XRange SX1272Lib
Dependents: XRangePingPong XRange-LoRaWAN-lmic-app lora-transceiver
Fork of SX1276Lib by
sx1272.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 ( C )2014 Semtech 00008 00009 Description: Actual implementation of a SX1276 radio, inherits Radio 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: www.netblocks.eu 00014 SX1272 LoRa RF module : http://www.netblocks.eu/xrange-sx1272-lora-datasheet/ 00015 00016 */ 00017 #ifndef __SX1272_H__ 00018 #define __SX1272_H__ 00019 00020 #include "radio.h" 00021 #include "./registers/sx1272Regs-Fsk.h" 00022 #include "./registers/sx1272Regs-LoRa.h" 00023 #include "./typedefs/typedefs.h" 00024 00025 /*! 00026 * Radio wakeup time from SLEEP mode 00027 */ 00028 #define RADIO_WAKEUP_TIME 1000 // [us] 00029 00030 /*! 00031 * SX1276 definitions 00032 */ 00033 #define XTAL_FREQ 32000000 00034 #define FREQ_STEP 61.03515625 00035 00036 #define RX_BUFFER_SIZE 256 00037 00038 #define DEFAULT_TIMEOUT 200 //usec 00039 #define RSSI_OFFSET -139.0 00040 00041 00042 /*! 00043 * Constant values need to compute the RSSI value 00044 */ 00045 //#define RSSI_OFFSET_LF -164.0 00046 //#define RSSI_OFFSET_HF -157.0 00047 00048 //#define RF_MID_BAND_THRESH 525000000 00049 00050 /*! 00051 * Actual implementation of a SX1276 radio, inherits Radio 00052 */ 00053 class SX1272 : public Radio 00054 { 00055 protected: 00056 /*! 00057 * SPI Interface 00058 */ 00059 SPI spi ; // mosi, miso, sclk 00060 DigitalOut nss; 00061 00062 /*! 00063 * SX1276 Reset pin 00064 */ 00065 DigitalInOut reset ; 00066 00067 /*! 00068 * SX1276 DIO pins 00069 */ 00070 InterruptIn dio0 ; 00071 InterruptIn dio1; 00072 InterruptIn dio2; 00073 InterruptIn dio3; 00074 InterruptIn dio4; 00075 DigitalIn dio5; 00076 00077 bool isRadioActive; 00078 00079 uint8_t *rxBuffer; 00080 00081 uint8_t previousOpMode; 00082 00083 /*! 00084 * Hardware DIO IRQ functions 00085 */ 00086 DioIrqHandler *dioIrq ; 00087 00088 /*! 00089 * Tx and Rx timers 00090 */ 00091 Timeout txTimeoutTimer ; 00092 Timeout rxTimeoutTimer; 00093 Timeout rxTimeoutSyncWord; 00094 00095 /*! 00096 * rxTx: [1: Tx, 0: Rx] 00097 */ 00098 uint8_t rxTx ; 00099 00100 RadioSettings_t settings; 00101 00102 static const FskBandwidth_t FskBandwidths[] ; 00103 protected: 00104 00105 /*! 00106 * Performs the Rx chain calibration for LF and HF bands 00107 * \remark Must be called just after the reset so all registers are at their 00108 * default values 00109 */ 00110 void RxChainCalibration ( void ); 00111 00112 public: 00113 SX1272 ( void ( *txDone )( ), void ( *txTimeout ) ( ), void ( *rxDone ) ( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ), 00114 void ( *rxTimeout ) ( ), void ( *rxError ) ( ), void ( *fhssChangeChannel ) ( uint8_t channelIndex ), void ( *cadDone ) ( bool channelActivityDetected ), 00115 PinName mosi, PinName miso, PinName sclk, PinName nss, PinName reset , 00116 PinName dio0 , PinName dio1, PinName dio2, PinName dio3, PinName dio4, PinName dio5 ); 00117 SX1272 ( void ( *txDone )( ), void ( *txTimeout ) ( ), void ( *rxDone ) ( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ), 00118 void ( *rxTimeout ) ( ), void ( *rxError ) ( ), void ( *fhssChangeChannel ) ( uint8_t channelIndex ), void ( *cadDone ) ( bool channelActivityDetected ) ); 00119 virtual ~SX1272 ( ); 00120 00121 //------------------------------------------------------------------------- 00122 // Redefined Radio functions 00123 //------------------------------------------------------------------------- 00124 /*! 00125 * Return current radio status 00126 * 00127 * @param status Radio status. [IDLE, RX_RUNNING, TX_RUNNING] 00128 */ 00129 virtual RadioState GetState ( void ); 00130 00131 /*! 00132 * @brief Configures the SX1276 with the given modem 00133 * 00134 * @param [IN] modem Modem to be used [0: FSK, 1: LoRa] 00135 */ 00136 virtual void SetModem( ModemType modem ); 00137 00138 /*! 00139 * @brief Sets the channel frequency 00140 * 00141 * @param [IN] freq Channel RF frequency 00142 */ 00143 virtual void SetChannel( uint32_t freq ); 00144 00145 /*! 00146 * @brief Sets the channels configuration 00147 * 00148 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00149 * @param [IN] freq Channel RF frequency 00150 * @param [IN] rssiThresh RSSI threshold 00151 * 00152 * @retval isFree [true: Channel is free, false: Channel is not free] 00153 */ 00154 virtual bool IsChannelFree( ModemType modem, uint32_t freq, int8_t rssiThresh ); 00155 00156 /*! 00157 * @brief Generates a 32 bits random value based on the RSSI readings 00158 * 00159 * \remark This function sets the radio in LoRa modem mode and disables 00160 * all interrupts. 00161 * After calling this function either Radio.SetRxConfig or 00162 * Radio.SetTxConfig functions must be called. 00163 * 00164 * @retval randomValue 32 bits random value 00165 */ 00166 virtual uint32_t Random( void ); 00167 00168 /*! 00169 * @brief Sets the reception parameters 00170 * 00171 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00172 * @param [IN] bandwidth Sets the bandwidth 00173 * FSK : >= 2600 and <= 250000 Hz 00174 * LoRa: [0: 125 kHz, 1: 250 kHz, 00175 * 2: 500 kHz, 3: Reserved] 00176 * @param [IN] datarate Sets the Datarate 00177 * FSK : 600..300000 bits/s 00178 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00179 * 10: 1024, 11: 2048, 12: 4096 chips] 00180 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00181 * FSK : N/A ( set to 0 ) 00182 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00183 * @param [IN] bandwidthAfc Sets the AFC Bandwidth ( FSK only ) 00184 * FSK : >= 2600 and <= 250000 Hz 00185 * LoRa: N/A ( set to 0 ) 00186 * @param [IN] preambleLen Sets the Preamble length ( LoRa only ) 00187 * FSK : N/A ( set to 0 ) 00188 * LoRa: Length in symbols ( the hardware adds 4 more symbols ) 00189 * @param [IN] symbTimeout Sets the RxSingle timeout value ( LoRa only ) 00190 * FSK : N/A ( set to 0 ) 00191 * LoRa: timeout in symbols 00192 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00193 * @param [IN] payloadLen Sets payload length when fixed lenght is used 00194 * @param [IN] crcOn Enables/Disables the CRC [0: OFF, 1: ON] 00195 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00196 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00197 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00198 * FSK : N/A ( set to 0 ) 00199 * LoRa: [0: not inverted, 1: inverted] 00200 * @param [IN] rxContinuous Sets the reception in continuous mode 00201 * [false: single mode, true: continuous mode] 00202 */ 00203 virtual void SetRxConfig ( ModemType modem, uint32_t bandwidth, 00204 uint32_t datarate, uint8_t coderate, 00205 uint32_t bandwidthAfc, uint16_t preambleLen, 00206 uint16_t symbTimeout, bool fixLen, 00207 uint8_t payloadLen, 00208 bool crcOn, bool freqHopOn, uint8_t hopPeriod, 00209 bool iqInverted, bool rxContinuous ); 00210 00211 /*! 00212 * @brief Sets the transmission parameters 00213 * 00214 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00215 * @param [IN] power Sets the output power [dBm] 00216 * @param [IN] fdev Sets the frequency deviation ( FSK only ) 00217 * FSK : [Hz] 00218 * LoRa: 0 00219 * @param [IN] bandwidth Sets the bandwidth ( LoRa only ) 00220 * FSK : 0 00221 * LoRa: [0: 125 kHz, 1: 250 kHz, 00222 * 2: 500 kHz, 3: Reserved] 00223 * @param [IN] datarate Sets the Datarate 00224 * FSK : 600..300000 bits/s 00225 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00226 * 10: 1024, 11: 2048, 12: 4096 chips] 00227 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00228 * FSK : N/A ( set to 0 ) 00229 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00230 * @param [IN] preambleLen Sets the preamble length 00231 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00232 * @param [IN] crcOn Enables disables the CRC [0: OFF, 1: ON] 00233 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00234 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00235 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00236 * FSK : N/A ( set to 0 ) 00237 * LoRa: [0: not inverted, 1: inverted] 00238 * @param [IN] timeout Transmission timeout [us] 00239 */ 00240 virtual void SetTxConfig( ModemType modem, int8_t power, uint32_t fdev, 00241 uint32_t bandwidth, uint32_t datarate, 00242 uint8_t coderate, uint16_t preambleLen, 00243 bool fixLen, bool crcOn, bool freqHopOn, 00244 uint8_t hopPeriod, bool iqInverted, uint32_t timeout ); 00245 00246 /*! 00247 * @brief Computes the packet time on air for the given payload 00248 * 00249 * \Remark Can only be called once SetRxConfig or SetTxConfig have been called 00250 * 00251 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00252 * @param [IN] pktLen Packet payload length 00253 * 00254 * @retval airTime Computed airTime for the given packet payload length 00255 */ 00256 virtual double TimeOnAir ( ModemType modem, uint8_t pktLen ); 00257 00258 /*! 00259 * @brief Sends the buffer of size. Prepares the packet to be sent and sets 00260 * the radio in transmission 00261 * 00262 * @param [IN]: buffer Buffer pointer 00263 * @param [IN]: size Buffer size 00264 */ 00265 virtual void Send( uint8_t *buffer, uint8_t size ); 00266 00267 /*! 00268 * @brief Sets the radio in sleep mode 00269 */ 00270 virtual void Sleep( void ); 00271 00272 /*! 00273 * @brief Sets the radio in standby mode 00274 */ 00275 virtual void Standby( void ); 00276 00277 /*! 00278 * @brief Sets the radio in reception mode for the given time 00279 * @param [IN] timeout Reception timeout [us] 00280 * [0: continuous, others timeout] 00281 */ 00282 virtual void Rx( uint32_t timeout ); 00283 00284 /*! 00285 * @brief Sets the radio in transmission mode for the given time 00286 * @param [IN] timeout Transmission timeout [us] 00287 * [0: continuous, others timeout] 00288 */ 00289 virtual void Tx( uint32_t timeout ); 00290 00291 /*! 00292 * @brief Start a Channel Activity Detection 00293 */ 00294 virtual void StartCad( void ); 00295 00296 /*! 00297 * @brief Reads the current RSSI value 00298 * 00299 * @retval rssiValue Current RSSI value in [dBm] 00300 */ 00301 virtual int16_t GetRssi ( ModemType modem ); 00302 00303 /*! 00304 * @brief Writes the radio register at the specified address 00305 * 00306 * @param [IN]: addr Register address 00307 * @param [IN]: data New register value 00308 */ 00309 virtual void Write ( uint8_t addr, uint8_t data ) = 0; 00310 00311 /*! 00312 * @brief Reads the radio register at the specified address 00313 * 00314 * @param [IN]: addr Register address 00315 * @retval data Register value 00316 */ 00317 virtual uint8_t Read ( uint8_t addr ) = 0; 00318 00319 /*! 00320 * @brief Writes multiple radio registers starting at address 00321 * 00322 * @param [IN] addr First Radio register address 00323 * @param [IN] buffer Buffer containing the new register's values 00324 * @param [IN] size Number of registers to be written 00325 */ 00326 virtual void Write( uint8_t addr, uint8_t *buffer, uint8_t size ) = 0; 00327 00328 /*! 00329 * @brief Reads multiple radio registers starting at address 00330 * 00331 * @param [IN] addr First Radio register address 00332 * @param [OUT] buffer Buffer where to copy the registers data 00333 * @param [IN] size Number of registers to be read 00334 */ 00335 virtual void Read ( uint8_t addr, uint8_t *buffer, uint8_t size ) = 0; 00336 00337 /*! 00338 * @brief Writes the buffer contents to the SX1276 FIFO 00339 * 00340 * @param [IN] buffer Buffer containing data to be put on the FIFO. 00341 * @param [IN] size Number of bytes to be written to the FIFO 00342 */ 00343 virtual void WriteFifo( uint8_t *buffer, uint8_t size ) = 0; 00344 00345 /*! 00346 * @brief Reads the contents of the SX1276 FIFO 00347 * 00348 * @param [OUT] buffer Buffer where to copy the FIFO read data. 00349 * @param [IN] size Number of bytes to be read from the FIFO 00350 */ 00351 virtual void ReadFifo( uint8_t *buffer, uint8_t size ) = 0; 00352 /*! 00353 * @brief Resets the SX1276 00354 */ 00355 virtual void Reset( void ) = 0; 00356 00357 //------------------------------------------------------------------------- 00358 // Board relative functions 00359 //------------------------------------------------------------------------- 00360 00361 protected: 00362 /*! 00363 * @brief Initializes the radio I/Os pins interface 00364 */ 00365 virtual void IoInit( void ) = 0; 00366 00367 /*! 00368 * @brief Initializes the radio registers 00369 */ 00370 virtual void RadioRegistersInit( ) = 0; 00371 00372 /*! 00373 * @brief Initializes the radio SPI 00374 */ 00375 virtual void SpiInit( void ) = 0; 00376 00377 /*! 00378 * @brief Initializes DIO IRQ handlers 00379 * 00380 * @param [IN] irqHandlers Array containing the IRQ callback functions 00381 */ 00382 virtual void IoIrqInit( DioIrqHandler *irqHandlers ) = 0; 00383 00384 /*! 00385 * @brief De-initializes the radio I/Os pins interface. 00386 * 00387 * \remark Useful when going in MCU lowpower modes 00388 */ 00389 virtual void IoDeInit( void ) = 0; 00390 00391 /*! 00392 * @brief Gets the board PA selection configuration 00393 * 00394 * @param [IN] channel Channel frequency in Hz 00395 * @retval PaSelect RegPaConfig PaSelect value 00396 */ 00397 virtual uint8_t GetPaSelect( uint32_t channel ) = 0; 00398 00399 /*! 00400 * @brief Set the RF Switch I/Os pins in Low Power mode 00401 * 00402 * @param [IN] status enable or disable 00403 */ 00404 virtual void SetAntSwLowPower( bool status ) = 0; 00405 00406 /*! 00407 * @brief Initializes the RF Switch I/Os pins interface 00408 */ 00409 virtual void AntSwInit( void ) = 0; 00410 00411 /*! 00412 * @brief De-initializes the RF Switch I/Os pins interface 00413 * 00414 * \remark Needed to decrease the power consumption in MCU lowpower modes 00415 */ 00416 virtual void AntSwDeInit( void ) = 0; 00417 00418 /*! 00419 * @brief Controls the antena switch if necessary. 00420 * 00421 * \remark see errata note 00422 * 00423 * @param [IN] rxTx [1: Tx, 0: Rx] 00424 */ 00425 virtual void SetAntSw( uint8_t rxTx ) = 0; 00426 00427 /*! 00428 * @brief Checks if the given RF frequency is supported by the hardware 00429 * 00430 * @param [IN] frequency RF frequency to be checked 00431 * @retval isSupported [true: supported, false: unsupported] 00432 */ 00433 virtual bool CheckRfFrequency( uint32_t frequency ) = 0; 00434 protected: 00435 00436 /*! 00437 * @brief Sets the SX1276 operating mode 00438 * 00439 * @param [IN] opMode New operating mode 00440 */ 00441 virtual void SetOpMode( uint8_t opMode ); 00442 00443 /* 00444 * SX1276 DIO IRQ callback functions prototype 00445 */ 00446 00447 /*! 00448 * @brief DIO 0 IRQ callback 00449 */ 00450 virtual void OnDio0Irq( void ); 00451 00452 /*! 00453 * @brief DIO 1 IRQ callback 00454 */ 00455 virtual void OnDio1Irq( void ); 00456 00457 /*! 00458 * @brief DIO 2 IRQ callback 00459 */ 00460 virtual void OnDio2Irq( void ); 00461 00462 /*! 00463 * @brief DIO 3 IRQ callback 00464 */ 00465 virtual void OnDio3Irq( void ); 00466 00467 /*! 00468 * @brief DIO 4 IRQ callback 00469 */ 00470 virtual void OnDio4Irq( void ); 00471 00472 /*! 00473 * @brief DIO 5 IRQ callback 00474 */ 00475 virtual void OnDio5Irq( void ); 00476 00477 /*! 00478 * @brief Tx & Rx timeout timer callback 00479 */ 00480 virtual void OnTimeoutIrq( void ); 00481 00482 /*! 00483 * Returns the known FSK bandwidth registers value 00484 * 00485 * \param [IN] bandwidth Bandwidth value in Hz 00486 * \retval regValue Bandwidth register value. 00487 */ 00488 static uint8_t GetFskBandwidthRegValue ( uint32_t bandwidth ); 00489 }; 00490 00491 #endif //__SX1272_H__
Generated on Wed Jul 13 2022 07:28:43 by
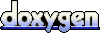