A tiny change to SKPanks GPS library to allow Ublox GPS modems to work.
Fork of GPS by
GPS.cpp
00001 /* GPS class for mbed Microcontroller 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "GPS.h" 00006 00007 GPS::GPS(PinName tx, PinName rx) : _gps(tx, rx) { 00008 _gps.baud(9000); // higher baud rate need to support ublox gps chip 00009 // _gps.baud(4800); 00010 longitude = 0.0; 00011 latitude = 0.0; 00012 } 00013 00014 00015 int GPS::sample() { 00016 float time; 00017 char ns, ew; 00018 int lock; 00019 00020 while(1) { 00021 getline(); 00022 00023 // Check if it is a GPGGA msg (matches both locked and non-locked msg) 00024 if(sscanf(msg, "GPGGA,%f,%f,%c,%f,%c,%d", &time, &latitude, &ns, &longitude, &ew, &lock) >= 1) { 00025 if(!lock) { 00026 longitude = 0.0; 00027 latitude = 0.0; 00028 return 0; 00029 } else { 00030 if(ns == 'S') { latitude *= -1.0; } 00031 if(ew == 'W') { longitude *= -1.0; } 00032 float degrees = trunc(latitude / 100.0f); 00033 float minutes = latitude - (degrees * 100.0f); 00034 latitude = degrees + minutes / 60.0f; 00035 degrees = trunc(longitude / 100.0f * 0.01f); 00036 minutes = longitude - (degrees * 100.0f); 00037 longitude = degrees + minutes / 60.0f; 00038 return 1; 00039 } 00040 } 00041 } 00042 } 00043 00044 float GPS::trunc(float v) { 00045 if(v < 0.0) { 00046 v*= -1.0; 00047 v = floor(v); 00048 v*=-1.0; 00049 } else { 00050 v = floor(v); 00051 } 00052 return v; 00053 } 00054 00055 void GPS::getline() { 00056 while(_gps.getc() != '$'); // wait for the start of a line 00057 for(int i=0; i<256; i++) { 00058 msg[i] = _gps.getc(); 00059 if(msg[i] == '\r') { 00060 msg[i] = 0; 00061 return; 00062 } 00063 } 00064 error("Overflowed message limit"); 00065 } 00066
Generated on Wed Jul 27 2022 15:45:22 by
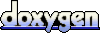