20:44:24 by
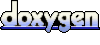