A lightweight AES implementation with Cipher Block Chaining and Ciphertext Stealing.
Dependents: AES_HelloWorld AES_ExtendedTests AESslave_modified_test AESslave_modified_test_27-9-2017 ... more
AES Class Reference
#include <AES.h>
Public Types | |
enum | KeySize { KEY_128 = 4, KEY_192 = 6, KEY_256 = 8 } |
Represents the different AES key sizes. More... | |
enum | CipherMode { MODE_ECB, MODE_CBC } |
Represents the different cipher modes. More... | |
Public Member Functions | |
AES () | |
Create a blank AES object. | |
AES (const char *key, KeySize keySize, CipherMode mode=MODE_ECB, const char *iv=NULL) | |
Create an AES object with the specified parameters. | |
~AES () | |
Destructor. | |
void | setup (const char *key, KeySize keySize, CipherMode mode=MODE_ECB, const char *iv=NULL) |
Set up this AES object for encryption/decryption with the specified parameters. | |
void | encrypt (void *data, size_t length) |
Encrypt the specified data in-place, using CTS or zero-padding if necessary. | |
void | encrypt (const void *src, char *dest, size_t length) |
Encrypt the specified data, using CTS or zero-padding if necessary. | |
void | decrypt (void *data, size_t length) |
Decrypt the specified data in-place, and remove the padding if necessary. | |
void | decrypt (const char *src, void *dest, size_t length) |
Decrypt the specified data, and remove the padding if necessary. | |
void | clear () |
Erase any sensitive information in this AES object. |
Detailed Description
AES class.
Used for encrypting/decrypting data using the AES block cipher.
Example:
#include "mbed.h" #include "AES.h" char message[] = { "Hello World!" }; const char key[32] = { 0x60, 0x3D, 0xEB, 0x10, 0x15, 0xCA, 0x71, 0xBE, 0x2B, 0x73, 0xAE, 0xF0, 0x85, 0x7D, 0x77, 0x81, 0x1F, 0x35, 0x2C, 0x07, 0x3B, 0x61, 0x08, 0xD7, 0x2D, 0x98, 0x10, 0xA3, 0x09, 0x14, 0xDF, 0xF4 }; const char iv[16] = { 0x74, 0x11, 0xF0, 0x45, 0xD6, 0xA4, 0x3F, 0x69, 0x18, 0xC6, 0x75, 0x42, 0xDF, 0x4C, 0xA7, 0x84 }; void printData(const void* data, size_t length) { const char* dataBytes = (const char*)data; for (size_t i = 0; i < length; i++) { if ((i % 8) == 0) printf("\n\t"); printf("0x%02X, ", dataBytes[i]); } printf("\n"); } int main() { AES aes; //Print the original message printf("Original message: \"%s\"", message); printData(message, sizeof(message)); //Encrypt the message in-place aes.setup(key, AES::KEY_256, AES::MODE_CBC, iv); aes.encrypt(message, sizeof(message)); aes.clear(); //Print the encrypted message printf("Encrypted message:"); printData(message, sizeof(message)); //Decrypt the message in-place aes.setup(key, AES::KEY_256, AES::MODE_CBC, iv); aes.decrypt(message, sizeof(message)); aes.clear(); //Print the decrypted message printf("Decrypted message: \"%s\"", message); printData(message, sizeof(message)); }
Definition at line 85 of file AES.h.
Member Enumeration Documentation
enum CipherMode |
enum KeySize |
Constructor & Destructor Documentation
AES | ( | const char * | key, |
KeySize | keySize, | ||
CipherMode | mode = MODE_ECB , |
||
const char * | iv = NULL |
||
) |
Member Function Documentation
void clear | ( | ) |
void decrypt | ( | const char * | src, |
void * | dest, | ||
size_t | length | ||
) |
void decrypt | ( | void * | data, |
size_t | length | ||
) |
void encrypt | ( | const void * | src, |
char * | dest, | ||
size_t | length | ||
) |
void encrypt | ( | void * | data, |
size_t | length | ||
) |
void setup | ( | const char * | key, |
KeySize | keySize, | ||
CipherMode | mode = MODE_ECB , |
||
const char * | iv = NULL |
||
) |
Generated on Tue Jul 12 2022 18:19:48 by
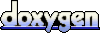