New
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /********************************** 00002 * Author: Seungcheol Baek 00003 * Institution: Georgia Tech 00004 * 00005 * Title: MAIN 00006 * Class: ECE2035 00007 * Assignment: Project 2 00008 **********************************/ 00009 00010 //includes 00011 #include <string.h> 00012 #include <stdio.h> 00013 #include <math.h> 00014 #include <cstdarg> 00015 #include "mbed.h" 00016 #include "rtos.h" 00017 #include "TextLCD.h" 00018 #include "SDFileSystem.h" 00019 #include "CommModule.h" 00020 #include "wave_player.h" 00021 #include "FATFileSystem.h" 00022 00023 //defines 00024 #define BAUDRATE 9600 00025 #define BUFFSIZE 100 00026 #define GRAVITY 1.0 00027 #define PHIGH 10 // NEW 00028 #define PLOW 5 // NEW 00029 #define PI 3.141592653589793238462643f 00030 #define BOMB_WAVFILE "/sd/wavfiles/bomb.wav" 00031 #define MONKEY_WAVFILE "/sd/wavfiles/monkey.wav" 00032 #define ACK "status-ack" 00033 00034 //function prototypes 00035 int invert(int value); 00036 void print(const char *format, ...); 00037 void lowerCase(char *src); 00038 void startGame(void); 00039 void waitForAck(void); 00040 void playBombSound(void); 00041 void playMonkeySound(void); 00042 void pb1_hit_callback(void); 00043 void pb2_hit_callback(void); 00044 void pb3_hit_callback(void); 00045 void pb4_hit_callback(void); 00046 void getworld (int**world, char *World); 00047 void updateShot(int row, int column, int del); 00048 void colorTile(int row, int column, int strength); 00049 void deleteTile(int row, int column); 00050 void paaUpdate(int power, int angle); 00051 void hint(int row, int column, int power, int angle); 00052 00053 //declare functions (assembly subroutines) 00054 extern "C" void setup_sequence(void); 00055 extern "C" void seg_driver_initialize(void); 00056 extern "C" void seg_driver(int value); 00057 00058 //initialize hardware 00059 SDFileSystem sd(p5, p6, p7, p8, "sd"); // mosi, miso, sck, cs 00060 TextLCD lcd(p26, p25, p24, p23, p22, p21); // rs, e, d4-d7 00061 AnalogOut DACout(p18); 00062 wave_player waver(&DACout); 00063 DigitalIn pb1(p30); 00064 DigitalIn pb2(p29); 00065 DigitalIn pb3(p28); 00066 DigitalIn pb4(p27); 00067 00068 //communication device 00069 commSerial serDevice(USBTX, USBRX, BAUDRATE); //tx, rx 00070 00071 // Global variables for push buttons 00072 char volatile power=PHIGH, angle=45, fire; // NEW 00073 00074 //main 00075 int main() { 00076 //initialize and clear 7-Segment Display (assembly subroutine) 00077 setup_sequence(); 00078 seg_driver_initialize(); 00079 seg_driver(0); 00080 00081 // Use internal pullups for pushbuttons 00082 pb1.mode(PullUp); 00083 pb2.mode(PullUp); 00084 pb3.mode(PullUp); 00085 pb4.mode(PullUp); 00086 00087 //variables 00088 char World[10000]; 00089 00090 //check for wav file 00091 lcd.cls(); 00092 lcd.printf("Locating WAV file..."); 00093 FILE *test_file; 00094 while(1) { 00095 test_file=fopen(BOMB_WAVFILE,"r"); 00096 if(test_file != NULL) {break;} 00097 wait(0.5); 00098 } 00099 fclose(test_file); 00100 while(1) { 00101 test_file=fopen(MONKEY_WAVFILE,"r"); 00102 if(test_file != NULL) {break;} 00103 wait(0.5); 00104 } 00105 fclose(test_file); 00106 00107 //notification 00108 lcd.cls(); 00109 lcd.printf("Angry Monkeys"); 00110 wait(1); 00111 00112 00113 /******** HW 3 *********/ 00114 00115 /** HW3.A During the check off, You should show the seg_driver is working correctly **/ 00116 int i; 00117 for(i=0;i<10;i++){ 00118 seg_driver(i); 00119 wait(0.3); 00120 } 00121 00122 /** HW3.B During the check off, You should show the pushbuttons are debounced **/ 00123 00124 //temp variables 00125 int num_cannon=30, val1, val2, val3, val4; 00126 00127 fire=0; //pb4 is set to fire 00128 lcd.cls(); 00129 while(fire<9){ 00130 00131 /**** BEGIN - your code goes here for HW3.B ****/ 00132 00133 //read inputs 00134 val1 = invert(pb1.read()); 00135 val2 = invert(pb2.read()); 00136 val3 = invert(pb3.read()); 00137 val4 = invert(pb4.read()); 00138 00139 if(val1){ 00140 pb1_hit_callback(); //power 00141 } 00142 if(val2){ 00143 pb2_hit_callback(); //angle 00144 } 00145 if(val3){ 00146 pb3_hit_callback(); //angle 00147 } 00148 if(val4){ 00149 pb4_hit_callback(); //fire 00150 } 00151 00152 if (val1||val2||val3||val4){ 00153 wait(.2); 00154 } 00155 /**** END - your code stops here ****/ 00156 00157 //print lcd 00158 lcd.cls(); 00159 lcd.printf("Left cannon:%d\n", num_cannon); 00160 if(power==PHIGH) // NEW 00161 lcd.printf("Angle:%d PHIGH", angle); // NEW 00162 else // NEW 00163 lcd.printf("Angle:%d PLOW", angle); // NEW 00164 00165 wait(0.02); // NEW 00166 00167 } 00168 00169 /******** HW 3 END*********/ 00170 /** Note that you can use the HW3 for the Project 2 by moving it to the appropriate place in the Project 2. 00171 If you do not want to use HW3 for the Project 2, then you can simply remove it after check off. **/ 00172 00173 00174 /******** Project 2 *********/ 00175 //loop 00176 while(1) { 00177 00178 //synchronize front end display 00179 startGame(); 00180 00181 //receive World 00182 serDevice.receiveData(World); 00183 wait(1); 00184 00185 //get world that will be used for your work 00186 int *world; 00187 getworld(&world, World); 00188 00189 /**** BEGIN - your code goes here for project 2 ****/ 00190 00191 //debug statements 00192 print("testing"); 00193 print("string1: %s\nstring2: %s", "hello", "world"); 00194 print("int: %d, int: %d", world[2], world[3]); 00195 00196 //Just for test... 00197 for(i=1;i<10;i++){ 00198 updateShot(i-1,i,0); 00199 wait(1); 00200 } 00201 00202 playBombSound(); 00203 playMonkeySound(); 00204 00205 for(i=0;i<5;i++){ 00206 deleteTile(world[4*i+2],world[4*i+3]); 00207 wait(1); 00208 } 00209 //Just for test ends... 00210 00211 00212 //have fun... 00213 00214 /**** END - your code stops here ****/ 00215 free(world); 00216 } 00217 //end loop 00218 } 00219 00220 //fcn to send update 00221 void updateShot(int row, int column, int del){ 00222 //temp variables 00223 char buffer[BUFFSIZE]; 00224 00225 //construct message 00226 sprintf(buffer, "%s-%d-%d-%d;", "update", row, column, del); 00227 00228 //send message 00229 serDevice.sendData(buffer); 00230 } 00231 00232 //fcn to send color 00233 void colorTile(int row, int column, int strength){ 00234 //temp variables 00235 char buffer[BUFFSIZE]; 00236 00237 //construct message 00238 sprintf(buffer, "%s-%d-%d-%d;", "color", row, column, strength); 00239 00240 //send message 00241 serDevice.sendData(buffer); 00242 } 00243 00244 //fcn to send delete 00245 void deleteTile(int row, int column){ 00246 //temp variables 00247 char buffer[BUFFSIZE]; 00248 00249 //construct message 00250 sprintf(buffer, "%s-%d-%d;", "delete", row, column); 00251 00252 //send message 00253 serDevice.sendData(buffer); 00254 } 00255 00256 //fcn to send power and angle 00257 void paaUpdate(int power, int angle){ 00258 //temp variables 00259 char buffer[BUFFSIZE]; 00260 00261 //construct message 00262 sprintf(buffer, "%s-%d-%d;", "paa", power, angle); 00263 00264 //send message 00265 serDevice.sendData(buffer); 00266 } 00267 00268 //fcn to send hint 00269 void hint(int row, int column, int power, int angle){ 00270 //temp variables 00271 char buffer[BUFFSIZE]; 00272 00273 //construct message 00274 sprintf(buffer, "%s-%d-%d-%d-%d;", "hint", row, column, power, angle); 00275 00276 //send message 00277 serDevice.sendData(buffer); 00278 } 00279 00280 //fcn to get acknowledgement from serial peripheral 00281 void waitForAck(void) { 00282 //get acknowlegement 00283 char buffer[BUFFSIZE]; 00284 while(1) { 00285 serDevice.receiveData(buffer); 00286 lowerCase(buffer); 00287 if(strcmp(ACK, buffer) == 0) { 00288 break; 00289 } 00290 memset(&buffer[0],0,strlen(buffer)); 00291 } 00292 return; 00293 } 00294 00295 //fcn to initialize the frontend display 00296 void startGame(void) { 00297 //temp variables 00298 char buffer[BUFFSIZE]; 00299 00300 //construct message 00301 sprintf(buffer, "start"); 00302 00303 //send message 00304 serDevice.sendData(buffer); 00305 00306 //wait for acknowledgement 00307 waitForAck(); 00308 } 00309 00310 //fcn to play bomb noise 00311 void playBombSound(void) { 00312 //open wav file 00313 FILE *wave_file; 00314 wave_file=fopen(BOMB_WAVFILE,"r"); 00315 00316 //play wav file 00317 waver.play(wave_file); 00318 00319 //close wav file 00320 fclose(wave_file); 00321 } 00322 00323 //fcn to play bomb noise 00324 void playMonkeySound(void) { 00325 //open wav file 00326 FILE *wave_file; 00327 wave_file=fopen(MONKEY_WAVFILE,"r"); 00328 00329 //play wav file 00330 waver.play(wave_file); 00331 00332 //close wav file 00333 fclose(wave_file); 00334 } 00335 00336 //fcn to print to console 00337 void print(const char *format, ...) { 00338 //temp variables 00339 char buffer[BUFFSIZE]; 00340 char temp[BUFFSIZE-6]; 00341 00342 //construct message part 1 00343 sprintf(buffer, "print-"); 00344 00345 //construct message part 2 00346 va_list arguments; 00347 va_start(arguments, format); 00348 vsnprintf(temp, BUFFSIZE-7, format, arguments); 00349 va_end(arguments); 00350 00351 //concatenate parts 00352 strcat(buffer, temp); 00353 00354 //send message 00355 serDevice.sendData(buffer); 00356 00357 //wait for acknowledgement 00358 waitForAck(); 00359 } 00360 00361 //fcn to convert string to lowercase 00362 void lowerCase(char *src) { 00363 int i=0;; 00364 while(src[i] != '\0') { 00365 if((src[i] > 64) && (src[i] < 91)) { 00366 src[i]+=32; 00367 } 00368 i++; 00369 } 00370 return; 00371 } 00372 00373 //function to perform bitwise inversion 00374 int invert(int value) { 00375 if (value == 0) { 00376 return 1; 00377 } else { 00378 return 0; 00379 } 00380 } 00381 00382 // Callback routine is interrupt activated by a debounced pb hit 00383 void pb1_hit_callback (void) { 00384 if(power==PHIGH) // NEW 00385 power=PLOW; // NEW 00386 else // NEW 00387 power=PHIGH; // NEW 00388 } 00389 void pb2_hit_callback (void) { 00390 if(angle<90) 00391 angle++; 00392 else if(angle>=90) 00393 angle=0; 00394 } 00395 void pb3_hit_callback (void) { 00396 if(angle>0) 00397 angle--; 00398 else if(angle<=0) 00399 angle=90; 00400 } 00401 void pb4_hit_callback (void) { 00402 fire++; 00403 } 00404 00405 //func. to get world 00406 void getworld (int**world, char *World){ 00407 int i; 00408 char temp[3]; 00409 00410 //allocate world 00411 *world = (int*)malloc(sizeof(int)*(((World[2]<<8)+World[3])*4+2)); 00412 00413 //get it 00414 (*world)[0]=(World[0]<<8)+World[1]; 00415 (*world)[1]=(World[2]<<8)+World[3]; 00416 for(i=0;i<((*world)[1]*4);i++){ 00417 temp[0] = World[(2*i)+4]; 00418 temp[1] = World[(2*i)+5]; 00419 temp[2] = '\0'; 00420 sscanf(temp, "%d", &((*world)[i+2])); 00421 } 00422 }
Generated on Mon Aug 1 2022 12:01:30 by
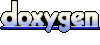