
Basic snake game
Dependencies: mbed C12832_lcd MMA7660
main.cpp
00001 // snake made for mbed application board 00002 #include "mbed.h" 00003 #include "C12832_lcd.h" 00004 00005 #define UP 1 00006 #define DOWN 2 00007 #define LEFT 4 00008 #define RIGHT 8 00009 00010 C12832_LCD lcd; 00011 00012 BusIn joystick(p15,p12,p13,p16); //up, down , left, right 00013 00014 00015 //globals 00016 int ver[50]; 00017 int hor[50]; 00018 int tail =1; 00019 int coinHor =200; //coin x,y coordinates 00020 int coinVer =200; 00021 int ranC = 12; //used for random spwan point 00022 int ranL = 52; //used for random spawn point 00023 int direction= RIGHT; 00024 00025 //function prototypes 00026 void display(int a); 00027 void control(); 00028 void coin(); 00029 00030 int main() 00031 { 00032 hor[0]= 0; 00033 ver[0] = 1; 00034 for (int p = 49; p > 0 ; p--){ 00035 hor[p]= 200; 00036 ver[p] = 200; 00037 }//end of for 00038 coin(); 00039 while(1){ 00040 lcd.cls(); 00041 control(); 00042 for (int n=0; n<tail; n++){ 00043 display(n); 00044 } 00045 wait(0.1); 00046 } 00047 }//end of main 00048 00049 00050 void display(int a) 00051 { 00052 //display box 00053 lcd.fillrect(hor[a],ver[a],hor[a]+2,ver[a]+2,1); 00054 //display coin 00055 lcd.fillrect(coinHor,coinVer,coinHor+2,coinVer+2,1); 00056 //check if maximum tail length reached 00057 if (tail>49){ lcd.locate(40,16); 00058 lcd.printf("YOU WIN!"); 00059 while(1){}} 00060 00061 00062 }//end void display 00063 00064 00065 void control(){ 00066 //shift boxes position in array 00067 for (int p = 49; p > 0 ; p--){ 00068 hor[p]= hor [p-1]; 00069 ver[p] = ver [p-1]; 00070 }//end of for 00071 00072 //moves the main box depending on direction 00073 switch (direction){ 00074 case RIGHT: if (hor[0]<123){hor[0]+=5;}else{hor[0]=0;}ranC +=2; ranL +=3; break; 00075 case LEFT: if (hor[0]>0){hor[0]-=5;}else{hor[0]=123;}ranC +=3; ranL +=5; break; 00076 case UP: if (ver[0]>0){ver[0]-=5;}else{ver[0]=25;}ranC +=8; ranL +=2; break; 00077 case DOWN: if (ver[0]<25){ver[0]+=5;}else{ver[0]=0;}ranC +=5; ranL +=9; break; 00078 }//end of switch 00079 //change direction if statements 00080 if (joystick == UP) 00081 {if (direction !=DOWN){ 00082 direction = UP; 00083 } 00084 00085 }//end of if UP 00086 00087 if (joystick == DOWN) 00088 { 00089 if (direction !=UP){ 00090 direction = DOWN;} 00091 00092 }//end of if DOWN 00093 00094 if (joystick == LEFT) 00095 { 00096 if (direction !=RIGHT){ 00097 direction = LEFT;} 00098 00099 }//end of if LEFT 00100 00101 if (joystick == RIGHT) 00102 { 00103 if (direction !=LEFT){ 00104 direction = RIGHT;} 00105 00106 }//end of if RIGHT 00107 00108 //checks if first box hits any other box 00109 for (int p = tail; p > 3 ; p--){ 00110 if( hor[0]==hor[p] && ver[0] == ver[p]){ 00111 lcd.locate(40,10); 00112 lcd.printf("YOU LOSE!"); 00113 lcd.locate(40,20); 00114 lcd.printf("Score: %d",tail); 00115 while(1){} 00116 }//end of if 00117 }//end of for 00118 00119 00120 //check if first box hits coin, adds tail and moves coin 00121 if (coinHor+5>hor[0] && hor[0]>coinHor-5 && coinVer+5>ver[0] && ver[0] >coinVer-5){ 00122 tail++; 00123 coin(); 00124 } 00125 }//end of button pressed 00126 00127 00128 void coin() { 00129 //changes location of coin 00130 coinVer = ranC % 25; 00131 coinHor = ranL % 123; 00132 00133 }//end void coin
Generated on Tue Jul 19 2022 21:22:34 by
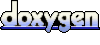