
1
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include <sstream> 00004 #include <vector> 00005 #include "ESP8266.h" 00006 00007 int localOutPort = 49100; 00008 int localInPort = 49101; 00009 00010 char IP[16]; //IP of client app 00011 bool wasHandshake = false; //was handshake with client or not 00012 00013 Serial console(USBTX,USBRX); 00014 ESP8266 wifi(PF_7,PF_6,localOutPort,localInPort); 00015 00016 const char* ap = "Clapeyron_Industries"; 00017 const char* passPhrase = "06737184"; 00018 00019 Thread listeningThread; 00020 00021 void onReceive(void); 00022 void processReceivedData(string&, char*, int); 00023 std::vector<std::string> split(const std::string&, char); 00024 00025 //сначала запускается AP, надо подключиться прямо к точке RobotClapeyronAP (пароль passpass123), 00026 //либо начать общение, либо передать данные о подключении робота к другой AP: имя, пароль 00027 int main() { 00028 console.baud(9600); 00029 if (wifi.startup(3) && wifi.setupAP()) { 00030 console.printf("AP set up\n"); 00031 console.printf("Your IP is: %s\n",wifi.getIPAddress()); 00032 } 00033 else 00034 console.printf("Can not set up AP\n"); 00035 listeningThread.start(onReceive); 00036 } 00037 00038 void onReceive(void) { 00039 const int maxSize = 100; 00040 00041 char buffer[100]; 00042 string buf = ""; 00043 int port; 00044 int bytes; 00045 while(1) { 00046 buf = ""; 00047 bytes = wifi.recv(&buffer,maxSize,IP,&port); 00048 if (bytes != -1) { 00049 console.printf("Bytes received: %d; from %s:%d\n",bytes,IP,port); 00050 for(int i = 0; i < bytes; i++) { 00051 buf += buffer[i]; 00052 } 00053 console.printf("Data: %s\n",buf); 00054 processReceivedData(buf,IP,port); 00055 } 00056 } 00057 } 00058 00059 void processReceivedData(string& data, char* IP, int port) { 00060 if (port == localOutPort) { //TODO проверка того, что разговариваем с клиентом 00061 std::vector<std::string> splittedStream = split(data, '?'); 00062 if (splittedStream.size() > 1) { 00063 std::vector<std::string> splittedMessage = split(splittedStream[1],'|'); //TODO цикл по splittedStream от второго (1) до предпоследнего (size-2) 00064 if (splittedMessage.size()>0) 00065 /*-->*/ if (splittedMessage[0] == "HiRobotClapeyronImAClient") { 00066 wasHandshake = true; //TODO говорим, что с этим клиентом поздоровались (сейчас вообще как будто один клиент может быть) 00067 string msg = "?HiClientImARobotClapeyron|hardvers|0.1?"; 00068 wifi.send(msg.c_str(),msg.length(),IP,localInPort); 00069 } else if (wasHandshake) //TODO проверка того, что работаем с поздоровавшимся клиентом 00070 /*-->*/ if ((splittedMessage[0] == "ConnectToTheAP") && (splittedMessage.size() >= 3)) { //TODO проверка формата сообщения не полная, разумеется 00071 if (wifi.disconnect() && wifi.connect(splittedMessage[1].c_str(),splittedMessage[2].c_str())) { 00072 string msg = "?ConnectedToTheAP|"+splittedMessage[1]+"|IP|"+wifi.getIPAddress()+"?"; 00073 console.printf(msg.c_str()); //Debug 00074 wifi.send(msg.c_str(),msg.length(),IP,localInPort); 00075 } else { 00076 string msg = "?CanNotConnectToTheAP|"+splittedMessage[1]+"?"; 00077 console.printf(msg.c_str()); //Debug 00078 wifi.send(msg.c_str(),msg.length(),IP,localInPort); 00079 } /*else 00080 --> if () {}*/ 00081 } 00082 } 00083 } 00084 } 00085 00086 std::vector<std::string> split(const std::string &s, char delim) { 00087 std::stringstream ss(s); 00088 std::string item; 00089 std::vector<std::string> elems; 00090 while (std::getline(ss, item, delim)) { 00091 elems.push_back(item); 00092 // elems.push_back(std::move(item)); // if C++11 00093 } 00094 return elems; 00095 }
Generated on Tue Jul 26 2022 21:51:59 by
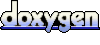